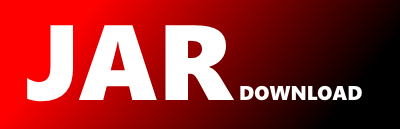
com.pulumi.dockerbuild.Image Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.dockerbuild.ImageArgs;
import com.pulumi.dockerbuild.Utilities;
import com.pulumi.dockerbuild.enums.NetworkMode;
import com.pulumi.dockerbuild.enums.Platform;
import com.pulumi.dockerbuild.outputs.BuildContext;
import com.pulumi.dockerbuild.outputs.BuilderConfig;
import com.pulumi.dockerbuild.outputs.CacheFrom;
import com.pulumi.dockerbuild.outputs.CacheTo;
import com.pulumi.dockerbuild.outputs.Dockerfile;
import com.pulumi.dockerbuild.outputs.Registry;
import com.pulumi.dockerbuild.outputs.SSH;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A Docker image built using buildx -- Docker's interface to the improved
* BuildKit backend.
*
* ## Stability
*
* **This resource is pre-1.0 and in public preview.**
*
* We will strive to keep APIs and behavior as stable as possible, but we
* cannot guarantee stability until version 1.0.
*
* ## Migrating Pulumi Docker v3 and v4 Image resources
*
* This provider's `Image` resource provides a superset of functionality over the `Image` resources available in versions 3 and 4 of the Pulumi Docker provider.
* Existing `Image` resources can be converted to the docker-build `Image` resources with minor modifications.
*
* ### Behavioral differences
*
* There are several key behavioral differences to keep in mind when transitioning images to the new `Image` resource.
*
* #### Previews
*
* Version `3.x` of the Pulumi Docker provider always builds images during preview operations.
* This is helpful as a safeguard to prevent "broken" images from merging, but users found the behavior unnecessarily redundant when running previews and updates locally.
*
* Version `4.x` changed build-on-preview behavior to be opt-in.
* By default, `v4.x` `Image` resources do _not_ build during previews, but this behavior can be toggled with the `buildOnPreview` option.
* Several users reported outages due to the default behavior allowing bad images to accidentally sneak through CI.
*
* The default behavior of this provider's `Image` resource is similar to `3.x` and will build images during previews.
* This behavior can be changed by specifying `buildOnPreview`.
*
* #### Push behavior
*
* Versions `3.x` and `4.x` of the Pulumi Docker provider attempt to push images to remote registries by default.
* They expose a `skipPush: true` option to disable pushing.
*
* This provider's `Image` resource matches the Docker CLI's behavior and does not push images anywhere by default.
*
* To push images to a registry you can include `push: true` (equivalent to Docker's `--push` flag) or configure an `export` of type `registry` (equivalent to Docker's `--output type=registry`).
* Like Docker, if an image is configured without exports you will see a warning with instructions for how to enable pushing, but the build will still proceed normally.
*
* #### Secrets
*
* Version `3.x` of the Pulumi Docker provider supports secrets by way of the `extraOptions` field.
*
* Version `4.x` of the Pulumi Docker provider does not support secrets.
*
* The `Image` resource supports secrets but does not require those secrets to exist on-disk or in environment variables.
* Instead, they should be passed directly as values.
* (Please be sure to familiarize yourself with Pulumi's [native secret handling](https://www.pulumi.com/docs/concepts/secrets/).)
* Pulumi also provides [ESC](https://www.pulumi.com/product/esc/) to make it easier to share secrets across stacks and environments.
*
* #### Caching
*
* Version `3.x` of the Pulumi Docker provider exposes `cacheFrom: bool | { stages: [...] }`.
* It builds targets individually and pushes them to separate images for caching.
*
* Version `4.x` exposes a similar parameter `cacheFrom: { images: [...] }` which pushes and pulls inline caches.
*
* Both versions 3 and 4 require specific environment variables to be set and deviate from Docker's native caching behavior.
* This can result in inefficient builds due to unnecessary image pulls, repeated file transfers, etc.
*
* The `Image` resource delegates all caching behavior to Docker.
* `cacheFrom` and `cacheTo` options (equivalent to Docker's `--cache-to` and `--cache-from`) are exposed and provide additional cache targets, such as local disk, S3 storage, etc.
*
* #### Outputs
*
* Versions `3.x` and `4.x` of the provider exposed a `repoDigest` output which was a fully qualified tag with digest.
* In `4.x` this could also be a single sha256 hash if the image wasn't pushed.
*
* Unlike earlier providers the `Image` resource can push multiple tags.
* As a convenience, it exposes a `ref` output consisting of a tag with digest as long as the image was pushed.
* If multiple tags were pushed this uses one at random.
*
* If you need more control over tag references you can use the `digest` output, which is always a single sha256 hash as long as the image was exported somewhere.
*
* #### Tag deletion and refreshes
*
* Versions 3 and 4 of Pulumi Docker provider do not delete tags when the `Image` resource is deleted, nor do they confirm expected tags exist during `refresh` operations.
*
* The `buidx.Image` will query your registries during `refresh` to ensure the expected tags exist.
* If any are missing a subsequent `update` will push them.
*
* When a `Image` is deleted, it will _attempt_ to also delete any pushed tags.
* Deletion of remote tags is not guaranteed because not all registries support the manifest `DELETE` API (`docker.io` in particular).
* Manifests are _not_ deleted in the same way during updates -- to do so safely would require a full build to determine whether a Pulumi operation should be an update or update-replace.
*
* Use the [`retainOnDelete: true`](https://www.pulumi.com/docs/concepts/options/retainondelete/) option if you do not want tags deleted.
*
* ### Example migration
*
* Examples of "fully-featured" `v3` and `v4` `Image` resources are shown below, along with an example `Image` resource showing how they would look after migration.
*
* The `v3` resource leverages `buildx` via a `DOCKER_BUILDKIT` environment variable and CLI flags passed in with `extraOption`.
* After migration, the environment variable is no longer needed and CLI flags are now properties on the `Image`.
* In almost all cases, properties of `Image` are named after the Docker CLI flag they correspond to.
*
* The `v4` resource is less functional than its `v3` counterpart because it lacks the flexibility of `extraOptions`.
* It it is shown with parameters similar to the `v3` example for completeness.
*
* ## Example Usage
*
* ## Example Usage
* ### Push to AWS ECR with caching
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ecr.Repository;
* import com.pulumi.aws.ecr.EcrFunctions;
* import com.pulumi.aws.ecr.inputs.GetAuthorizationTokenArgs;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.CacheFromArgs;
* import com.pulumi.dockerbuild.inputs.CacheFromRegistryArgs;
* import com.pulumi.dockerbuild.inputs.CacheToArgs;
* import com.pulumi.dockerbuild.inputs.CacheToRegistryArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import com.pulumi.dockerbuild.inputs.RegistryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ecrRepository = new Repository("ecrRepository");
*
* final var authToken = EcrFunctions.getAuthorizationToken(GetAuthorizationTokenArgs.builder()
* .registryId(ecrRepository.registryId())
* .build());
*
* var myImage = new Image("myImage", ImageArgs.builder()
* .cacheFrom(CacheFromArgs.builder()
* .registry(CacheFromRegistryArgs.builder()
* .ref(ecrRepository.repositoryUrl().applyValue(repositoryUrl -> String.format("%s:cache", repositoryUrl)))
* .build())
* .build())
* .cacheTo(CacheToArgs.builder()
* .registry(CacheToRegistryArgs.builder()
* .imageManifest(true)
* .ociMediaTypes(true)
* .ref(ecrRepository.repositoryUrl().applyValue(repositoryUrl -> String.format("%s:cache", repositoryUrl)))
* .build())
* .build())
* .context(BuildContextArgs.builder()
* .location("./app")
* .build())
* .push(true)
* .registries(RegistryArgs.builder()
* .address(ecrRepository.repositoryUrl())
* .password(authToken.applyValue(getAuthorizationTokenResult -> getAuthorizationTokenResult).applyValue(authToken -> authToken.applyValue(getAuthorizationTokenResult -> getAuthorizationTokenResult.password())))
* .username(authToken.applyValue(getAuthorizationTokenResult -> getAuthorizationTokenResult).applyValue(authToken -> authToken.applyValue(getAuthorizationTokenResult -> getAuthorizationTokenResult.userName())))
* .build())
* .tags(ecrRepository.repositoryUrl().applyValue(repositoryUrl -> String.format("%s:latest", repositoryUrl)))
* .build());
*
* ctx.export("ref", myImage.ref());
* }
* }
* }
*
* ### Multi-platform image
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .platforms(
* "plan9/amd64",
* "plan9/386")
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Registry export
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import com.pulumi.dockerbuild.inputs.RegistryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .push(true)
* .registries(RegistryArgs.builder()
* .address("docker.io")
* .password(dockerHubPassword)
* .username("pulumibot")
* .build())
* .tags("docker.io/pulumi/pulumi:3.107.0")
* .build());
*
* ctx.export("ref", myImage.ref());
* }
* }
* }
*
* ### Caching
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.CacheFromArgs;
* import com.pulumi.dockerbuild.inputs.CacheFromLocalArgs;
* import com.pulumi.dockerbuild.inputs.CacheToArgs;
* import com.pulumi.dockerbuild.inputs.CacheToLocalArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .cacheFrom(CacheFromArgs.builder()
* .local(CacheFromLocalArgs.builder()
* .src("tmp/cache")
* .build())
* .build())
* .cacheTo(CacheToArgs.builder()
* .local(CacheToLocalArgs.builder()
* .dest("tmp/cache")
* .mode("max")
* .build())
* .build())
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Docker Build Cloud
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuilderConfigArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .builder(BuilderConfigArgs.builder()
* .name("cloud-builder-name")
* .build())
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .exec(true)
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Build arguments
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .buildArgs(Map.of("SET_ME_TO_TRUE", "true"))
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Build target
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .push(false)
* .target("build-me")
* .build());
*
* }
* }
* }
*
* ### Named contexts
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("app")
* .named(Map.of("golang:latest", Map.of("location", "docker-image://golang{@literal @}sha256:b8e62cf593cdaff36efd90aa3a37de268e6781a2e68c6610940c48f7cdf36984")))
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Remote context
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("https://raw.githubusercontent.com/pulumi/pulumi-docker/api-types/provider/testdata/Dockerfile")
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Inline Dockerfile
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import com.pulumi.dockerbuild.inputs.DockerfileArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .dockerfile(DockerfileArgs.builder()
* .inline("""
* FROM busybox
* COPY hello.c ./
* """)
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Remote context
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import com.pulumi.dockerbuild.inputs.DockerfileArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("https://github.com/docker-library/hello-world.git")
* .build())
* .dockerfile(DockerfileArgs.builder()
* .location("app/Dockerfile")
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
* ### Local export
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.dockerbuild.Image;
* import com.pulumi.dockerbuild.ImageArgs;
* import com.pulumi.dockerbuild.inputs.BuildContextArgs;
* import com.pulumi.dockerbuild.inputs.ExportArgs;
* import com.pulumi.dockerbuild.inputs.ExportDockerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var image = new Image("image", ImageArgs.builder()
* .context(BuildContextArgs.builder()
* .location("app")
* .build())
* .exports(ExportArgs.builder()
* .docker(ExportDockerArgs.builder()
* .tar(true)
* .build())
* .build())
* .push(false)
* .build());
*
* }
* }
* }
*
*
*/
@ResourceType(type="docker-build:index:Image")
public class Image extends com.pulumi.resources.CustomResource {
/**
* Custom `host:ip` mappings to use during the build.
*
* Equivalent to Docker's `--add-host` flag.
*
*/
@Export(name="addHosts", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> addHosts;
/**
* @return Custom `host:ip` mappings to use during the build.
*
* Equivalent to Docker's `--add-host` flag.
*
*/
public Output>> addHosts() {
return Codegen.optional(this.addHosts);
}
/**
* `ARG` names and values to set during the build.
*
* These variables are accessed like environment variables inside `RUN`
* instructions.
*
* Build arguments are persisted in the image, so you should use `secrets`
* if these arguments are sensitive.
*
* Equivalent to Docker's `--build-arg` flag.
*
*/
@Export(name="buildArgs", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> buildArgs;
/**
* @return `ARG` names and values to set during the build.
*
* These variables are accessed like environment variables inside `RUN`
* instructions.
*
* Build arguments are persisted in the image, so you should use `secrets`
* if these arguments are sensitive.
*
* Equivalent to Docker's `--build-arg` flag.
*
*/
public Output>> buildArgs() {
return Codegen.optional(this.buildArgs);
}
/**
* Setting this to `false` will always skip image builds during previews,
* and setting it to `true` will always build images during previews.
*
* Images built during previews are never exported to registries, however
* cache manifests are still exported.
*
* On-disk Dockerfiles are always validated for syntactic correctness
* regardless of this setting.
*
* Defaults to `true` as a safeguard against broken images merging as part
* of CI pipelines.
*
*/
@Export(name="buildOnPreview", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> buildOnPreview;
/**
* @return Setting this to `false` will always skip image builds during previews,
* and setting it to `true` will always build images during previews.
*
* Images built during previews are never exported to registries, however
* cache manifests are still exported.
*
* On-disk Dockerfiles are always validated for syntactic correctness
* regardless of this setting.
*
* Defaults to `true` as a safeguard against broken images merging as part
* of CI pipelines.
*
*/
public Output> buildOnPreview() {
return Codegen.optional(this.buildOnPreview);
}
/**
* Builder configuration.
*
*/
@Export(name="builder", refs={BuilderConfig.class}, tree="[0]")
private Output* @Nullable */ BuilderConfig> builder;
/**
* @return Builder configuration.
*
*/
public Output> builder_() {
return Codegen.optional(this.builder);
}
/**
* Cache export configuration.
*
* Equivalent to Docker's `--cache-from` flag.
*
*/
@Export(name="cacheFrom", refs={List.class,CacheFrom.class}, tree="[0,1]")
private Output* @Nullable */ List> cacheFrom;
/**
* @return Cache export configuration.
*
* Equivalent to Docker's `--cache-from` flag.
*
*/
public Output>> cacheFrom() {
return Codegen.optional(this.cacheFrom);
}
/**
* Cache import configuration.
*
* Equivalent to Docker's `--cache-to` flag.
*
*/
@Export(name="cacheTo", refs={List.class,CacheTo.class}, tree="[0,1]")
private Output* @Nullable */ List> cacheTo;
/**
* @return Cache import configuration.
*
* Equivalent to Docker's `--cache-to` flag.
*
*/
public Output>> cacheTo() {
return Codegen.optional(this.cacheTo);
}
/**
* Build context settings. Defaults to the current directory.
*
* Equivalent to Docker's `PATH | URL | -` positional argument.
*
*/
@Export(name="context", refs={BuildContext.class}, tree="[0]")
private Output* @Nullable */ BuildContext> context;
/**
* @return Build context settings. Defaults to the current directory.
*
* Equivalent to Docker's `PATH | URL | -` positional argument.
*
*/
public Output> context() {
return Codegen.optional(this.context);
}
/**
* A preliminary hash of the image's build context.
*
* Pulumi uses this to determine if an image _may_ need to be re-built.
*
*/
@Export(name="contextHash", refs={String.class}, tree="[0]")
private Output contextHash;
/**
* @return A preliminary hash of the image's build context.
*
* Pulumi uses this to determine if an image _may_ need to be re-built.
*
*/
public Output contextHash() {
return this.contextHash;
}
/**
* A SHA256 digest of the image if it was exported to a registry or
* elsewhere.
*
* Empty if the image was not exported.
*
* Registry images can be referenced precisely as `<tag>{@literal @}<digest>`. The
* `ref` output provides one such reference as a convenience.
*
*/
@Export(name="digest", refs={String.class}, tree="[0]")
private Output digest;
/**
* @return A SHA256 digest of the image if it was exported to a registry or
* elsewhere.
*
* Empty if the image was not exported.
*
* Registry images can be referenced precisely as `<tag>{@literal @}<digest>`. The
* `ref` output provides one such reference as a convenience.
*
*/
public Output digest() {
return this.digest;
}
/**
* Dockerfile settings.
*
* Equivalent to Docker's `--file` flag.
*
*/
@Export(name="dockerfile", refs={Dockerfile.class}, tree="[0]")
private Output* @Nullable */ Dockerfile> dockerfile;
/**
* @return Dockerfile settings.
*
* Equivalent to Docker's `--file` flag.
*
*/
public Output> dockerfile() {
return Codegen.optional(this.dockerfile);
}
/**
* Use `exec` mode to build this image.
*
* By default the provider embeds a v25 Docker client with v0.12 buildx
* support. This helps ensure consistent behavior across environments and
* is compatible with alternative build backends (e.g. `buildkitd`), but
* it may not be desirable if you require a specific version of buildx.
* For example you may want to run a custom `docker-buildx` binary with
* support for [Docker Build
* Cloud](https://docs.docker.com/build/cloud/setup/) (DBC).
*
* When this is set to `true` the provider will instead execute the
* `docker-buildx` binary directly to perform its operations. The user is
* responsible for ensuring this binary exists, with correct permissions
* and pre-configured builders, at a path Docker expects (e.g.
* `~/.docker/cli-plugins`).
*
* Debugging `exec` mode may be more difficult as Pulumi will not be able
* to surface fine-grained errors and warnings. Additionally credentials
* are temporarily written to disk in order to provide them to the
* `docker-buildx` binary.
*
*/
@Export(name="exec", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> exec;
/**
* @return Use `exec` mode to build this image.
*
* By default the provider embeds a v25 Docker client with v0.12 buildx
* support. This helps ensure consistent behavior across environments and
* is compatible with alternative build backends (e.g. `buildkitd`), but
* it may not be desirable if you require a specific version of buildx.
* For example you may want to run a custom `docker-buildx` binary with
* support for [Docker Build
* Cloud](https://docs.docker.com/build/cloud/setup/) (DBC).
*
* When this is set to `true` the provider will instead execute the
* `docker-buildx` binary directly to perform its operations. The user is
* responsible for ensuring this binary exists, with correct permissions
* and pre-configured builders, at a path Docker expects (e.g.
* `~/.docker/cli-plugins`).
*
* Debugging `exec` mode may be more difficult as Pulumi will not be able
* to surface fine-grained errors and warnings. Additionally credentials
* are temporarily written to disk in order to provide them to the
* `docker-buildx` binary.
*
*/
public Output> exec() {
return Codegen.optional(this.exec);
}
/**
* Controls where images are persisted after building.
*
* Images are only stored in the local cache unless `exports` are
* explicitly configured.
*
* Exporting to multiple destinations requires a daemon running BuildKit
* 0.13 or later.
*
* Equivalent to Docker's `--output` flag.
*
*/
@Export(name="exports", refs={List.class,com.pulumi.dockerbuild.outputs.Export.class}, tree="[0,1]")
private Output* @Nullable */ List> exports;
/**
* @return Controls where images are persisted after building.
*
* Images are only stored in the local cache unless `exports` are
* explicitly configured.
*
* Exporting to multiple destinations requires a daemon running BuildKit
* 0.13 or later.
*
* Equivalent to Docker's `--output` flag.
*
*/
public Output>> exports() {
return Codegen.optional(this.exports);
}
/**
* Attach arbitrary key/value metadata to the image.
*
* Equivalent to Docker's `--label` flag.
*
*/
@Export(name="labels", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> labels;
/**
* @return Attach arbitrary key/value metadata to the image.
*
* Equivalent to Docker's `--label` flag.
*
*/
public Output>> labels() {
return Codegen.optional(this.labels);
}
/**
* When `true` the build will automatically include a `docker` export.
*
* Defaults to `false`.
*
* Equivalent to Docker's `--load` flag.
*
*/
@Export(name="load", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> load;
/**
* @return When `true` the build will automatically include a `docker` export.
*
* Defaults to `false`.
*
* Equivalent to Docker's `--load` flag.
*
*/
public Output> load() {
return Codegen.optional(this.load);
}
/**
* Set the network mode for `RUN` instructions. Defaults to `default`.
*
* For custom networks, configure your builder with `--driver-opt network=...`.
*
* Equivalent to Docker's `--network` flag.
*
*/
@Export(name="network", refs={NetworkMode.class}, tree="[0]")
private Output* @Nullable */ NetworkMode> network;
/**
* @return Set the network mode for `RUN` instructions. Defaults to `default`.
*
* For custom networks, configure your builder with `--driver-opt network=...`.
*
* Equivalent to Docker's `--network` flag.
*
*/
public Output> network() {
return Codegen.optional(this.network);
}
/**
* Do not import cache manifests when building the image.
*
* Equivalent to Docker's `--no-cache` flag.
*
*/
@Export(name="noCache", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> noCache;
/**
* @return Do not import cache manifests when building the image.
*
* Equivalent to Docker's `--no-cache` flag.
*
*/
public Output> noCache() {
return Codegen.optional(this.noCache);
}
/**
* Set target platform(s) for the build. Defaults to the host's platform.
*
* Equivalent to Docker's `--platform` flag.
*
*/
@Export(name="platforms", refs={List.class,Platform.class}, tree="[0,1]")
private Output* @Nullable */ List> platforms;
/**
* @return Set target platform(s) for the build. Defaults to the host's platform.
*
* Equivalent to Docker's `--platform` flag.
*
*/
public Output>> platforms() {
return Codegen.optional(this.platforms);
}
/**
* Always pull referenced images.
*
* Equivalent to Docker's `--pull` flag.
*
*/
@Export(name="pull", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> pull;
/**
* @return Always pull referenced images.
*
* Equivalent to Docker's `--pull` flag.
*
*/
public Output> pull() {
return Codegen.optional(this.pull);
}
/**
* When `true` the build will automatically include a `registry` export.
*
* Defaults to `false`.
*
* Equivalent to Docker's `--push` flag.
*
*/
@Export(name="push", refs={Boolean.class}, tree="[0]")
private Output push;
/**
* @return When `true` the build will automatically include a `registry` export.
*
* Defaults to `false`.
*
* Equivalent to Docker's `--push` flag.
*
*/
public Output push() {
return this.push;
}
/**
* If the image was pushed to any registries then this will contain a
* single fully-qualified tag including the build's digest.
*
* If the image had tags but was not exported, this will take on a value
* of one of those tags.
*
* This will be empty if the image had no exports and no tags.
*
* This is only for convenience and may not be appropriate for situations
* where multiple tags or registries are involved. In those cases this
* output is not guaranteed to be stable.
*
* For more control over tags consumed by downstream resources you should
* use the `digest` output.
*
*/
@Export(name="ref", refs={String.class}, tree="[0]")
private Output ref;
/**
* @return If the image was pushed to any registries then this will contain a
* single fully-qualified tag including the build's digest.
*
* If the image had tags but was not exported, this will take on a value
* of one of those tags.
*
* This will be empty if the image had no exports and no tags.
*
* This is only for convenience and may not be appropriate for situations
* where multiple tags or registries are involved. In those cases this
* output is not guaranteed to be stable.
*
* For more control over tags consumed by downstream resources you should
* use the `digest` output.
*
*/
public Output ref() {
return this.ref;
}
/**
* Registry credentials. Required if reading or exporting to private
* repositories.
*
* Credentials are kept in-memory and do not pollute pre-existing
* credentials on the host.
*
* Similar to `docker login`.
*
*/
@Export(name="registries", refs={List.class,Registry.class}, tree="[0,1]")
private Output* @Nullable */ List> registries;
/**
* @return Registry credentials. Required if reading or exporting to private
* repositories.
*
* Credentials are kept in-memory and do not pollute pre-existing
* credentials on the host.
*
* Similar to `docker login`.
*
*/
public Output>> registries() {
return Codegen.optional(this.registries);
}
/**
* A mapping of secret names to their corresponding values.
*
* Unlike the Docker CLI, these can be passed by value and do not need to
* exist on-disk or in environment variables.
*
* Build arguments and environment variables are persistent in the final
* image, so you should use this for sensitive values.
*
* Similar to Docker's `--secret` flag.
*
*/
@Export(name="secrets", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> secrets;
/**
* @return A mapping of secret names to their corresponding values.
*
* Unlike the Docker CLI, these can be passed by value and do not need to
* exist on-disk or in environment variables.
*
* Build arguments and environment variables are persistent in the final
* image, so you should use this for sensitive values.
*
* Similar to Docker's `--secret` flag.
*
*/
public Output>> secrets() {
return Codegen.optional(this.secrets);
}
/**
* SSH agent socket or keys to expose to the build.
*
* Equivalent to Docker's `--ssh` flag.
*
*/
@Export(name="ssh", refs={List.class,SSH.class}, tree="[0,1]")
private Output* @Nullable */ List> ssh;
/**
* @return SSH agent socket or keys to expose to the build.
*
* Equivalent to Docker's `--ssh` flag.
*
*/
public Output>> ssh() {
return Codegen.optional(this.ssh);
}
/**
* Name and optionally a tag (format: `name:tag`).
*
* If exporting to a registry, the name should include the fully qualified
* registry address (e.g. `docker.io/pulumi/pulumi:latest`).
*
* Equivalent to Docker's `--tag` flag.
*
*/
@Export(name="tags", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> tags;
/**
* @return Name and optionally a tag (format: `name:tag`).
*
* If exporting to a registry, the name should include the fully qualified
* registry address (e.g. `docker.io/pulumi/pulumi:latest`).
*
* Equivalent to Docker's `--tag` flag.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Set the target build stage(s) to build.
*
* If not specified all targets will be built by default.
*
* Equivalent to Docker's `--target` flag.
*
*/
@Export(name="target", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> target;
/**
* @return Set the target build stage(s) to build.
*
* If not specified all targets will be built by default.
*
* Equivalent to Docker's `--target` flag.
*
*/
public Output> target() {
return Codegen.optional(this.target);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Image(String name) {
this(name, ImageArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Image(String name, ImageArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Image(String name, ImageArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("docker-build:index:Image", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()));
}
private Image(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("docker-build:index:Image", name, null, makeResourceOptions(options, id));
}
private static ImageArgs makeArgs(ImageArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ImageArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Image get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Image(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy