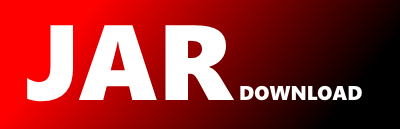
com.pulumi.github.outputs.GetRepositoryPullRequestsResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.github.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetRepositoryPullRequestsResult {
/**
* @return If set, filters Pull Requests by base branch name.
*
*/
private String baseRef;
/**
* @return Head commit SHA of the Pull Request base.
*
*/
private String baseSha;
/**
* @return Body of the Pull Request.
*
*/
private String body;
/**
* @return Indicates Whether this Pull Request is a draft.
*
*/
private Boolean draft;
/**
* @return Owner of the Pull Request head repository.
*
*/
private String headOwner;
/**
* @return If set, filters Pull Requests by head user or head organization and branch name in the format of "user:ref-name" or "organization:ref-name". For example: "github:new-script-format" or "octocat:test-branch".
*
*/
private String headRef;
/**
* @return Name of the Pull Request head repository.
*
*/
private String headRepository;
/**
* @return Head commit SHA of the Pull Request head.
*
*/
private String headSha;
/**
* @return List of label names set on the Pull Request.
*
*/
private List labels;
/**
* @return Indicates whether the base repository maintainers can modify the Pull Request.
*
*/
private Boolean maintainerCanModify;
/**
* @return The number of the Pull Request within the repository.
*
*/
private Integer number;
/**
* @return Unix timestamp indicating the Pull Request creation time.
*
*/
private Integer openedAt;
/**
* @return GitHub login of the user who opened the Pull Request.
*
*/
private String openedBy;
/**
* @return If set, filters Pull Requests by state. Can be "open", "closed", or "all". Default: "open".
*
*/
private String state;
/**
* @return The title of the Pull Request.
*
*/
private String title;
/**
* @return The timestamp of the last Pull Request update.
*
*/
private Integer updatedAt;
private GetRepositoryPullRequestsResult() {}
/**
* @return If set, filters Pull Requests by base branch name.
*
*/
public String baseRef() {
return this.baseRef;
}
/**
* @return Head commit SHA of the Pull Request base.
*
*/
public String baseSha() {
return this.baseSha;
}
/**
* @return Body of the Pull Request.
*
*/
public String body() {
return this.body;
}
/**
* @return Indicates Whether this Pull Request is a draft.
*
*/
public Boolean draft() {
return this.draft;
}
/**
* @return Owner of the Pull Request head repository.
*
*/
public String headOwner() {
return this.headOwner;
}
/**
* @return If set, filters Pull Requests by head user or head organization and branch name in the format of "user:ref-name" or "organization:ref-name". For example: "github:new-script-format" or "octocat:test-branch".
*
*/
public String headRef() {
return this.headRef;
}
/**
* @return Name of the Pull Request head repository.
*
*/
public String headRepository() {
return this.headRepository;
}
/**
* @return Head commit SHA of the Pull Request head.
*
*/
public String headSha() {
return this.headSha;
}
/**
* @return List of label names set on the Pull Request.
*
*/
public List labels() {
return this.labels;
}
/**
* @return Indicates whether the base repository maintainers can modify the Pull Request.
*
*/
public Boolean maintainerCanModify() {
return this.maintainerCanModify;
}
/**
* @return The number of the Pull Request within the repository.
*
*/
public Integer number() {
return this.number;
}
/**
* @return Unix timestamp indicating the Pull Request creation time.
*
*/
public Integer openedAt() {
return this.openedAt;
}
/**
* @return GitHub login of the user who opened the Pull Request.
*
*/
public String openedBy() {
return this.openedBy;
}
/**
* @return If set, filters Pull Requests by state. Can be "open", "closed", or "all". Default: "open".
*
*/
public String state() {
return this.state;
}
/**
* @return The title of the Pull Request.
*
*/
public String title() {
return this.title;
}
/**
* @return The timestamp of the last Pull Request update.
*
*/
public Integer updatedAt() {
return this.updatedAt;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRepositoryPullRequestsResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String baseRef;
private String baseSha;
private String body;
private Boolean draft;
private String headOwner;
private String headRef;
private String headRepository;
private String headSha;
private List labels;
private Boolean maintainerCanModify;
private Integer number;
private Integer openedAt;
private String openedBy;
private String state;
private String title;
private Integer updatedAt;
public Builder() {}
public Builder(GetRepositoryPullRequestsResult defaults) {
Objects.requireNonNull(defaults);
this.baseRef = defaults.baseRef;
this.baseSha = defaults.baseSha;
this.body = defaults.body;
this.draft = defaults.draft;
this.headOwner = defaults.headOwner;
this.headRef = defaults.headRef;
this.headRepository = defaults.headRepository;
this.headSha = defaults.headSha;
this.labels = defaults.labels;
this.maintainerCanModify = defaults.maintainerCanModify;
this.number = defaults.number;
this.openedAt = defaults.openedAt;
this.openedBy = defaults.openedBy;
this.state = defaults.state;
this.title = defaults.title;
this.updatedAt = defaults.updatedAt;
}
@CustomType.Setter
public Builder baseRef(String baseRef) {
if (baseRef == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "baseRef");
}
this.baseRef = baseRef;
return this;
}
@CustomType.Setter
public Builder baseSha(String baseSha) {
if (baseSha == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "baseSha");
}
this.baseSha = baseSha;
return this;
}
@CustomType.Setter
public Builder body(String body) {
if (body == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "body");
}
this.body = body;
return this;
}
@CustomType.Setter
public Builder draft(Boolean draft) {
if (draft == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "draft");
}
this.draft = draft;
return this;
}
@CustomType.Setter
public Builder headOwner(String headOwner) {
if (headOwner == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "headOwner");
}
this.headOwner = headOwner;
return this;
}
@CustomType.Setter
public Builder headRef(String headRef) {
if (headRef == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "headRef");
}
this.headRef = headRef;
return this;
}
@CustomType.Setter
public Builder headRepository(String headRepository) {
if (headRepository == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "headRepository");
}
this.headRepository = headRepository;
return this;
}
@CustomType.Setter
public Builder headSha(String headSha) {
if (headSha == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "headSha");
}
this.headSha = headSha;
return this;
}
@CustomType.Setter
public Builder labels(List labels) {
if (labels == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "labels");
}
this.labels = labels;
return this;
}
public Builder labels(String... labels) {
return labels(List.of(labels));
}
@CustomType.Setter
public Builder maintainerCanModify(Boolean maintainerCanModify) {
if (maintainerCanModify == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "maintainerCanModify");
}
this.maintainerCanModify = maintainerCanModify;
return this;
}
@CustomType.Setter
public Builder number(Integer number) {
if (number == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "number");
}
this.number = number;
return this;
}
@CustomType.Setter
public Builder openedAt(Integer openedAt) {
if (openedAt == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "openedAt");
}
this.openedAt = openedAt;
return this;
}
@CustomType.Setter
public Builder openedBy(String openedBy) {
if (openedBy == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "openedBy");
}
this.openedBy = openedBy;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder title(String title) {
if (title == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "title");
}
this.title = title;
return this;
}
@CustomType.Setter
public Builder updatedAt(Integer updatedAt) {
if (updatedAt == null) {
throw new MissingRequiredPropertyException("GetRepositoryPullRequestsResult", "updatedAt");
}
this.updatedAt = updatedAt;
return this;
}
public GetRepositoryPullRequestsResult build() {
final var _resultValue = new GetRepositoryPullRequestsResult();
_resultValue.baseRef = baseRef;
_resultValue.baseSha = baseSha;
_resultValue.body = body;
_resultValue.draft = draft;
_resultValue.headOwner = headOwner;
_resultValue.headRef = headRef;
_resultValue.headRepository = headRepository;
_resultValue.headSha = headSha;
_resultValue.labels = labels;
_resultValue.maintainerCanModify = maintainerCanModify;
_resultValue.number = number;
_resultValue.openedAt = openedAt;
_resultValue.openedBy = openedBy;
_resultValue.state = state;
_resultValue.title = title;
_resultValue.updatedAt = updatedAt;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy