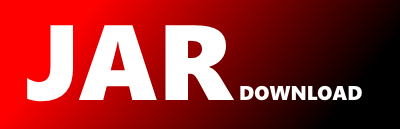
com.pulumi.consul.outputs.ConfigEntryServiceDefaultsUpstreamConfigOverride Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul.outputs;
import com.pulumi.consul.outputs.ConfigEntryServiceDefaultsUpstreamConfigOverrideLimit;
import com.pulumi.consul.outputs.ConfigEntryServiceDefaultsUpstreamConfigOverrideMeshGateway;
import com.pulumi.consul.outputs.ConfigEntryServiceDefaultsUpstreamConfigOverridePassiveHealthCheck;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ConfigEntryServiceDefaultsUpstreamConfigOverride {
/**
* @return Sets the strategy for allocating outbound connections from upstreams across Envoy proxy threads.
*
*/
private @Nullable String balanceOutboundConnections;
private @Nullable Integer connectTimeoutMs;
private @Nullable String envoyListenerJson;
/**
* @return Map that specifies a set of limits to apply to when connecting upstream services.
*
*/
private @Nullable List limits;
/**
* @return Specifies the default mesh gateway mode field for all upstreams.
*
*/
private @Nullable List meshGateways;
/**
* @return Specifies the name of the service you are setting the defaults for.
*
*/
private @Nullable String name;
/**
* @return Specifies the namespace containing the upstream service that the configuration applies to.
*
*/
private @Nullable String namespace;
/**
* @return Specifies the name of the name of the Consul admin partition that the configuration entry applies to.
*
*/
private @Nullable String partition;
/**
* @return Map that specifies a set of rules that enable Consul to remove hosts from the upstream cluster that are unreachable or that return errors.
*
*/
private @Nullable List passiveHealthChecks;
/**
* @return Specifies the peer name of the upstream service that the configuration applies to.
*
*/
private @Nullable String peer;
/**
* @return Specifies the default protocol for the service.
*
*/
private @Nullable String protocol;
private ConfigEntryServiceDefaultsUpstreamConfigOverride() {}
/**
* @return Sets the strategy for allocating outbound connections from upstreams across Envoy proxy threads.
*
*/
public Optional balanceOutboundConnections() {
return Optional.ofNullable(this.balanceOutboundConnections);
}
public Optional connectTimeoutMs() {
return Optional.ofNullable(this.connectTimeoutMs);
}
public Optional envoyListenerJson() {
return Optional.ofNullable(this.envoyListenerJson);
}
/**
* @return Map that specifies a set of limits to apply to when connecting upstream services.
*
*/
public List limits() {
return this.limits == null ? List.of() : this.limits;
}
/**
* @return Specifies the default mesh gateway mode field for all upstreams.
*
*/
public List meshGateways() {
return this.meshGateways == null ? List.of() : this.meshGateways;
}
/**
* @return Specifies the name of the service you are setting the defaults for.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Specifies the namespace containing the upstream service that the configuration applies to.
*
*/
public Optional namespace() {
return Optional.ofNullable(this.namespace);
}
/**
* @return Specifies the name of the name of the Consul admin partition that the configuration entry applies to.
*
*/
public Optional partition() {
return Optional.ofNullable(this.partition);
}
/**
* @return Map that specifies a set of rules that enable Consul to remove hosts from the upstream cluster that are unreachable or that return errors.
*
*/
public List passiveHealthChecks() {
return this.passiveHealthChecks == null ? List.of() : this.passiveHealthChecks;
}
/**
* @return Specifies the peer name of the upstream service that the configuration applies to.
*
*/
public Optional peer() {
return Optional.ofNullable(this.peer);
}
/**
* @return Specifies the default protocol for the service.
*
*/
public Optional protocol() {
return Optional.ofNullable(this.protocol);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ConfigEntryServiceDefaultsUpstreamConfigOverride defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String balanceOutboundConnections;
private @Nullable Integer connectTimeoutMs;
private @Nullable String envoyListenerJson;
private @Nullable List limits;
private @Nullable List meshGateways;
private @Nullable String name;
private @Nullable String namespace;
private @Nullable String partition;
private @Nullable List passiveHealthChecks;
private @Nullable String peer;
private @Nullable String protocol;
public Builder() {}
public Builder(ConfigEntryServiceDefaultsUpstreamConfigOverride defaults) {
Objects.requireNonNull(defaults);
this.balanceOutboundConnections = defaults.balanceOutboundConnections;
this.connectTimeoutMs = defaults.connectTimeoutMs;
this.envoyListenerJson = defaults.envoyListenerJson;
this.limits = defaults.limits;
this.meshGateways = defaults.meshGateways;
this.name = defaults.name;
this.namespace = defaults.namespace;
this.partition = defaults.partition;
this.passiveHealthChecks = defaults.passiveHealthChecks;
this.peer = defaults.peer;
this.protocol = defaults.protocol;
}
@CustomType.Setter
public Builder balanceOutboundConnections(@Nullable String balanceOutboundConnections) {
this.balanceOutboundConnections = balanceOutboundConnections;
return this;
}
@CustomType.Setter
public Builder connectTimeoutMs(@Nullable Integer connectTimeoutMs) {
this.connectTimeoutMs = connectTimeoutMs;
return this;
}
@CustomType.Setter
public Builder envoyListenerJson(@Nullable String envoyListenerJson) {
this.envoyListenerJson = envoyListenerJson;
return this;
}
@CustomType.Setter
public Builder limits(@Nullable List limits) {
this.limits = limits;
return this;
}
public Builder limits(ConfigEntryServiceDefaultsUpstreamConfigOverrideLimit... limits) {
return limits(List.of(limits));
}
@CustomType.Setter
public Builder meshGateways(@Nullable List meshGateways) {
this.meshGateways = meshGateways;
return this;
}
public Builder meshGateways(ConfigEntryServiceDefaultsUpstreamConfigOverrideMeshGateway... meshGateways) {
return meshGateways(List.of(meshGateways));
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder partition(@Nullable String partition) {
this.partition = partition;
return this;
}
@CustomType.Setter
public Builder passiveHealthChecks(@Nullable List passiveHealthChecks) {
this.passiveHealthChecks = passiveHealthChecks;
return this;
}
public Builder passiveHealthChecks(ConfigEntryServiceDefaultsUpstreamConfigOverridePassiveHealthCheck... passiveHealthChecks) {
return passiveHealthChecks(List.of(passiveHealthChecks));
}
@CustomType.Setter
public Builder peer(@Nullable String peer) {
this.peer = peer;
return this;
}
@CustomType.Setter
public Builder protocol(@Nullable String protocol) {
this.protocol = protocol;
return this;
}
public ConfigEntryServiceDefaultsUpstreamConfigOverride build() {
final var _resultValue = new ConfigEntryServiceDefaultsUpstreamConfigOverride();
_resultValue.balanceOutboundConnections = balanceOutboundConnections;
_resultValue.connectTimeoutMs = connectTimeoutMs;
_resultValue.envoyListenerJson = envoyListenerJson;
_resultValue.limits = limits;
_resultValue.meshGateways = meshGateways;
_resultValue.name = name;
_resultValue.namespace = namespace;
_resultValue.partition = partition;
_resultValue.passiveHealthChecks = passiveHealthChecks;
_resultValue.peer = peer;
_resultValue.protocol = protocol;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy