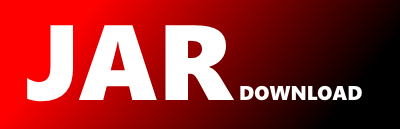
com.pulumi.dockerbuild.outputs.CacheToLocal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.dockerbuild.enums.CacheMode;
import com.pulumi.dockerbuild.enums.CompressionType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CacheToLocal {
/**
* @return The compression type to use.
*
*/
private @Nullable CompressionType compression;
/**
* @return Compression level from 0 to 22.
*
*/
private @Nullable Integer compressionLevel;
/**
* @return Path of the local directory to export the cache.
*
*/
private String dest;
/**
* @return Forcefully apply compression.
*
*/
private @Nullable Boolean forceCompression;
/**
* @return Ignore errors caused by failed cache exports.
*
*/
private @Nullable Boolean ignoreError;
/**
* @return The cache mode to use. Defaults to `min`.
*
*/
private @Nullable CacheMode mode;
private CacheToLocal() {}
/**
* @return The compression type to use.
*
*/
public Optional compression() {
return Optional.ofNullable(this.compression);
}
/**
* @return Compression level from 0 to 22.
*
*/
public Optional compressionLevel() {
return Optional.ofNullable(this.compressionLevel);
}
/**
* @return Path of the local directory to export the cache.
*
*/
public String dest() {
return this.dest;
}
/**
* @return Forcefully apply compression.
*
*/
public Optional forceCompression() {
return Optional.ofNullable(this.forceCompression);
}
/**
* @return Ignore errors caused by failed cache exports.
*
*/
public Optional ignoreError() {
return Optional.ofNullable(this.ignoreError);
}
/**
* @return The cache mode to use. Defaults to `min`.
*
*/
public Optional mode() {
return Optional.ofNullable(this.mode);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CacheToLocal defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable CompressionType compression;
private @Nullable Integer compressionLevel;
private String dest;
private @Nullable Boolean forceCompression;
private @Nullable Boolean ignoreError;
private @Nullable CacheMode mode;
public Builder() {}
public Builder(CacheToLocal defaults) {
Objects.requireNonNull(defaults);
this.compression = defaults.compression;
this.compressionLevel = defaults.compressionLevel;
this.dest = defaults.dest;
this.forceCompression = defaults.forceCompression;
this.ignoreError = defaults.ignoreError;
this.mode = defaults.mode;
}
@CustomType.Setter
public Builder compression(@Nullable CompressionType compression) {
this.compression = compression;
return this;
}
@CustomType.Setter
public Builder compressionLevel(@Nullable Integer compressionLevel) {
this.compressionLevel = compressionLevel;
return this;
}
@CustomType.Setter
public Builder dest(String dest) {
if (dest == null) {
throw new MissingRequiredPropertyException("CacheToLocal", "dest");
}
this.dest = dest;
return this;
}
@CustomType.Setter
public Builder forceCompression(@Nullable Boolean forceCompression) {
this.forceCompression = forceCompression;
return this;
}
@CustomType.Setter
public Builder ignoreError(@Nullable Boolean ignoreError) {
this.ignoreError = ignoreError;
return this;
}
@CustomType.Setter
public Builder mode(@Nullable CacheMode mode) {
this.mode = mode;
return this;
}
public CacheToLocal build() {
final var _resultValue = new CacheToLocal();
_resultValue.compression = compression;
_resultValue.compressionLevel = compressionLevel;
_resultValue.dest = dest;
_resultValue.forceCompression = forceCompression;
_resultValue.ignoreError = ignoreError;
_resultValue.mode = mode;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy