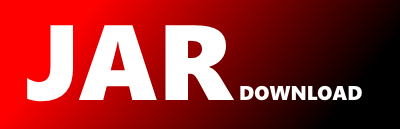
com.pulumi.consul.outputs.ConfigEntryServiceRouterRouteDestination Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul.outputs;
import com.pulumi.consul.outputs.ConfigEntryServiceRouterRouteDestinationRequestHeaders;
import com.pulumi.consul.outputs.ConfigEntryServiceRouterRouteDestinationResponseHeaders;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ConfigEntryServiceRouterRouteDestination {
/**
* @return Specifies the total amount of time permitted for the request stream to be idle.
*
*/
private @Nullable String idleTimeout;
/**
* @return Specifies the Consul namespace to resolve the service from instead of the current namespace.
*
*/
private @Nullable String namespace;
/**
* @return Specifies the number of times to retry the request when a retry condition occurs.
*
*/
private @Nullable Integer numRetries;
/**
* @return Specifies the Consul admin partition to resolve the service from instead of the current partition.
*
*/
private @Nullable String partition;
/**
* @return Specifies rewrites to the HTTP request path before proxying it to its final destination.
*
*/
private @Nullable String prefixRewrite;
/**
* @return Specifies a set of HTTP-specific header modification rules applied to requests routed with the service router.
*
*/
private @Nullable ConfigEntryServiceRouterRouteDestinationRequestHeaders requestHeaders;
/**
* @return Specifies the total amount of time permitted for the entire downstream request to be processed, including retry attempts.
*
*/
private @Nullable String requestTimeout;
/**
* @return Specifies a set of HTTP-specific header modification rules applied to responses routed with the service router.
*
*/
private @Nullable ConfigEntryServiceRouterRouteDestinationResponseHeaders responseHeaders;
/**
* @return Specifies that connection failure errors that trigger a retry request.
*
*/
private @Nullable Boolean retryOnConnectFailure;
/**
* @return Specifies a list of integers for HTTP response status codes that trigger a retry request.
*
*/
private @Nullable List retryOnStatusCodes;
/**
* @return Specifies a list of conditions for Consul to retry requests based on the response from an upstream service.
*
*/
private @Nullable List retryOns;
/**
* @return Specifies the name of the service to resolve.
*
*/
private @Nullable String service;
/**
* @return Specifies a named subset of the given service to resolve instead of the one defined as that service's `default_subset` in the service resolver configuration entry.
*
*/
private @Nullable String serviceSubset;
private ConfigEntryServiceRouterRouteDestination() {}
/**
* @return Specifies the total amount of time permitted for the request stream to be idle.
*
*/
public Optional idleTimeout() {
return Optional.ofNullable(this.idleTimeout);
}
/**
* @return Specifies the Consul namespace to resolve the service from instead of the current namespace.
*
*/
public Optional namespace() {
return Optional.ofNullable(this.namespace);
}
/**
* @return Specifies the number of times to retry the request when a retry condition occurs.
*
*/
public Optional numRetries() {
return Optional.ofNullable(this.numRetries);
}
/**
* @return Specifies the Consul admin partition to resolve the service from instead of the current partition.
*
*/
public Optional partition() {
return Optional.ofNullable(this.partition);
}
/**
* @return Specifies rewrites to the HTTP request path before proxying it to its final destination.
*
*/
public Optional prefixRewrite() {
return Optional.ofNullable(this.prefixRewrite);
}
/**
* @return Specifies a set of HTTP-specific header modification rules applied to requests routed with the service router.
*
*/
public Optional requestHeaders() {
return Optional.ofNullable(this.requestHeaders);
}
/**
* @return Specifies the total amount of time permitted for the entire downstream request to be processed, including retry attempts.
*
*/
public Optional requestTimeout() {
return Optional.ofNullable(this.requestTimeout);
}
/**
* @return Specifies a set of HTTP-specific header modification rules applied to responses routed with the service router.
*
*/
public Optional responseHeaders() {
return Optional.ofNullable(this.responseHeaders);
}
/**
* @return Specifies that connection failure errors that trigger a retry request.
*
*/
public Optional retryOnConnectFailure() {
return Optional.ofNullable(this.retryOnConnectFailure);
}
/**
* @return Specifies a list of integers for HTTP response status codes that trigger a retry request.
*
*/
public List retryOnStatusCodes() {
return this.retryOnStatusCodes == null ? List.of() : this.retryOnStatusCodes;
}
/**
* @return Specifies a list of conditions for Consul to retry requests based on the response from an upstream service.
*
*/
public List retryOns() {
return this.retryOns == null ? List.of() : this.retryOns;
}
/**
* @return Specifies the name of the service to resolve.
*
*/
public Optional service() {
return Optional.ofNullable(this.service);
}
/**
* @return Specifies a named subset of the given service to resolve instead of the one defined as that service's `default_subset` in the service resolver configuration entry.
*
*/
public Optional serviceSubset() {
return Optional.ofNullable(this.serviceSubset);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ConfigEntryServiceRouterRouteDestination defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String idleTimeout;
private @Nullable String namespace;
private @Nullable Integer numRetries;
private @Nullable String partition;
private @Nullable String prefixRewrite;
private @Nullable ConfigEntryServiceRouterRouteDestinationRequestHeaders requestHeaders;
private @Nullable String requestTimeout;
private @Nullable ConfigEntryServiceRouterRouteDestinationResponseHeaders responseHeaders;
private @Nullable Boolean retryOnConnectFailure;
private @Nullable List retryOnStatusCodes;
private @Nullable List retryOns;
private @Nullable String service;
private @Nullable String serviceSubset;
public Builder() {}
public Builder(ConfigEntryServiceRouterRouteDestination defaults) {
Objects.requireNonNull(defaults);
this.idleTimeout = defaults.idleTimeout;
this.namespace = defaults.namespace;
this.numRetries = defaults.numRetries;
this.partition = defaults.partition;
this.prefixRewrite = defaults.prefixRewrite;
this.requestHeaders = defaults.requestHeaders;
this.requestTimeout = defaults.requestTimeout;
this.responseHeaders = defaults.responseHeaders;
this.retryOnConnectFailure = defaults.retryOnConnectFailure;
this.retryOnStatusCodes = defaults.retryOnStatusCodes;
this.retryOns = defaults.retryOns;
this.service = defaults.service;
this.serviceSubset = defaults.serviceSubset;
}
@CustomType.Setter
public Builder idleTimeout(@Nullable String idleTimeout) {
this.idleTimeout = idleTimeout;
return this;
}
@CustomType.Setter
public Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder numRetries(@Nullable Integer numRetries) {
this.numRetries = numRetries;
return this;
}
@CustomType.Setter
public Builder partition(@Nullable String partition) {
this.partition = partition;
return this;
}
@CustomType.Setter
public Builder prefixRewrite(@Nullable String prefixRewrite) {
this.prefixRewrite = prefixRewrite;
return this;
}
@CustomType.Setter
public Builder requestHeaders(@Nullable ConfigEntryServiceRouterRouteDestinationRequestHeaders requestHeaders) {
this.requestHeaders = requestHeaders;
return this;
}
@CustomType.Setter
public Builder requestTimeout(@Nullable String requestTimeout) {
this.requestTimeout = requestTimeout;
return this;
}
@CustomType.Setter
public Builder responseHeaders(@Nullable ConfigEntryServiceRouterRouteDestinationResponseHeaders responseHeaders) {
this.responseHeaders = responseHeaders;
return this;
}
@CustomType.Setter
public Builder retryOnConnectFailure(@Nullable Boolean retryOnConnectFailure) {
this.retryOnConnectFailure = retryOnConnectFailure;
return this;
}
@CustomType.Setter
public Builder retryOnStatusCodes(@Nullable List retryOnStatusCodes) {
this.retryOnStatusCodes = retryOnStatusCodes;
return this;
}
public Builder retryOnStatusCodes(Integer... retryOnStatusCodes) {
return retryOnStatusCodes(List.of(retryOnStatusCodes));
}
@CustomType.Setter
public Builder retryOns(@Nullable List retryOns) {
this.retryOns = retryOns;
return this;
}
public Builder retryOns(String... retryOns) {
return retryOns(List.of(retryOns));
}
@CustomType.Setter
public Builder service(@Nullable String service) {
this.service = service;
return this;
}
@CustomType.Setter
public Builder serviceSubset(@Nullable String serviceSubset) {
this.serviceSubset = serviceSubset;
return this;
}
public ConfigEntryServiceRouterRouteDestination build() {
final var _resultValue = new ConfigEntryServiceRouterRouteDestination();
_resultValue.idleTimeout = idleTimeout;
_resultValue.namespace = namespace;
_resultValue.numRetries = numRetries;
_resultValue.partition = partition;
_resultValue.prefixRewrite = prefixRewrite;
_resultValue.requestHeaders = requestHeaders;
_resultValue.requestTimeout = requestTimeout;
_resultValue.responseHeaders = responseHeaders;
_resultValue.retryOnConnectFailure = retryOnConnectFailure;
_resultValue.retryOnStatusCodes = retryOnStatusCodes;
_resultValue.retryOns = retryOns;
_resultValue.service = service;
_resultValue.serviceSubset = serviceSubset;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy