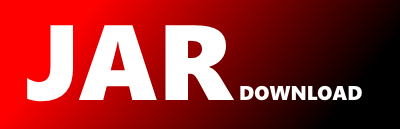
com.pulumi.github.inputs.RepositoryPullRequestState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of github Show documentation
Show all versions of github Show documentation
A Pulumi package for creating and managing github cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.github.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RepositoryPullRequestState extends com.pulumi.resources.ResourceArgs {
public static final RepositoryPullRequestState Empty = new RepositoryPullRequestState();
/**
* Name of the branch serving as the base of the Pull Request.
*
*/
@Import(name="baseRef")
private @Nullable Output baseRef;
/**
* @return Name of the branch serving as the base of the Pull Request.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy