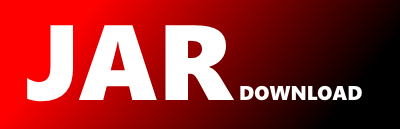
com.pulumi.consul.ProviderArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul;
import com.pulumi.consul.inputs.ProviderAuthJwtArgs;
import com.pulumi.consul.inputs.ProviderHeaderArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProviderArgs extends com.pulumi.resources.ResourceArgs {
public static final ProviderArgs Empty = new ProviderArgs();
/**
* The HTTP(S) API address of the agent to use. Defaults to "127.0.0.1:8500".
*
*/
@Import(name="address")
private @Nullable Output address;
/**
* @return The HTTP(S) API address of the agent to use. Defaults to "127.0.0.1:8500".
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy