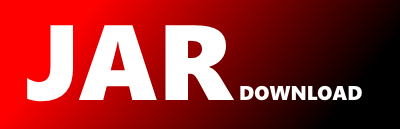
com.pulumi.googlenative.apigee.v1.InstanceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigee.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.apigee.v1.enums.InstancePeeringCidrRange;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InstanceArgs extends com.pulumi.resources.ResourceArgs {
public static final InstanceArgs Empty = new InstanceArgs();
/**
* Optional. Customer accept list represents the list of projects (id/number) on customer side that can privately connect to the service attachment. It is an optional field which the customers can provide during the instance creation. By default, the customer project associated with the Apigee organization will be included to the list.
*
*/
@Import(name="consumerAcceptList")
private @Nullable Output> consumerAcceptList;
/**
* @return Optional. Customer accept list represents the list of projects (id/number) on customer side that can privately connect to the service attachment. It is an optional field which the customers can provide during the instance creation. By default, the customer project associated with the Apigee organization will be included to the list.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy