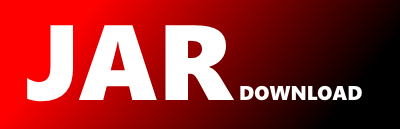
com.pulumi.gitlab.inputs.GetGroupProvisionedUsersProvisionedUserArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gitlab Show documentation
Show all versions of gitlab Show documentation
A Pulumi package for creating and managing GitLab resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.gitlab.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
public final class GetGroupProvisionedUsersProvisionedUserArgs extends com.pulumi.resources.ResourceArgs {
public static final GetGroupProvisionedUsersProvisionedUserArgs Empty = new GetGroupProvisionedUsersProvisionedUserArgs();
/**
* The avatar URL of the provisioned user.
*
*/
@Import(name="avatarUrl", required=true)
private Output avatarUrl;
/**
* @return The avatar URL of the provisioned user.
*
*/
public Output avatarUrl() {
return this.avatarUrl;
}
/**
* The bio of the provisioned user.
*
*/
@Import(name="bio", required=true)
private Output bio;
/**
* @return The bio of the provisioned user.
*
*/
public Output bio() {
return this.bio;
}
/**
* Whether the provisioned user is a bot.
*
*/
@Import(name="bot", required=true)
private Output bot;
/**
* @return Whether the provisioned user is a bot.
*
*/
public Output bot() {
return this.bot;
}
/**
* The confirmation date of the provisioned user.
*
*/
@Import(name="confirmedAt", required=true)
private Output confirmedAt;
/**
* @return The confirmation date of the provisioned user.
*
*/
public Output confirmedAt() {
return this.confirmedAt;
}
/**
* The creation date of the provisioned user.
*
*/
@Import(name="createdAt", required=true)
private Output createdAt;
/**
* @return The creation date of the provisioned user.
*
*/
public Output createdAt() {
return this.createdAt;
}
/**
* The email of the provisioned user.
*
*/
@Import(name="email", required=true)
private Output email;
/**
* @return The email of the provisioned user.
*
*/
public Output email() {
return this.email;
}
/**
* Whether the provisioned user is external.
*
*/
@Import(name="external", required=true)
private Output external;
/**
* @return Whether the provisioned user is external.
*
*/
public Output external() {
return this.external;
}
/**
* The ID of the provisioned user.
*
*/
@Import(name="id", required=true)
private Output id;
/**
* @return The ID of the provisioned user.
*
*/
public Output id() {
return this.id;
}
/**
* The job title of the provisioned user.
*
*/
@Import(name="jobTitle", required=true)
private Output jobTitle;
/**
* @return The job title of the provisioned user.
*
*/
public Output jobTitle() {
return this.jobTitle;
}
/**
* The last activity date of the provisioned user.
*
*/
@Import(name="lastActivityOn", required=true)
private Output lastActivityOn;
/**
* @return The last activity date of the provisioned user.
*
*/
public Output lastActivityOn() {
return this.lastActivityOn;
}
/**
* The last sign-in date of the provisioned user.
*
*/
@Import(name="lastSignInAt", required=true)
private Output lastSignInAt;
/**
* @return The last sign-in date of the provisioned user.
*
*/
public Output lastSignInAt() {
return this.lastSignInAt;
}
/**
* The LinkedIn ID of the provisioned user.
*
*/
@Import(name="linkedin", required=true)
private Output linkedin;
/**
* @return The LinkedIn ID of the provisioned user.
*
*/
public Output linkedin() {
return this.linkedin;
}
/**
* The location of the provisioned user.
*
*/
@Import(name="location", required=true)
private Output location;
/**
* @return The location of the provisioned user.
*
*/
public Output location() {
return this.location;
}
/**
* The name of the provisioned user.
*
*/
@Import(name="name", required=true)
private Output name;
/**
* @return The name of the provisioned user.
*
*/
public Output name() {
return this.name;
}
/**
* The organization of the provisioned user.
*
*/
@Import(name="organization", required=true)
private Output organization;
/**
* @return The organization of the provisioned user.
*
*/
public Output organization() {
return this.organization;
}
/**
* Whether the provisioned user has a private profile.
*
*/
@Import(name="privateProfile", required=true)
private Output privateProfile;
/**
* @return Whether the provisioned user has a private profile.
*
*/
public Output privateProfile() {
return this.privateProfile;
}
/**
* The pronouns of the provisioned user.
*
*/
@Import(name="pronouns", required=true)
private Output pronouns;
/**
* @return The pronouns of the provisioned user.
*
*/
public Output pronouns() {
return this.pronouns;
}
/**
* The public email of the provisioned user.
*
*/
@Import(name="publicEmail", required=true)
private Output publicEmail;
/**
* @return The public email of the provisioned user.
*
*/
public Output publicEmail() {
return this.publicEmail;
}
/**
* The Skype ID of the provisioned user.
*
*/
@Import(name="skype", required=true)
private Output skype;
/**
* @return The Skype ID of the provisioned user.
*
*/
public Output skype() {
return this.skype;
}
/**
* The state of the provisioned user.
*
*/
@Import(name="state", required=true)
private Output state;
/**
* @return The state of the provisioned user.
*
*/
public Output state() {
return this.state;
}
/**
* The Twitter ID of the provisioned user.
*
*/
@Import(name="twitter", required=true)
private Output twitter;
/**
* @return The Twitter ID of the provisioned user.
*
*/
public Output twitter() {
return this.twitter;
}
/**
* Whether two-factor authentication is enabled for the provisioned user.
*
*/
@Import(name="twoFactorEnabled", required=true)
private Output twoFactorEnabled;
/**
* @return Whether two-factor authentication is enabled for the provisioned user.
*
*/
public Output twoFactorEnabled() {
return this.twoFactorEnabled;
}
/**
* The username of the provisioned user.
*
*/
@Import(name="username", required=true)
private Output username;
/**
* @return The username of the provisioned user.
*
*/
public Output username() {
return this.username;
}
/**
* The web URL of the provisioned user.
*
*/
@Import(name="webUrl", required=true)
private Output webUrl;
/**
* @return The web URL of the provisioned user.
*
*/
public Output webUrl() {
return this.webUrl;
}
/**
* The website URL of the provisioned user.
*
*/
@Import(name="websiteUrl", required=true)
private Output websiteUrl;
/**
* @return The website URL of the provisioned user.
*
*/
public Output websiteUrl() {
return this.websiteUrl;
}
private GetGroupProvisionedUsersProvisionedUserArgs() {}
private GetGroupProvisionedUsersProvisionedUserArgs(GetGroupProvisionedUsersProvisionedUserArgs $) {
this.avatarUrl = $.avatarUrl;
this.bio = $.bio;
this.bot = $.bot;
this.confirmedAt = $.confirmedAt;
this.createdAt = $.createdAt;
this.email = $.email;
this.external = $.external;
this.id = $.id;
this.jobTitle = $.jobTitle;
this.lastActivityOn = $.lastActivityOn;
this.lastSignInAt = $.lastSignInAt;
this.linkedin = $.linkedin;
this.location = $.location;
this.name = $.name;
this.organization = $.organization;
this.privateProfile = $.privateProfile;
this.pronouns = $.pronouns;
this.publicEmail = $.publicEmail;
this.skype = $.skype;
this.state = $.state;
this.twitter = $.twitter;
this.twoFactorEnabled = $.twoFactorEnabled;
this.username = $.username;
this.webUrl = $.webUrl;
this.websiteUrl = $.websiteUrl;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetGroupProvisionedUsersProvisionedUserArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetGroupProvisionedUsersProvisionedUserArgs $;
public Builder() {
$ = new GetGroupProvisionedUsersProvisionedUserArgs();
}
public Builder(GetGroupProvisionedUsersProvisionedUserArgs defaults) {
$ = new GetGroupProvisionedUsersProvisionedUserArgs(Objects.requireNonNull(defaults));
}
/**
* @param avatarUrl The avatar URL of the provisioned user.
*
* @return builder
*
*/
public Builder avatarUrl(Output avatarUrl) {
$.avatarUrl = avatarUrl;
return this;
}
/**
* @param avatarUrl The avatar URL of the provisioned user.
*
* @return builder
*
*/
public Builder avatarUrl(String avatarUrl) {
return avatarUrl(Output.of(avatarUrl));
}
/**
* @param bio The bio of the provisioned user.
*
* @return builder
*
*/
public Builder bio(Output bio) {
$.bio = bio;
return this;
}
/**
* @param bio The bio of the provisioned user.
*
* @return builder
*
*/
public Builder bio(String bio) {
return bio(Output.of(bio));
}
/**
* @param bot Whether the provisioned user is a bot.
*
* @return builder
*
*/
public Builder bot(Output bot) {
$.bot = bot;
return this;
}
/**
* @param bot Whether the provisioned user is a bot.
*
* @return builder
*
*/
public Builder bot(Boolean bot) {
return bot(Output.of(bot));
}
/**
* @param confirmedAt The confirmation date of the provisioned user.
*
* @return builder
*
*/
public Builder confirmedAt(Output confirmedAt) {
$.confirmedAt = confirmedAt;
return this;
}
/**
* @param confirmedAt The confirmation date of the provisioned user.
*
* @return builder
*
*/
public Builder confirmedAt(String confirmedAt) {
return confirmedAt(Output.of(confirmedAt));
}
/**
* @param createdAt The creation date of the provisioned user.
*
* @return builder
*
*/
public Builder createdAt(Output createdAt) {
$.createdAt = createdAt;
return this;
}
/**
* @param createdAt The creation date of the provisioned user.
*
* @return builder
*
*/
public Builder createdAt(String createdAt) {
return createdAt(Output.of(createdAt));
}
/**
* @param email The email of the provisioned user.
*
* @return builder
*
*/
public Builder email(Output email) {
$.email = email;
return this;
}
/**
* @param email The email of the provisioned user.
*
* @return builder
*
*/
public Builder email(String email) {
return email(Output.of(email));
}
/**
* @param external Whether the provisioned user is external.
*
* @return builder
*
*/
public Builder external(Output external) {
$.external = external;
return this;
}
/**
* @param external Whether the provisioned user is external.
*
* @return builder
*
*/
public Builder external(Boolean external) {
return external(Output.of(external));
}
/**
* @param id The ID of the provisioned user.
*
* @return builder
*
*/
public Builder id(Output id) {
$.id = id;
return this;
}
/**
* @param id The ID of the provisioned user.
*
* @return builder
*
*/
public Builder id(String id) {
return id(Output.of(id));
}
/**
* @param jobTitle The job title of the provisioned user.
*
* @return builder
*
*/
public Builder jobTitle(Output jobTitle) {
$.jobTitle = jobTitle;
return this;
}
/**
* @param jobTitle The job title of the provisioned user.
*
* @return builder
*
*/
public Builder jobTitle(String jobTitle) {
return jobTitle(Output.of(jobTitle));
}
/**
* @param lastActivityOn The last activity date of the provisioned user.
*
* @return builder
*
*/
public Builder lastActivityOn(Output lastActivityOn) {
$.lastActivityOn = lastActivityOn;
return this;
}
/**
* @param lastActivityOn The last activity date of the provisioned user.
*
* @return builder
*
*/
public Builder lastActivityOn(String lastActivityOn) {
return lastActivityOn(Output.of(lastActivityOn));
}
/**
* @param lastSignInAt The last sign-in date of the provisioned user.
*
* @return builder
*
*/
public Builder lastSignInAt(Output lastSignInAt) {
$.lastSignInAt = lastSignInAt;
return this;
}
/**
* @param lastSignInAt The last sign-in date of the provisioned user.
*
* @return builder
*
*/
public Builder lastSignInAt(String lastSignInAt) {
return lastSignInAt(Output.of(lastSignInAt));
}
/**
* @param linkedin The LinkedIn ID of the provisioned user.
*
* @return builder
*
*/
public Builder linkedin(Output linkedin) {
$.linkedin = linkedin;
return this;
}
/**
* @param linkedin The LinkedIn ID of the provisioned user.
*
* @return builder
*
*/
public Builder linkedin(String linkedin) {
return linkedin(Output.of(linkedin));
}
/**
* @param location The location of the provisioned user.
*
* @return builder
*
*/
public Builder location(Output location) {
$.location = location;
return this;
}
/**
* @param location The location of the provisioned user.
*
* @return builder
*
*/
public Builder location(String location) {
return location(Output.of(location));
}
/**
* @param name The name of the provisioned user.
*
* @return builder
*
*/
public Builder name(Output name) {
$.name = name;
return this;
}
/**
* @param name The name of the provisioned user.
*
* @return builder
*
*/
public Builder name(String name) {
return name(Output.of(name));
}
/**
* @param organization The organization of the provisioned user.
*
* @return builder
*
*/
public Builder organization(Output organization) {
$.organization = organization;
return this;
}
/**
* @param organization The organization of the provisioned user.
*
* @return builder
*
*/
public Builder organization(String organization) {
return organization(Output.of(organization));
}
/**
* @param privateProfile Whether the provisioned user has a private profile.
*
* @return builder
*
*/
public Builder privateProfile(Output privateProfile) {
$.privateProfile = privateProfile;
return this;
}
/**
* @param privateProfile Whether the provisioned user has a private profile.
*
* @return builder
*
*/
public Builder privateProfile(Boolean privateProfile) {
return privateProfile(Output.of(privateProfile));
}
/**
* @param pronouns The pronouns of the provisioned user.
*
* @return builder
*
*/
public Builder pronouns(Output pronouns) {
$.pronouns = pronouns;
return this;
}
/**
* @param pronouns The pronouns of the provisioned user.
*
* @return builder
*
*/
public Builder pronouns(String pronouns) {
return pronouns(Output.of(pronouns));
}
/**
* @param publicEmail The public email of the provisioned user.
*
* @return builder
*
*/
public Builder publicEmail(Output publicEmail) {
$.publicEmail = publicEmail;
return this;
}
/**
* @param publicEmail The public email of the provisioned user.
*
* @return builder
*
*/
public Builder publicEmail(String publicEmail) {
return publicEmail(Output.of(publicEmail));
}
/**
* @param skype The Skype ID of the provisioned user.
*
* @return builder
*
*/
public Builder skype(Output skype) {
$.skype = skype;
return this;
}
/**
* @param skype The Skype ID of the provisioned user.
*
* @return builder
*
*/
public Builder skype(String skype) {
return skype(Output.of(skype));
}
/**
* @param state The state of the provisioned user.
*
* @return builder
*
*/
public Builder state(Output state) {
$.state = state;
return this;
}
/**
* @param state The state of the provisioned user.
*
* @return builder
*
*/
public Builder state(String state) {
return state(Output.of(state));
}
/**
* @param twitter The Twitter ID of the provisioned user.
*
* @return builder
*
*/
public Builder twitter(Output twitter) {
$.twitter = twitter;
return this;
}
/**
* @param twitter The Twitter ID of the provisioned user.
*
* @return builder
*
*/
public Builder twitter(String twitter) {
return twitter(Output.of(twitter));
}
/**
* @param twoFactorEnabled Whether two-factor authentication is enabled for the provisioned user.
*
* @return builder
*
*/
public Builder twoFactorEnabled(Output twoFactorEnabled) {
$.twoFactorEnabled = twoFactorEnabled;
return this;
}
/**
* @param twoFactorEnabled Whether two-factor authentication is enabled for the provisioned user.
*
* @return builder
*
*/
public Builder twoFactorEnabled(Boolean twoFactorEnabled) {
return twoFactorEnabled(Output.of(twoFactorEnabled));
}
/**
* @param username The username of the provisioned user.
*
* @return builder
*
*/
public Builder username(Output username) {
$.username = username;
return this;
}
/**
* @param username The username of the provisioned user.
*
* @return builder
*
*/
public Builder username(String username) {
return username(Output.of(username));
}
/**
* @param webUrl The web URL of the provisioned user.
*
* @return builder
*
*/
public Builder webUrl(Output webUrl) {
$.webUrl = webUrl;
return this;
}
/**
* @param webUrl The web URL of the provisioned user.
*
* @return builder
*
*/
public Builder webUrl(String webUrl) {
return webUrl(Output.of(webUrl));
}
/**
* @param websiteUrl The website URL of the provisioned user.
*
* @return builder
*
*/
public Builder websiteUrl(Output websiteUrl) {
$.websiteUrl = websiteUrl;
return this;
}
/**
* @param websiteUrl The website URL of the provisioned user.
*
* @return builder
*
*/
public Builder websiteUrl(String websiteUrl) {
return websiteUrl(Output.of(websiteUrl));
}
public GetGroupProvisionedUsersProvisionedUserArgs build() {
if ($.avatarUrl == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "avatarUrl");
}
if ($.bio == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "bio");
}
if ($.bot == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "bot");
}
if ($.confirmedAt == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "confirmedAt");
}
if ($.createdAt == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "createdAt");
}
if ($.email == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "email");
}
if ($.external == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "external");
}
if ($.id == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "id");
}
if ($.jobTitle == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "jobTitle");
}
if ($.lastActivityOn == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "lastActivityOn");
}
if ($.lastSignInAt == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "lastSignInAt");
}
if ($.linkedin == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "linkedin");
}
if ($.location == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "location");
}
if ($.name == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "name");
}
if ($.organization == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "organization");
}
if ($.privateProfile == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "privateProfile");
}
if ($.pronouns == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "pronouns");
}
if ($.publicEmail == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "publicEmail");
}
if ($.skype == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "skype");
}
if ($.state == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "state");
}
if ($.twitter == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "twitter");
}
if ($.twoFactorEnabled == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "twoFactorEnabled");
}
if ($.username == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "username");
}
if ($.webUrl == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "webUrl");
}
if ($.websiteUrl == null) {
throw new MissingRequiredPropertyException("GetGroupProvisionedUsersProvisionedUserArgs", "websiteUrl");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy