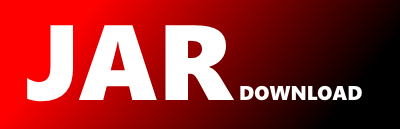
com.pulumi.googlenative.apigee.v1.HostSecurityReport Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigee.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.apigee.v1.HostSecurityReportArgs;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1SecurityReportMetadataResponse;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1SecurityReportResultMetadataResponse;
import java.lang.String;
import javax.annotation.Nullable;
/**
* Submit a query at host level to be processed in the background. If the submission of the query succeeds, the API returns a 201 status and an ID that refer to the query. In addition to the HTTP status 201, the `state` of "enqueued" means that the request succeeded.
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
*
*/
@ResourceType(type="google-native:apigee/v1:HostSecurityReport")
public class HostSecurityReport extends com.pulumi.resources.CustomResource {
/**
* Creation time of the query.
*
*/
@Export(name="created", type=String.class, parameters={})
private Output created;
/**
* @return Creation time of the query.
*
*/
public Output created() {
return this.created;
}
/**
* Display Name specified by the user.
*
*/
@Export(name="displayName", type=String.class, parameters={})
private Output displayName;
/**
* @return Display Name specified by the user.
*
*/
public Output displayName() {
return this.displayName;
}
/**
* Hostname is available only when query is executed at host level.
*
*/
@Export(name="envgroupHostname", type=String.class, parameters={})
private Output envgroupHostname;
/**
* @return Hostname is available only when query is executed at host level.
*
*/
public Output envgroupHostname() {
return this.envgroupHostname;
}
/**
* Error is set when query fails.
*
*/
@Export(name="error", type=String.class, parameters={})
private Output error;
/**
* @return Error is set when query fails.
*
*/
public Output error() {
return this.error;
}
/**
* ExecutionTime is available only after the query is completed.
*
*/
@Export(name="executionTime", type=String.class, parameters={})
private Output executionTime;
/**
* @return ExecutionTime is available only after the query is completed.
*
*/
public Output executionTime() {
return this.executionTime;
}
@Export(name="organizationId", type=String.class, parameters={})
private Output organizationId;
public Output organizationId() {
return this.organizationId;
}
/**
* Contains information like metrics, dimenstions etc of the Security Report.
*
*/
@Export(name="queryParams", type=GoogleCloudApigeeV1SecurityReportMetadataResponse.class, parameters={})
private Output queryParams;
/**
* @return Contains information like metrics, dimenstions etc of the Security Report.
*
*/
public Output queryParams() {
return this.queryParams;
}
/**
* Report Definition ID.
*
*/
@Export(name="reportDefinitionId", type=String.class, parameters={})
private Output reportDefinitionId;
/**
* @return Report Definition ID.
*
*/
public Output reportDefinitionId() {
return this.reportDefinitionId;
}
/**
* Result is available only after the query is completed.
*
*/
@Export(name="result", type=GoogleCloudApigeeV1SecurityReportResultMetadataResponse.class, parameters={})
private Output result;
/**
* @return Result is available only after the query is completed.
*
*/
public Output result() {
return this.result;
}
/**
* ResultFileSize is available only after the query is completed.
*
*/
@Export(name="resultFileSize", type=String.class, parameters={})
private Output resultFileSize;
/**
* @return ResultFileSize is available only after the query is completed.
*
*/
public Output resultFileSize() {
return this.resultFileSize;
}
/**
* ResultRows is available only after the query is completed.
*
*/
@Export(name="resultRows", type=String.class, parameters={})
private Output resultRows;
/**
* @return ResultRows is available only after the query is completed.
*
*/
public Output resultRows() {
return this.resultRows;
}
/**
* Self link of the query. Example: `/organizations/myorg/environments/myenv/securityReports/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd` or following format if query is running at host level: `/organizations/myorg/hostSecurityReports/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd`
*
*/
@Export(name="self", type=String.class, parameters={})
private Output self;
/**
* @return Self link of the query. Example: `/organizations/myorg/environments/myenv/securityReports/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd` or following format if query is running at host level: `/organizations/myorg/hostSecurityReports/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd`
*
*/
public Output self() {
return this.self;
}
/**
* Query state could be "enqueued", "running", "completed", "expired" and "failed".
*
*/
@Export(name="state", type=String.class, parameters={})
private Output state;
/**
* @return Query state could be "enqueued", "running", "completed", "expired" and "failed".
*
*/
public Output state() {
return this.state;
}
/**
* Last updated timestamp for the query.
*
*/
@Export(name="updated", type=String.class, parameters={})
private Output updated;
/**
* @return Last updated timestamp for the query.
*
*/
public Output updated() {
return this.updated;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public HostSecurityReport(String name) {
this(name, HostSecurityReportArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public HostSecurityReport(String name, HostSecurityReportArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public HostSecurityReport(String name, HostSecurityReportArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:apigee/v1:HostSecurityReport", name, args == null ? HostSecurityReportArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private HostSecurityReport(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:apigee/v1:HostSecurityReport", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static HostSecurityReport get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new HostSecurityReport(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy