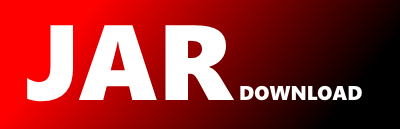
com.pulumi.dnsimple.LetsEncryptCertificateArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dnsimple Show documentation
Show all versions of dnsimple Show documentation
A Pulumi package for creating and managing dnsimple cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dnsimple;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LetsEncryptCertificateArgs extends com.pulumi.resources.ResourceArgs {
public static final LetsEncryptCertificateArgs Empty = new LetsEncryptCertificateArgs();
/**
* The certificate alternate names
*
*/
@Import(name="alternateNames")
private @Nullable Output> alternateNames;
/**
* @return The certificate alternate names
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy