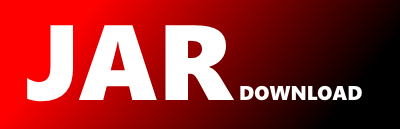
com.pulumi.dockerbuild.inputs.ExportImageArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.dockerbuild.enums.CompressionType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ExportImageArgs extends com.pulumi.resources.ResourceArgs {
public static final ExportImageArgs Empty = new ExportImageArgs();
/**
* Attach an arbitrary key/value annotation to the image.
*
*/
@Import(name="annotations")
private @Nullable Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy