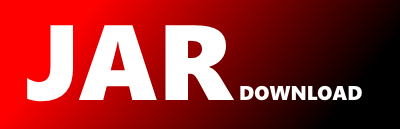
com.pulumi.consul.inputs.GetKeyPrefixPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul.inputs;
import com.pulumi.consul.inputs.GetKeyPrefixSubkeyCollection;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetKeyPrefixPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetKeyPrefixPlainArgs Empty = new GetKeyPrefixPlainArgs();
/**
* The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
*/
@Import(name="datacenter")
private @Nullable String datacenter;
/**
* @return The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
*/
public Optional datacenter() {
return Optional.ofNullable(this.datacenter);
}
/**
* The namespace to lookup the keys within.
*
*/
@Import(name="namespace")
private @Nullable String namespace;
/**
* @return The namespace to lookup the keys within.
*
*/
public Optional namespace() {
return Optional.ofNullable(this.namespace);
}
/**
* The namespace to lookup the keys within.
*
*/
@Import(name="partition")
private @Nullable String partition;
/**
* @return The namespace to lookup the keys within.
*
*/
public Optional partition() {
return Optional.ofNullable(this.partition);
}
/**
* Specifies the common prefix shared by all keys
* that will be read by this data source instance. In most cases, this will
* end with a slash to read a "folder" of subkeys.
*
*/
@Import(name="pathPrefix", required=true)
private String pathPrefix;
/**
* @return Specifies the common prefix shared by all keys
* that will be read by this data source instance. In most cases, this will
* end with a slash to read a "folder" of subkeys.
*
*/
public String pathPrefix() {
return this.pathPrefix;
}
/**
* Specifies a subkey in Consul to be read. Supported
* values documented below. Multiple blocks supported.
*
*/
@Import(name="subkeyCollection")
private @Nullable List subkeyCollection;
/**
* @return Specifies a subkey in Consul to be read. Supported
* values documented below. Multiple blocks supported.
*
*/
public Optional> subkeyCollection() {
return Optional.ofNullable(this.subkeyCollection);
}
/**
* The ACL token to use. This overrides the
* token that the agent provides by default.
*
* @deprecated
* The token argument has been deprecated and will be removed in a future release.
* Please use the token argument in the provider configuration
*
*/
@Deprecated /* The token argument has been deprecated and will be removed in a future release.
Please use the token argument in the provider configuration */
@Import(name="token")
private @Nullable String token;
/**
* @return The ACL token to use. This overrides the
* token that the agent provides by default.
*
* @deprecated
* The token argument has been deprecated and will be removed in a future release.
* Please use the token argument in the provider configuration
*
*/
@Deprecated /* The token argument has been deprecated and will be removed in a future release.
Please use the token argument in the provider configuration */
public Optional token() {
return Optional.ofNullable(this.token);
}
private GetKeyPrefixPlainArgs() {}
private GetKeyPrefixPlainArgs(GetKeyPrefixPlainArgs $) {
this.datacenter = $.datacenter;
this.namespace = $.namespace;
this.partition = $.partition;
this.pathPrefix = $.pathPrefix;
this.subkeyCollection = $.subkeyCollection;
this.token = $.token;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetKeyPrefixPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetKeyPrefixPlainArgs $;
public Builder() {
$ = new GetKeyPrefixPlainArgs();
}
public Builder(GetKeyPrefixPlainArgs defaults) {
$ = new GetKeyPrefixPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param datacenter The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
* @return builder
*
*/
public Builder datacenter(@Nullable String datacenter) {
$.datacenter = datacenter;
return this;
}
/**
* @param namespace The namespace to lookup the keys within.
*
* @return builder
*
*/
public Builder namespace(@Nullable String namespace) {
$.namespace = namespace;
return this;
}
/**
* @param partition The namespace to lookup the keys within.
*
* @return builder
*
*/
public Builder partition(@Nullable String partition) {
$.partition = partition;
return this;
}
/**
* @param pathPrefix Specifies the common prefix shared by all keys
* that will be read by this data source instance. In most cases, this will
* end with a slash to read a "folder" of subkeys.
*
* @return builder
*
*/
public Builder pathPrefix(String pathPrefix) {
$.pathPrefix = pathPrefix;
return this;
}
/**
* @param subkeyCollection Specifies a subkey in Consul to be read. Supported
* values documented below. Multiple blocks supported.
*
* @return builder
*
*/
public Builder subkeyCollection(@Nullable List subkeyCollection) {
$.subkeyCollection = subkeyCollection;
return this;
}
/**
* @param subkeyCollection Specifies a subkey in Consul to be read. Supported
* values documented below. Multiple blocks supported.
*
* @return builder
*
*/
public Builder subkeyCollection(GetKeyPrefixSubkeyCollection... subkeyCollection) {
return subkeyCollection(List.of(subkeyCollection));
}
/**
* @param token The ACL token to use. This overrides the
* token that the agent provides by default.
*
* @return builder
*
* @deprecated
* The token argument has been deprecated and will be removed in a future release.
* Please use the token argument in the provider configuration
*
*/
@Deprecated /* The token argument has been deprecated and will be removed in a future release.
Please use the token argument in the provider configuration */
public Builder token(@Nullable String token) {
$.token = token;
return this;
}
public GetKeyPrefixPlainArgs build() {
if ($.pathPrefix == null) {
throw new MissingRequiredPropertyException("GetKeyPrefixPlainArgs", "pathPrefix");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy