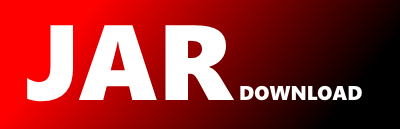
com.pulumi.consul.ServiceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul;
import com.pulumi.consul.inputs.ServiceCheckArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServiceArgs extends com.pulumi.resources.ResourceArgs {
public static final ServiceArgs Empty = new ServiceArgs();
/**
* The address of the service. Defaults to the address of the node.
*
*/
@Import(name="address")
private @Nullable Output address;
/**
* @return The address of the service. Defaults to the address of the node.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy