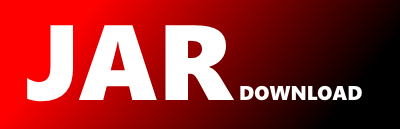
com.pulumi.dbtcloud.outputs.GlobalConnectionSnowflake Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbtcloud Show documentation
Show all versions of dbtcloud Show documentation
A Pulumi package for creating and managing dbt Cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dbtcloud.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GlobalConnectionSnowflake {
/**
* @return The Snowflake account name
*
*/
private String account;
/**
* @return Whether to allow Snowflake OAuth for the connection. If true, the `oauth_client_id` and `oauth_client_secret` fields must be set
*
*/
private @Nullable Boolean allowSso;
/**
* @return If true, the snowflake client will keep connections for longer than the default 4 hours. This is helpful when particularly long-running queries are executing (> 4 hours)
*
*/
private @Nullable Boolean clientSessionKeepAlive;
/**
* @return The default database for the connection
*
*/
private String database;
/**
* @return OAuth Client ID. Required to allow OAuth between dbt Cloud and Snowflake
*
*/
private @Nullable String oauthClientId;
/**
* @return OAuth Client Secret. Required to allow OAuth between dbt Cloud and Snowflake
*
*/
private @Nullable String oauthClientSecret;
/**
* @return The Snowflake role to use when running queries on the connection
*
*/
private @Nullable String role;
/**
* @return The default Snowflake Warehouse to use for the connection
*
*/
private String warehouse;
private GlobalConnectionSnowflake() {}
/**
* @return The Snowflake account name
*
*/
public String account() {
return this.account;
}
/**
* @return Whether to allow Snowflake OAuth for the connection. If true, the `oauth_client_id` and `oauth_client_secret` fields must be set
*
*/
public Optional allowSso() {
return Optional.ofNullable(this.allowSso);
}
/**
* @return If true, the snowflake client will keep connections for longer than the default 4 hours. This is helpful when particularly long-running queries are executing (> 4 hours)
*
*/
public Optional clientSessionKeepAlive() {
return Optional.ofNullable(this.clientSessionKeepAlive);
}
/**
* @return The default database for the connection
*
*/
public String database() {
return this.database;
}
/**
* @return OAuth Client ID. Required to allow OAuth between dbt Cloud and Snowflake
*
*/
public Optional oauthClientId() {
return Optional.ofNullable(this.oauthClientId);
}
/**
* @return OAuth Client Secret. Required to allow OAuth between dbt Cloud and Snowflake
*
*/
public Optional oauthClientSecret() {
return Optional.ofNullable(this.oauthClientSecret);
}
/**
* @return The Snowflake role to use when running queries on the connection
*
*/
public Optional role() {
return Optional.ofNullable(this.role);
}
/**
* @return The default Snowflake Warehouse to use for the connection
*
*/
public String warehouse() {
return this.warehouse;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GlobalConnectionSnowflake defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String account;
private @Nullable Boolean allowSso;
private @Nullable Boolean clientSessionKeepAlive;
private String database;
private @Nullable String oauthClientId;
private @Nullable String oauthClientSecret;
private @Nullable String role;
private String warehouse;
public Builder() {}
public Builder(GlobalConnectionSnowflake defaults) {
Objects.requireNonNull(defaults);
this.account = defaults.account;
this.allowSso = defaults.allowSso;
this.clientSessionKeepAlive = defaults.clientSessionKeepAlive;
this.database = defaults.database;
this.oauthClientId = defaults.oauthClientId;
this.oauthClientSecret = defaults.oauthClientSecret;
this.role = defaults.role;
this.warehouse = defaults.warehouse;
}
@CustomType.Setter
public Builder account(String account) {
if (account == null) {
throw new MissingRequiredPropertyException("GlobalConnectionSnowflake", "account");
}
this.account = account;
return this;
}
@CustomType.Setter
public Builder allowSso(@Nullable Boolean allowSso) {
this.allowSso = allowSso;
return this;
}
@CustomType.Setter
public Builder clientSessionKeepAlive(@Nullable Boolean clientSessionKeepAlive) {
this.clientSessionKeepAlive = clientSessionKeepAlive;
return this;
}
@CustomType.Setter
public Builder database(String database) {
if (database == null) {
throw new MissingRequiredPropertyException("GlobalConnectionSnowflake", "database");
}
this.database = database;
return this;
}
@CustomType.Setter
public Builder oauthClientId(@Nullable String oauthClientId) {
this.oauthClientId = oauthClientId;
return this;
}
@CustomType.Setter
public Builder oauthClientSecret(@Nullable String oauthClientSecret) {
this.oauthClientSecret = oauthClientSecret;
return this;
}
@CustomType.Setter
public Builder role(@Nullable String role) {
this.role = role;
return this;
}
@CustomType.Setter
public Builder warehouse(String warehouse) {
if (warehouse == null) {
throw new MissingRequiredPropertyException("GlobalConnectionSnowflake", "warehouse");
}
this.warehouse = warehouse;
return this;
}
public GlobalConnectionSnowflake build() {
final var _resultValue = new GlobalConnectionSnowflake();
_resultValue.account = account;
_resultValue.allowSso = allowSso;
_resultValue.clientSessionKeepAlive = clientSessionKeepAlive;
_resultValue.database = database;
_resultValue.oauthClientId = oauthClientId;
_resultValue.oauthClientSecret = oauthClientSecret;
_resultValue.role = role;
_resultValue.warehouse = warehouse;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy