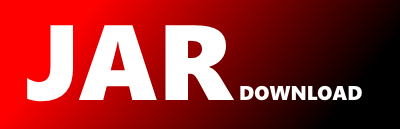
com.pulumi.dockerbuild.inputs.CacheToArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.dockerbuild.inputs.CacheToAzureBlobArgs;
import com.pulumi.dockerbuild.inputs.CacheToGitHubActionsArgs;
import com.pulumi.dockerbuild.inputs.CacheToInlineArgs;
import com.pulumi.dockerbuild.inputs.CacheToLocalArgs;
import com.pulumi.dockerbuild.inputs.CacheToRegistryArgs;
import com.pulumi.dockerbuild.inputs.CacheToS3Args;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CacheToArgs extends com.pulumi.resources.ResourceArgs {
public static final CacheToArgs Empty = new CacheToArgs();
/**
* Push cache to Azure's blob storage service.
*
*/
@Import(name="azblob")
private @Nullable Output azblob;
/**
* @return Push cache to Azure's blob storage service.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy