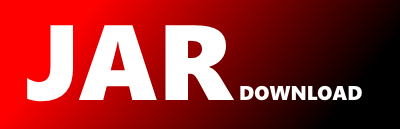
com.pulumi.consul.outputs.GetServiceService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetServiceService {
private String address;
private String createIndex;
private String enableTagOverride;
private String id;
private Map meta;
private String modifyIndex;
/**
* @return The service name to select.
*
*/
private String name;
private String nodeAddress;
/**
* @return The Node ID of the Consul agent advertising the service.
* * [`node_meta`](https://www.consul.io/docs/agent/http/catalog.html#Meta) - Node
* meta data tag information, if any.
* * [`node_name`](https://www.consul.io/docs/agent/http/catalog.html#Node) - The
* name of the Consul node.
* * [`address`](https://www.consul.io/docs/agent/http/catalog.html#ServiceAddress) -
* The IP address of the service. If the `ServiceAddress` in the Consul catalog
* is empty, this value is automatically populated with the `node_address` (the
* `Address` in the Consul Catalog).
* * [`enable_tag_override`](https://www.consul.io/docs/agent/http/catalog.html#ServiceEnableTagOverride) -
* Whether service tags can be overridden on this service.
* * [`id`](https://www.consul.io/docs/agent/http/catalog.html#ServiceID) - A
* unique service instance identifier.
* * [`name`](https://www.consul.io/docs/agent/http/catalog.html#ServiceName) - The
* name of the service.
* * [`port`](https://www.consul.io/docs/agent/http/catalog.html#ServicePort) -
* Port number of the service.
* * [`tagged_addresses`](https://www.consul.io/docs/agent/http/catalog.html#TaggedAddresses) -
* List of explicit LAN and WAN IP addresses for the agent.
* * [`tags`](https://www.consul.io/docs/agent/http/catalog.html#ServiceTags) -
* List of tags for the service.
* * [`meta`](https://www.consul.io/docs/agent/http/catalog.html#Meta) - Service meta
* data tag information, if any.
*
*/
private String nodeId;
private Map nodeMeta;
private String nodeName;
private String port;
private Map taggedAddresses;
private List tags;
private GetServiceService() {}
public String address() {
return this.address;
}
public String createIndex() {
return this.createIndex;
}
public String enableTagOverride() {
return this.enableTagOverride;
}
public String id() {
return this.id;
}
public Map meta() {
return this.meta;
}
public String modifyIndex() {
return this.modifyIndex;
}
/**
* @return The service name to select.
*
*/
public String name() {
return this.name;
}
public String nodeAddress() {
return this.nodeAddress;
}
/**
* @return The Node ID of the Consul agent advertising the service.
* * [`node_meta`](https://www.consul.io/docs/agent/http/catalog.html#Meta) - Node
* meta data tag information, if any.
* * [`node_name`](https://www.consul.io/docs/agent/http/catalog.html#Node) - The
* name of the Consul node.
* * [`address`](https://www.consul.io/docs/agent/http/catalog.html#ServiceAddress) -
* The IP address of the service. If the `ServiceAddress` in the Consul catalog
* is empty, this value is automatically populated with the `node_address` (the
* `Address` in the Consul Catalog).
* * [`enable_tag_override`](https://www.consul.io/docs/agent/http/catalog.html#ServiceEnableTagOverride) -
* Whether service tags can be overridden on this service.
* * [`id`](https://www.consul.io/docs/agent/http/catalog.html#ServiceID) - A
* unique service instance identifier.
* * [`name`](https://www.consul.io/docs/agent/http/catalog.html#ServiceName) - The
* name of the service.
* * [`port`](https://www.consul.io/docs/agent/http/catalog.html#ServicePort) -
* Port number of the service.
* * [`tagged_addresses`](https://www.consul.io/docs/agent/http/catalog.html#TaggedAddresses) -
* List of explicit LAN and WAN IP addresses for the agent.
* * [`tags`](https://www.consul.io/docs/agent/http/catalog.html#ServiceTags) -
* List of tags for the service.
* * [`meta`](https://www.consul.io/docs/agent/http/catalog.html#Meta) - Service meta
* data tag information, if any.
*
*/
public String nodeId() {
return this.nodeId;
}
public Map nodeMeta() {
return this.nodeMeta;
}
public String nodeName() {
return this.nodeName;
}
public String port() {
return this.port;
}
public Map taggedAddresses() {
return this.taggedAddresses;
}
public List tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServiceService defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String address;
private String createIndex;
private String enableTagOverride;
private String id;
private Map meta;
private String modifyIndex;
private String name;
private String nodeAddress;
private String nodeId;
private Map nodeMeta;
private String nodeName;
private String port;
private Map taggedAddresses;
private List tags;
public Builder() {}
public Builder(GetServiceService defaults) {
Objects.requireNonNull(defaults);
this.address = defaults.address;
this.createIndex = defaults.createIndex;
this.enableTagOverride = defaults.enableTagOverride;
this.id = defaults.id;
this.meta = defaults.meta;
this.modifyIndex = defaults.modifyIndex;
this.name = defaults.name;
this.nodeAddress = defaults.nodeAddress;
this.nodeId = defaults.nodeId;
this.nodeMeta = defaults.nodeMeta;
this.nodeName = defaults.nodeName;
this.port = defaults.port;
this.taggedAddresses = defaults.taggedAddresses;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder address(String address) {
if (address == null) {
throw new MissingRequiredPropertyException("GetServiceService", "address");
}
this.address = address;
return this;
}
@CustomType.Setter
public Builder createIndex(String createIndex) {
if (createIndex == null) {
throw new MissingRequiredPropertyException("GetServiceService", "createIndex");
}
this.createIndex = createIndex;
return this;
}
@CustomType.Setter
public Builder enableTagOverride(String enableTagOverride) {
if (enableTagOverride == null) {
throw new MissingRequiredPropertyException("GetServiceService", "enableTagOverride");
}
this.enableTagOverride = enableTagOverride;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServiceService", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder meta(Map meta) {
if (meta == null) {
throw new MissingRequiredPropertyException("GetServiceService", "meta");
}
this.meta = meta;
return this;
}
@CustomType.Setter
public Builder modifyIndex(String modifyIndex) {
if (modifyIndex == null) {
throw new MissingRequiredPropertyException("GetServiceService", "modifyIndex");
}
this.modifyIndex = modifyIndex;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServiceService", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nodeAddress(String nodeAddress) {
if (nodeAddress == null) {
throw new MissingRequiredPropertyException("GetServiceService", "nodeAddress");
}
this.nodeAddress = nodeAddress;
return this;
}
@CustomType.Setter
public Builder nodeId(String nodeId) {
if (nodeId == null) {
throw new MissingRequiredPropertyException("GetServiceService", "nodeId");
}
this.nodeId = nodeId;
return this;
}
@CustomType.Setter
public Builder nodeMeta(Map nodeMeta) {
if (nodeMeta == null) {
throw new MissingRequiredPropertyException("GetServiceService", "nodeMeta");
}
this.nodeMeta = nodeMeta;
return this;
}
@CustomType.Setter
public Builder nodeName(String nodeName) {
if (nodeName == null) {
throw new MissingRequiredPropertyException("GetServiceService", "nodeName");
}
this.nodeName = nodeName;
return this;
}
@CustomType.Setter
public Builder port(String port) {
if (port == null) {
throw new MissingRequiredPropertyException("GetServiceService", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder taggedAddresses(Map taggedAddresses) {
if (taggedAddresses == null) {
throw new MissingRequiredPropertyException("GetServiceService", "taggedAddresses");
}
this.taggedAddresses = taggedAddresses;
return this;
}
@CustomType.Setter
public Builder tags(List tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetServiceService", "tags");
}
this.tags = tags;
return this;
}
public Builder tags(String... tags) {
return tags(List.of(tags));
}
public GetServiceService build() {
final var _resultValue = new GetServiceService();
_resultValue.address = address;
_resultValue.createIndex = createIndex;
_resultValue.enableTagOverride = enableTagOverride;
_resultValue.id = id;
_resultValue.meta = meta;
_resultValue.modifyIndex = modifyIndex;
_resultValue.name = name;
_resultValue.nodeAddress = nodeAddress;
_resultValue.nodeId = nodeId;
_resultValue.nodeMeta = nodeMeta;
_resultValue.nodeName = nodeName;
_resultValue.port = port;
_resultValue.taggedAddresses = taggedAddresses;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy