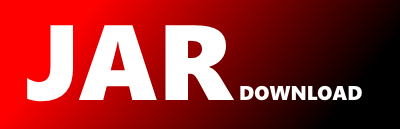
com.pulumi.googlenative.apigee.v1.RatePlan Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigee.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.apigee.v1.RatePlanArgs;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1RateRangeResponse;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1RevenueShareRangeResponse;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleTypeMoneyResponse;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* Create a rate plan that is associated with an API product in an organization. Using rate plans, API product owners can monetize their API products by configuring one or more of the following: - Billing frequency - Initial setup fees for using an API product - Payment funding model (postpaid only) - Fixed recurring or consumption-based charges for using an API product - Revenue sharing with developer partners An API product can have multiple rate plans associated with it but *only one* rate plan can be active at any point of time. **Note: From the developer's perspective, they purchase API products not rate plans.
* Auto-naming is currently not supported for this resource.
*
*/
@ResourceType(type="google-native:apigee/v1:RatePlan")
public class RatePlan extends com.pulumi.resources.CustomResource {
/**
* Name of the API product that the rate plan is associated with.
*
*/
@Export(name="apiproduct", type=String.class, parameters={})
private Output apiproduct;
/**
* @return Name of the API product that the rate plan is associated with.
*
*/
public Output apiproduct() {
return this.apiproduct;
}
@Export(name="apiproductId", type=String.class, parameters={})
private Output apiproductId;
public Output apiproductId() {
return this.apiproductId;
}
/**
* Frequency at which the customer will be billed.
*
*/
@Export(name="billingPeriod", type=String.class, parameters={})
private Output billingPeriod;
/**
* @return Frequency at which the customer will be billed.
*
*/
public Output billingPeriod() {
return this.billingPeriod;
}
/**
* API call volume ranges and the fees charged when the total number of API calls is within a given range. The method used to calculate the final fee depends on the selected pricing model. For example, if the pricing model is `STAIRSTEP` and the ranges are defined as follows: ```{ "start": 1, "end": 100, "fee": 75 }, { "start": 101, "end": 200, "fee": 100 }, }``` Then the following fees would be charged based on the total number of API calls (assuming the currency selected is `USD`): * 1 call costs $75 * 50 calls cost $75 * 150 calls cost $100 The number of API calls cannot exceed 200.
*
*/
@Export(name="consumptionPricingRates", type=List.class, parameters={GoogleCloudApigeeV1RateRangeResponse.class})
private Output> consumptionPricingRates;
/**
* @return API call volume ranges and the fees charged when the total number of API calls is within a given range. The method used to calculate the final fee depends on the selected pricing model. For example, if the pricing model is `STAIRSTEP` and the ranges are defined as follows: ```{ "start": 1, "end": 100, "fee": 75 }, { "start": 101, "end": 200, "fee": 100 }, }``` Then the following fees would be charged based on the total number of API calls (assuming the currency selected is `USD`): * 1 call costs $75 * 50 calls cost $75 * 150 calls cost $100 The number of API calls cannot exceed 200.
*
*/
public Output> consumptionPricingRates() {
return this.consumptionPricingRates;
}
/**
* Pricing model used for consumption-based charges.
*
*/
@Export(name="consumptionPricingType", type=String.class, parameters={})
private Output consumptionPricingType;
/**
* @return Pricing model used for consumption-based charges.
*
*/
public Output consumptionPricingType() {
return this.consumptionPricingType;
}
/**
* Time that the rate plan was created in milliseconds since epoch.
*
*/
@Export(name="createdAt", type=String.class, parameters={})
private Output createdAt;
/**
* @return Time that the rate plan was created in milliseconds since epoch.
*
*/
public Output createdAt() {
return this.createdAt;
}
/**
* Currency to be used for billing. Consists of a three-letter code as defined by the [ISO 4217](https://en.wikipedia.org/wiki/ISO_4217) standard.
*
*/
@Export(name="currencyCode", type=String.class, parameters={})
private Output currencyCode;
/**
* @return Currency to be used for billing. Consists of a three-letter code as defined by the [ISO 4217](https://en.wikipedia.org/wiki/ISO_4217) standard.
*
*/
public Output currencyCode() {
return this.currencyCode;
}
/**
* Description of the rate plan.
*
*/
@Export(name="description", type=String.class, parameters={})
private Output description;
/**
* @return Description of the rate plan.
*
*/
public Output description() {
return this.description;
}
/**
* Display name of the rate plan.
*
*/
@Export(name="displayName", type=String.class, parameters={})
private Output displayName;
/**
* @return Display name of the rate plan.
*
*/
public Output displayName() {
return this.displayName;
}
/**
* Time when the rate plan will expire in milliseconds since epoch. Set to 0 or `null` to indicate that the rate plan should never expire.
*
*/
@Export(name="endTime", type=String.class, parameters={})
private Output endTime;
/**
* @return Time when the rate plan will expire in milliseconds since epoch. Set to 0 or `null` to indicate that the rate plan should never expire.
*
*/
public Output endTime() {
return this.endTime;
}
/**
* Frequency at which the fixed fee is charged.
*
*/
@Export(name="fixedFeeFrequency", type=Integer.class, parameters={})
private Output fixedFeeFrequency;
/**
* @return Frequency at which the fixed fee is charged.
*
*/
public Output fixedFeeFrequency() {
return this.fixedFeeFrequency;
}
/**
* Fixed amount that is charged at a defined interval and billed in advance of use of the API product. The fee will be prorated for the first billing period.
*
*/
@Export(name="fixedRecurringFee", type=GoogleTypeMoneyResponse.class, parameters={})
private Output fixedRecurringFee;
/**
* @return Fixed amount that is charged at a defined interval and billed in advance of use of the API product. The fee will be prorated for the first billing period.
*
*/
public Output fixedRecurringFee() {
return this.fixedRecurringFee;
}
/**
* Time the rate plan was last modified in milliseconds since epoch.
*
*/
@Export(name="lastModifiedAt", type=String.class, parameters={})
private Output lastModifiedAt;
/**
* @return Time the rate plan was last modified in milliseconds since epoch.
*
*/
public Output lastModifiedAt() {
return this.lastModifiedAt;
}
/**
* Name of the rate plan.
*
*/
@Export(name="name", type=String.class, parameters={})
private Output name;
/**
* @return Name of the rate plan.
*
*/
public Output name() {
return this.name;
}
@Export(name="organizationId", type=String.class, parameters={})
private Output organizationId;
public Output organizationId() {
return this.organizationId;
}
/**
* DEPRECATED: This field is no longer supported and will eventually be removed when Apigee Hybrid 1.5/1.6 is no longer supported. Instead, use the `billingType` field inside `DeveloperMonetizationConfig` resource. Flag that specifies the billing account type, prepaid or postpaid.
*
* @deprecated
* DEPRECATED: This field is no longer supported and will eventually be removed when Apigee Hybrid 1.5/1.6 is no longer supported. Instead, use the `billingType` field inside `DeveloperMonetizationConfig` resource. Flag that specifies the billing account type, prepaid or postpaid.
*
*/
@Deprecated /* DEPRECATED: This field is no longer supported and will eventually be removed when Apigee Hybrid 1.5/1.6 is no longer supported. Instead, use the `billingType` field inside `DeveloperMonetizationConfig` resource. Flag that specifies the billing account type, prepaid or postpaid. */
@Export(name="paymentFundingModel", type=String.class, parameters={})
private Output paymentFundingModel;
/**
* @return DEPRECATED: This field is no longer supported and will eventually be removed when Apigee Hybrid 1.5/1.6 is no longer supported. Instead, use the `billingType` field inside `DeveloperMonetizationConfig` resource. Flag that specifies the billing account type, prepaid or postpaid.
*
*/
public Output paymentFundingModel() {
return this.paymentFundingModel;
}
/**
* Details of the revenue sharing model.
*
*/
@Export(name="revenueShareRates", type=List.class, parameters={GoogleCloudApigeeV1RevenueShareRangeResponse.class})
private Output> revenueShareRates;
/**
* @return Details of the revenue sharing model.
*
*/
public Output> revenueShareRates() {
return this.revenueShareRates;
}
/**
* Method used to calculate the revenue that is shared with developers.
*
*/
@Export(name="revenueShareType", type=String.class, parameters={})
private Output revenueShareType;
/**
* @return Method used to calculate the revenue that is shared with developers.
*
*/
public Output revenueShareType() {
return this.revenueShareType;
}
/**
* Initial, one-time fee paid when purchasing the API product.
*
*/
@Export(name="setupFee", type=GoogleTypeMoneyResponse.class, parameters={})
private Output setupFee;
/**
* @return Initial, one-time fee paid when purchasing the API product.
*
*/
public Output setupFee() {
return this.setupFee;
}
/**
* Time when the rate plan becomes active in milliseconds since epoch.
*
*/
@Export(name="startTime", type=String.class, parameters={})
private Output startTime;
/**
* @return Time when the rate plan becomes active in milliseconds since epoch.
*
*/
public Output startTime() {
return this.startTime;
}
/**
* Current state of the rate plan (draft or published).
*
*/
@Export(name="state", type=String.class, parameters={})
private Output state;
/**
* @return Current state of the rate plan (draft or published).
*
*/
public Output state() {
return this.state;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public RatePlan(String name) {
this(name, RatePlanArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public RatePlan(String name, RatePlanArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public RatePlan(String name, RatePlanArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:apigee/v1:RatePlan", name, args == null ? RatePlanArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private RatePlan(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:apigee/v1:RatePlan", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static RatePlan get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new RatePlan(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy