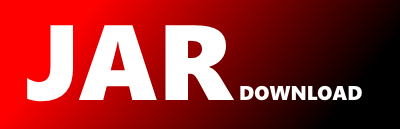
com.pulumi.dockerbuild.outputs.CacheFrom Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.dockerbuild.outputs.CacheFromAzureBlob;
import com.pulumi.dockerbuild.outputs.CacheFromGitHubActions;
import com.pulumi.dockerbuild.outputs.CacheFromLocal;
import com.pulumi.dockerbuild.outputs.CacheFromRegistry;
import com.pulumi.dockerbuild.outputs.CacheFromS3;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CacheFrom {
/**
* @return Upload build caches to Azure's blob storage service.
*
*/
private @Nullable CacheFromAzureBlob azblob;
/**
* @return When `true` this entry will be excluded. Defaults to `false`.
*
*/
private @Nullable Boolean disabled;
/**
* @return Recommended for use with GitHub Actions workflows.
*
* An action like `crazy-max/ghaction-github-runtime` is recommended to
* expose appropriate credentials to your GitHub workflow.
*
*/
private @Nullable CacheFromGitHubActions gha;
/**
* @return A simple backend which caches images on your local filesystem.
*
*/
private @Nullable CacheFromLocal local;
/**
* @return A raw string as you would provide it to the Docker CLI (e.g.,
* `type=inline`).
*
*/
private @Nullable String raw;
/**
* @return Upload build caches to remote registries.
*
*/
private @Nullable CacheFromRegistry registry;
/**
* @return Upload build caches to AWS S3 or an S3-compatible services such as
* MinIO.
*
*/
private @Nullable CacheFromS3 s3;
private CacheFrom() {}
/**
* @return Upload build caches to Azure's blob storage service.
*
*/
public Optional azblob() {
return Optional.ofNullable(this.azblob);
}
/**
* @return When `true` this entry will be excluded. Defaults to `false`.
*
*/
public Optional disabled() {
return Optional.ofNullable(this.disabled);
}
/**
* @return Recommended for use with GitHub Actions workflows.
*
* An action like `crazy-max/ghaction-github-runtime` is recommended to
* expose appropriate credentials to your GitHub workflow.
*
*/
public Optional gha() {
return Optional.ofNullable(this.gha);
}
/**
* @return A simple backend which caches images on your local filesystem.
*
*/
public Optional local() {
return Optional.ofNullable(this.local);
}
/**
* @return A raw string as you would provide it to the Docker CLI (e.g.,
* `type=inline`).
*
*/
public Optional raw() {
return Optional.ofNullable(this.raw);
}
/**
* @return Upload build caches to remote registries.
*
*/
public Optional registry() {
return Optional.ofNullable(this.registry);
}
/**
* @return Upload build caches to AWS S3 or an S3-compatible services such as
* MinIO.
*
*/
public Optional s3() {
return Optional.ofNullable(this.s3);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CacheFrom defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable CacheFromAzureBlob azblob;
private @Nullable Boolean disabled;
private @Nullable CacheFromGitHubActions gha;
private @Nullable CacheFromLocal local;
private @Nullable String raw;
private @Nullable CacheFromRegistry registry;
private @Nullable CacheFromS3 s3;
public Builder() {}
public Builder(CacheFrom defaults) {
Objects.requireNonNull(defaults);
this.azblob = defaults.azblob;
this.disabled = defaults.disabled;
this.gha = defaults.gha;
this.local = defaults.local;
this.raw = defaults.raw;
this.registry = defaults.registry;
this.s3 = defaults.s3;
}
@CustomType.Setter
public Builder azblob(@Nullable CacheFromAzureBlob azblob) {
this.azblob = azblob;
return this;
}
@CustomType.Setter
public Builder disabled(@Nullable Boolean disabled) {
this.disabled = disabled;
return this;
}
@CustomType.Setter
public Builder gha(@Nullable CacheFromGitHubActions gha) {
this.gha = gha;
return this;
}
@CustomType.Setter
public Builder local(@Nullable CacheFromLocal local) {
this.local = local;
return this;
}
@CustomType.Setter
public Builder raw(@Nullable String raw) {
this.raw = raw;
return this;
}
@CustomType.Setter
public Builder registry(@Nullable CacheFromRegistry registry) {
this.registry = registry;
return this;
}
@CustomType.Setter
public Builder s3(@Nullable CacheFromS3 s3) {
this.s3 = s3;
return this;
}
public CacheFrom build() {
final var _resultValue = new CacheFrom();
_resultValue.azblob = azblob;
_resultValue.disabled = disabled;
_resultValue.gha = gha;
_resultValue.local = local;
_resultValue.raw = raw;
_resultValue.registry = registry;
_resultValue.s3 = s3;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy