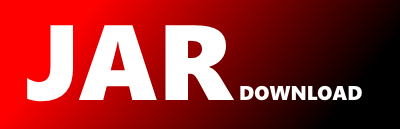
com.pulumi.dockerbuild.inputs.ExportArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.dockerbuild.inputs.ExportCacheOnlyArgs;
import com.pulumi.dockerbuild.inputs.ExportDockerArgs;
import com.pulumi.dockerbuild.inputs.ExportImageArgs;
import com.pulumi.dockerbuild.inputs.ExportLocalArgs;
import com.pulumi.dockerbuild.inputs.ExportOCIArgs;
import com.pulumi.dockerbuild.inputs.ExportRegistryArgs;
import com.pulumi.dockerbuild.inputs.ExportTarArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ExportArgs extends com.pulumi.resources.ResourceArgs {
public static final ExportArgs Empty = new ExportArgs();
/**
* A no-op export. Helpful for silencing the 'no exports' warning if you
* just want to populate caches.
*
*/
@Import(name="cacheonly")
private @Nullable Output cacheonly;
/**
* @return A no-op export. Helpful for silencing the 'no exports' warning if you
* just want to populate caches.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy