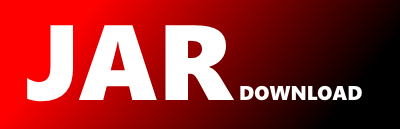
com.pulumi.github.outputs.GetTeamResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.github.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.github.outputs.GetTeamRepositoriesDetailed;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetTeamResult {
/**
* @return the team's description.
*
*/
private String description;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return List of team members (list of GitHub usernames). Not returned if `summary_only = true`
*
*/
private List members;
private @Nullable String membershipType;
/**
* @return the team's full name.
*
*/
private String name;
/**
* @return the Node ID of the team.
*
*/
private String nodeId;
/**
* @return the team's permission level.
*
*/
private String permission;
/**
* @return the team's privacy type.
*
*/
private String privacy;
/**
* @return List of team repositories (list of repo names). Not returned if `summary_only = true`
*
*/
private List repositories;
/**
* @return List of team repositories (list of `repo_id` and `role_name`). Not returned if `summary_only = true`
*
*/
private List repositoriesDetaileds;
private @Nullable Integer resultsPerPage;
private String slug;
private @Nullable Boolean summaryOnly;
private GetTeamResult() {}
/**
* @return the team's description.
*
*/
public String description() {
return this.description;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return List of team members (list of GitHub usernames). Not returned if `summary_only = true`
*
*/
public List members() {
return this.members;
}
public Optional membershipType() {
return Optional.ofNullable(this.membershipType);
}
/**
* @return the team's full name.
*
*/
public String name() {
return this.name;
}
/**
* @return the Node ID of the team.
*
*/
public String nodeId() {
return this.nodeId;
}
/**
* @return the team's permission level.
*
*/
public String permission() {
return this.permission;
}
/**
* @return the team's privacy type.
*
*/
public String privacy() {
return this.privacy;
}
/**
* @return List of team repositories (list of repo names). Not returned if `summary_only = true`
*
*/
public List repositories() {
return this.repositories;
}
/**
* @return List of team repositories (list of `repo_id` and `role_name`). Not returned if `summary_only = true`
*
*/
public List repositoriesDetaileds() {
return this.repositoriesDetaileds;
}
public Optional resultsPerPage() {
return Optional.ofNullable(this.resultsPerPage);
}
public String slug() {
return this.slug;
}
public Optional summaryOnly() {
return Optional.ofNullable(this.summaryOnly);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetTeamResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String description;
private String id;
private List members;
private @Nullable String membershipType;
private String name;
private String nodeId;
private String permission;
private String privacy;
private List repositories;
private List repositoriesDetaileds;
private @Nullable Integer resultsPerPage;
private String slug;
private @Nullable Boolean summaryOnly;
public Builder() {}
public Builder(GetTeamResult defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.id = defaults.id;
this.members = defaults.members;
this.membershipType = defaults.membershipType;
this.name = defaults.name;
this.nodeId = defaults.nodeId;
this.permission = defaults.permission;
this.privacy = defaults.privacy;
this.repositories = defaults.repositories;
this.repositoriesDetaileds = defaults.repositoriesDetaileds;
this.resultsPerPage = defaults.resultsPerPage;
this.slug = defaults.slug;
this.summaryOnly = defaults.summaryOnly;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder id(String id) {
this.id = Objects.requireNonNull(id);
return this;
}
@CustomType.Setter
public Builder members(List members) {
this.members = Objects.requireNonNull(members);
return this;
}
public Builder members(String... members) {
return members(List.of(members));
}
@CustomType.Setter
public Builder membershipType(@Nullable String membershipType) {
this.membershipType = membershipType;
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder nodeId(String nodeId) {
this.nodeId = Objects.requireNonNull(nodeId);
return this;
}
@CustomType.Setter
public Builder permission(String permission) {
this.permission = Objects.requireNonNull(permission);
return this;
}
@CustomType.Setter
public Builder privacy(String privacy) {
this.privacy = Objects.requireNonNull(privacy);
return this;
}
@CustomType.Setter
public Builder repositories(List repositories) {
this.repositories = Objects.requireNonNull(repositories);
return this;
}
public Builder repositories(String... repositories) {
return repositories(List.of(repositories));
}
@CustomType.Setter
public Builder repositoriesDetaileds(List repositoriesDetaileds) {
this.repositoriesDetaileds = Objects.requireNonNull(repositoriesDetaileds);
return this;
}
public Builder repositoriesDetaileds(GetTeamRepositoriesDetailed... repositoriesDetaileds) {
return repositoriesDetaileds(List.of(repositoriesDetaileds));
}
@CustomType.Setter
public Builder resultsPerPage(@Nullable Integer resultsPerPage) {
this.resultsPerPage = resultsPerPage;
return this;
}
@CustomType.Setter
public Builder slug(String slug) {
this.slug = Objects.requireNonNull(slug);
return this;
}
@CustomType.Setter
public Builder summaryOnly(@Nullable Boolean summaryOnly) {
this.summaryOnly = summaryOnly;
return this;
}
public GetTeamResult build() {
final var o = new GetTeamResult();
o.description = description;
o.id = id;
o.members = members;
o.membershipType = membershipType;
o.name = name;
o.nodeId = nodeId;
o.permission = permission;
o.privacy = privacy;
o.repositories = repositories;
o.repositoriesDetaileds = repositoriesDetaileds;
o.resultsPerPage = resultsPerPage;
o.slug = slug;
o.summaryOnly = summaryOnly;
return o;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy