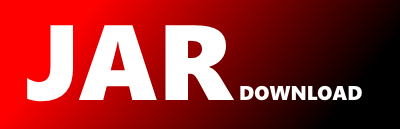
com.pulumi.dockerbuild.outputs.Export Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-build Show documentation
Show all versions of docker-build Show documentation
A Pulumi provider for building modern Docker images with buildx and BuildKit.
// *** WARNING: this file was generated by pulumi. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.dockerbuild.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.dockerbuild.outputs.ExportCacheOnly;
import com.pulumi.dockerbuild.outputs.ExportDocker;
import com.pulumi.dockerbuild.outputs.ExportImage;
import com.pulumi.dockerbuild.outputs.ExportLocal;
import com.pulumi.dockerbuild.outputs.ExportOCI;
import com.pulumi.dockerbuild.outputs.ExportRegistry;
import com.pulumi.dockerbuild.outputs.ExportTar;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class Export {
/**
* @return A no-op export. Helpful for silencing the 'no exports' warning if you
* just want to populate caches.
*
*/
private @Nullable ExportCacheOnly cacheonly;
/**
* @return When `true` this entry will be excluded. Defaults to `false`.
*
*/
private @Nullable Boolean disabled;
/**
* @return Export as a Docker image layout.
*
*/
private @Nullable ExportDocker docker;
/**
* @return Outputs the build result into a container image format.
*
*/
private @Nullable ExportImage image;
/**
* @return Export to a local directory as files and directories.
*
*/
private @Nullable ExportLocal local;
/**
* @return Identical to the Docker exporter but uses OCI media types by default.
*
*/
private @Nullable ExportOCI oci;
/**
* @return A raw string as you would provide it to the Docker CLI (e.g.,
* `type=docker`)
*
*/
private @Nullable String raw;
/**
* @return Identical to the Image exporter, but pushes by default.
*
*/
private @Nullable ExportRegistry registry;
/**
* @return Export to a local directory as a tarball.
*
*/
private @Nullable ExportTar tar;
private Export() {}
/**
* @return A no-op export. Helpful for silencing the 'no exports' warning if you
* just want to populate caches.
*
*/
public Optional cacheonly() {
return Optional.ofNullable(this.cacheonly);
}
/**
* @return When `true` this entry will be excluded. Defaults to `false`.
*
*/
public Optional disabled() {
return Optional.ofNullable(this.disabled);
}
/**
* @return Export as a Docker image layout.
*
*/
public Optional docker() {
return Optional.ofNullable(this.docker);
}
/**
* @return Outputs the build result into a container image format.
*
*/
public Optional image() {
return Optional.ofNullable(this.image);
}
/**
* @return Export to a local directory as files and directories.
*
*/
public Optional local() {
return Optional.ofNullable(this.local);
}
/**
* @return Identical to the Docker exporter but uses OCI media types by default.
*
*/
public Optional oci() {
return Optional.ofNullable(this.oci);
}
/**
* @return A raw string as you would provide it to the Docker CLI (e.g.,
* `type=docker`)
*
*/
public Optional raw() {
return Optional.ofNullable(this.raw);
}
/**
* @return Identical to the Image exporter, but pushes by default.
*
*/
public Optional registry() {
return Optional.ofNullable(this.registry);
}
/**
* @return Export to a local directory as a tarball.
*
*/
public Optional tar() {
return Optional.ofNullable(this.tar);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(Export defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ExportCacheOnly cacheonly;
private @Nullable Boolean disabled;
private @Nullable ExportDocker docker;
private @Nullable ExportImage image;
private @Nullable ExportLocal local;
private @Nullable ExportOCI oci;
private @Nullable String raw;
private @Nullable ExportRegistry registry;
private @Nullable ExportTar tar;
public Builder() {}
public Builder(Export defaults) {
Objects.requireNonNull(defaults);
this.cacheonly = defaults.cacheonly;
this.disabled = defaults.disabled;
this.docker = defaults.docker;
this.image = defaults.image;
this.local = defaults.local;
this.oci = defaults.oci;
this.raw = defaults.raw;
this.registry = defaults.registry;
this.tar = defaults.tar;
}
@CustomType.Setter
public Builder cacheonly(@Nullable ExportCacheOnly cacheonly) {
this.cacheonly = cacheonly;
return this;
}
@CustomType.Setter
public Builder disabled(@Nullable Boolean disabled) {
this.disabled = disabled;
return this;
}
@CustomType.Setter
public Builder docker(@Nullable ExportDocker docker) {
this.docker = docker;
return this;
}
@CustomType.Setter
public Builder image(@Nullable ExportImage image) {
this.image = image;
return this;
}
@CustomType.Setter
public Builder local(@Nullable ExportLocal local) {
this.local = local;
return this;
}
@CustomType.Setter
public Builder oci(@Nullable ExportOCI oci) {
this.oci = oci;
return this;
}
@CustomType.Setter
public Builder raw(@Nullable String raw) {
this.raw = raw;
return this;
}
@CustomType.Setter
public Builder registry(@Nullable ExportRegistry registry) {
this.registry = registry;
return this;
}
@CustomType.Setter
public Builder tar(@Nullable ExportTar tar) {
this.tar = tar;
return this;
}
public Export build() {
final var _resultValue = new Export();
_resultValue.cacheonly = cacheonly;
_resultValue.disabled = disabled;
_resultValue.docker = docker;
_resultValue.image = image;
_resultValue.local = local;
_resultValue.oci = oci;
_resultValue.raw = raw;
_resultValue.registry = registry;
_resultValue.tar = tar;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy