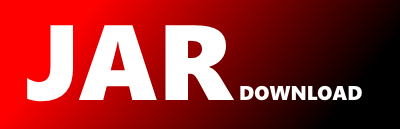
com.pulumi.gitlab.ComplianceFramework Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gitlab Show documentation
Show all versions of gitlab Show documentation
A Pulumi package for creating and managing GitLab resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.gitlab;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.gitlab.ComplianceFrameworkArgs;
import com.pulumi.gitlab.Utilities;
import com.pulumi.gitlab.inputs.ComplianceFrameworkState;
import java.lang.Boolean;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The `gitlab.ComplianceFramework` resource allows to manage the lifecycle of a compliance framework on top-level groups.
*
* There can be only one `default` compliance framework. Of all the configured compliance frameworks marked as default, the last one applied will be the default compliance framework.
*
* > This resource requires a GitLab Enterprise instance with a Premium license to create the compliance framework.
*
* > This resource requires a GitLab Enterprise instance with an Ultimate license to specify a compliance pipeline configuration in the compliance framework.
*
* **Upstream API**: [GitLab GraphQL API docs](https://docs.gitlab.com/ee/api/graphql/reference/#mutationcreatecomplianceframework)
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.ComplianceFramework;
* import com.pulumi.gitlab.ComplianceFrameworkArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var sample = new ComplianceFramework("sample", ComplianceFrameworkArgs.builder()
* .namespacePath("top-level-group")
* .name("HIPAA")
* .description("A HIPAA Compliance Framework")
* .color("#87BEEF")
* .default_(false)
* .pipelineConfigurationFullPath(".hipaa.yml}{@literal @}{@code top-level-group/compliance-frameworks")
* .build());
*
* }}{@code
* }}{@code
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Gitlab compliance frameworks can be imported with a key composed of `<namespace_path>:<framework_id>`, e.g.
*
* ```sh
* $ pulumi import gitlab:index/complianceFramework:ComplianceFramework sample "top-level-group:gid://gitlab/ComplianceManagement::Framework/12345"
* ```
*
*/
@ResourceType(type="gitlab:index/complianceFramework:ComplianceFramework")
public class ComplianceFramework extends com.pulumi.resources.CustomResource {
/**
* New color representation of the compliance framework in hex format. e.g. #FCA121.
*
*/
@Export(name="color", refs={String.class}, tree="[0]")
private Output color;
/**
* @return New color representation of the compliance framework in hex format. e.g. #FCA121.
*
*/
public Output color() {
return this.color;
}
/**
* Set this compliance framework as the default framework for the group. Default: `false`
*
*/
@Export(name="default", refs={Boolean.class}, tree="[0]")
private Output default_;
/**
* @return Set this compliance framework as the default framework for the group. Default: `false`
*
*/
public Output default_() {
return this.default_;
}
/**
* Description for the compliance framework.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return Description for the compliance framework.
*
*/
public Output description() {
return this.description;
}
/**
* Globally unique ID of the compliance framework.
*
*/
@Export(name="frameworkId", refs={String.class}, tree="[0]")
private Output frameworkId;
/**
* @return Globally unique ID of the compliance framework.
*
*/
public Output frameworkId() {
return this.frameworkId;
}
/**
* Name for the compliance framework.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name for the compliance framework.
*
*/
public Output name() {
return this.name;
}
/**
* Full path of the namespace to add the compliance framework to.
*
*/
@Export(name="namespacePath", refs={String.class}, tree="[0]")
private Output namespacePath;
/**
* @return Full path of the namespace to add the compliance framework to.
*
*/
public Output namespacePath() {
return this.namespacePath;
}
/**
* Full path of the compliance pipeline configuration stored in a project repository, such as `.gitlab/.compliance-gitlab-ci.yml{@literal @}compliance/hipaa`. Required format: `path/file.y[a]ml{@literal @}group-name/project-name` **Note**: Ultimate license required.
*
*/
@Export(name="pipelineConfigurationFullPath", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> pipelineConfigurationFullPath;
/**
* @return Full path of the compliance pipeline configuration stored in a project repository, such as `.gitlab/.compliance-gitlab-ci.yml{@literal @}compliance/hipaa`. Required format: `path/file.y[a]ml{@literal @}group-name/project-name` **Note**: Ultimate license required.
*
*/
public Output> pipelineConfigurationFullPath() {
return Codegen.optional(this.pipelineConfigurationFullPath);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ComplianceFramework(java.lang.String name) {
this(name, ComplianceFrameworkArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ComplianceFramework(java.lang.String name, ComplianceFrameworkArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ComplianceFramework(java.lang.String name, ComplianceFrameworkArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("gitlab:index/complianceFramework:ComplianceFramework", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ComplianceFramework(java.lang.String name, Output id, @Nullable ComplianceFrameworkState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("gitlab:index/complianceFramework:ComplianceFramework", name, state, makeResourceOptions(options, id), false);
}
private static ComplianceFrameworkArgs makeArgs(ComplianceFrameworkArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ComplianceFrameworkArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ComplianceFramework get(java.lang.String name, Output id, @Nullable ComplianceFrameworkState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ComplianceFramework(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy