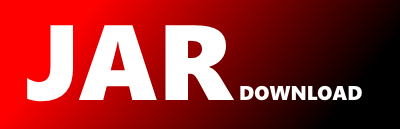
com.pulumi.googlenative.apigee.v1.outputs.GetKeyResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigee.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1AttributeResponse;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetKeyResult {
/**
* @return List of API products for which the credential can be used. **Note**: Do not specify the list of API products when creating a consumer key and secret for a developer app. Instead, use the UpdateDeveloperAppKey API to make the association after the consumer key and secret are created.
*
*/
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy