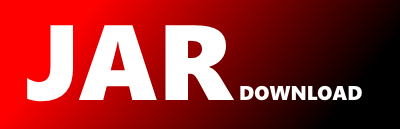
com.pulumi.consul.outputs.GetAclAuthMethodResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul.outputs;
import com.pulumi.consul.outputs.GetAclAuthMethodNamespaceRule;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAclAuthMethodResult {
/**
* @return The configuration options of the ACL Auth Method. This attribute is
* deprecated and will be removed in a future version. If the configuration is
* too complex to be represented as a map of strings, it will be blank.
* `config_json` should be used instead.
*
* @deprecated
* The config attribute is deprecated, please use config_json instead.
*
*/
@Deprecated /* The config attribute is deprecated, please use config_json instead. */
private Map config;
/**
* @return The configuration options of the ACL Auth Method.
*
*/
private String configJson;
/**
* @return The description of the ACL Auth Method.
*
*/
private String description;
/**
* @return An optional name to use instead of the name attribute when
* displaying information about this auth method.
*
*/
private String displayName;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return The maximum life of any token created by this auth method.
*
*/
private String maxTokenTtl;
private String name;
private @Nullable String namespace;
/**
* @return (Enterprise Only) A set of rules that control which
* namespace tokens created via this auth method will be created within
*
*/
private List namespaceRules;
private @Nullable String partition;
/**
* @return The kind of token that this auth method produces. This can
* be either 'local' or 'global'.
*
*/
private String tokenLocality;
/**
* @return The type of the ACL Auth Method.
*
*/
private String type;
private GetAclAuthMethodResult() {}
/**
* @return The configuration options of the ACL Auth Method. This attribute is
* deprecated and will be removed in a future version. If the configuration is
* too complex to be represented as a map of strings, it will be blank.
* `config_json` should be used instead.
*
* @deprecated
* The config attribute is deprecated, please use config_json instead.
*
*/
@Deprecated /* The config attribute is deprecated, please use config_json instead. */
public Map config() {
return this.config;
}
/**
* @return The configuration options of the ACL Auth Method.
*
*/
public String configJson() {
return this.configJson;
}
/**
* @return The description of the ACL Auth Method.
*
*/
public String description() {
return this.description;
}
/**
* @return An optional name to use instead of the name attribute when
* displaying information about this auth method.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The maximum life of any token created by this auth method.
*
*/
public String maxTokenTtl() {
return this.maxTokenTtl;
}
public String name() {
return this.name;
}
public Optional namespace() {
return Optional.ofNullable(this.namespace);
}
/**
* @return (Enterprise Only) A set of rules that control which
* namespace tokens created via this auth method will be created within
*
*/
public List namespaceRules() {
return this.namespaceRules;
}
public Optional partition() {
return Optional.ofNullable(this.partition);
}
/**
* @return The kind of token that this auth method produces. This can
* be either 'local' or 'global'.
*
*/
public String tokenLocality() {
return this.tokenLocality;
}
/**
* @return The type of the ACL Auth Method.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAclAuthMethodResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Map config;
private String configJson;
private String description;
private String displayName;
private String id;
private String maxTokenTtl;
private String name;
private @Nullable String namespace;
private List namespaceRules;
private @Nullable String partition;
private String tokenLocality;
private String type;
public Builder() {}
public Builder(GetAclAuthMethodResult defaults) {
Objects.requireNonNull(defaults);
this.config = defaults.config;
this.configJson = defaults.configJson;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.id = defaults.id;
this.maxTokenTtl = defaults.maxTokenTtl;
this.name = defaults.name;
this.namespace = defaults.namespace;
this.namespaceRules = defaults.namespaceRules;
this.partition = defaults.partition;
this.tokenLocality = defaults.tokenLocality;
this.type = defaults.type;
}
@CustomType.Setter
public Builder config(Map config) {
if (config == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "config");
}
this.config = config;
return this;
}
@CustomType.Setter
public Builder configJson(String configJson) {
if (configJson == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "configJson");
}
this.configJson = configJson;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder maxTokenTtl(String maxTokenTtl) {
if (maxTokenTtl == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "maxTokenTtl");
}
this.maxTokenTtl = maxTokenTtl;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder namespaceRules(List namespaceRules) {
if (namespaceRules == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "namespaceRules");
}
this.namespaceRules = namespaceRules;
return this;
}
public Builder namespaceRules(GetAclAuthMethodNamespaceRule... namespaceRules) {
return namespaceRules(List.of(namespaceRules));
}
@CustomType.Setter
public Builder partition(@Nullable String partition) {
this.partition = partition;
return this;
}
@CustomType.Setter
public Builder tokenLocality(String tokenLocality) {
if (tokenLocality == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "tokenLocality");
}
this.tokenLocality = tokenLocality;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAclAuthMethodResult", "type");
}
this.type = type;
return this;
}
public GetAclAuthMethodResult build() {
final var _resultValue = new GetAclAuthMethodResult();
_resultValue.config = config;
_resultValue.configJson = configJson;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.id = id;
_resultValue.maxTokenTtl = maxTokenTtl;
_resultValue.name = name;
_resultValue.namespace = namespace;
_resultValue.namespaceRules = namespaceRules;
_resultValue.partition = partition;
_resultValue.tokenLocality = tokenLocality;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy