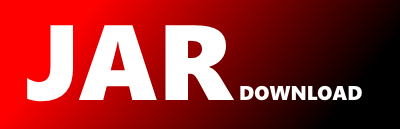
com.pulumi.googlenative.apigee.v1.outputs.GetQueryResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigee.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1AsyncQueryResultResponse;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1QueryMetadataResponse;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetQueryResult {
/**
* @return Creation time of the query.
*
*/
private String created;
/**
* @return Hostname is available only when query is executed at host level.
*
*/
private String envgroupHostname;
/**
* @return Error is set when query fails.
*
*/
private String error;
/**
* @return ExecutionTime is available only after the query is completed.
*
*/
private String executionTime;
/**
* @return Asynchronous Query Name.
*
*/
private String name;
/**
* @return Contains information like metrics, dimenstions etc of the AsyncQuery.
*
*/
private GoogleCloudApigeeV1QueryMetadataResponse queryParams;
/**
* @return Asynchronous Report ID.
*
*/
private String reportDefinitionId;
/**
* @return Result is available only after the query is completed.
*
*/
private GoogleCloudApigeeV1AsyncQueryResultResponse result;
/**
* @return ResultFileSize is available only after the query is completed.
*
*/
private String resultFileSize;
/**
* @return ResultRows is available only after the query is completed.
*
*/
private String resultRows;
/**
* @return Self link of the query. Example: `/organizations/myorg/environments/myenv/queries/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd` or following format if query is running at host level: `/organizations/myorg/hostQueries/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd`
*
*/
private String self;
/**
* @return Query state could be "enqueued", "running", "completed", "failed".
*
*/
private String state;
/**
* @return Last updated timestamp for the query.
*
*/
private String updated;
private GetQueryResult() {}
/**
* @return Creation time of the query.
*
*/
public String created() {
return this.created;
}
/**
* @return Hostname is available only when query is executed at host level.
*
*/
public String envgroupHostname() {
return this.envgroupHostname;
}
/**
* @return Error is set when query fails.
*
*/
public String error() {
return this.error;
}
/**
* @return ExecutionTime is available only after the query is completed.
*
*/
public String executionTime() {
return this.executionTime;
}
/**
* @return Asynchronous Query Name.
*
*/
public String name() {
return this.name;
}
/**
* @return Contains information like metrics, dimenstions etc of the AsyncQuery.
*
*/
public GoogleCloudApigeeV1QueryMetadataResponse queryParams() {
return this.queryParams;
}
/**
* @return Asynchronous Report ID.
*
*/
public String reportDefinitionId() {
return this.reportDefinitionId;
}
/**
* @return Result is available only after the query is completed.
*
*/
public GoogleCloudApigeeV1AsyncQueryResultResponse result() {
return this.result;
}
/**
* @return ResultFileSize is available only after the query is completed.
*
*/
public String resultFileSize() {
return this.resultFileSize;
}
/**
* @return ResultRows is available only after the query is completed.
*
*/
public String resultRows() {
return this.resultRows;
}
/**
* @return Self link of the query. Example: `/organizations/myorg/environments/myenv/queries/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd` or following format if query is running at host level: `/organizations/myorg/hostQueries/9cfc0d85-0f30-46d6-ae6f-318d0cb961bd`
*
*/
public String self() {
return this.self;
}
/**
* @return Query state could be "enqueued", "running", "completed", "failed".
*
*/
public String state() {
return this.state;
}
/**
* @return Last updated timestamp for the query.
*
*/
public String updated() {
return this.updated;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetQueryResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String created;
private String envgroupHostname;
private String error;
private String executionTime;
private String name;
private GoogleCloudApigeeV1QueryMetadataResponse queryParams;
private String reportDefinitionId;
private GoogleCloudApigeeV1AsyncQueryResultResponse result;
private String resultFileSize;
private String resultRows;
private String self;
private String state;
private String updated;
public Builder() {}
public Builder(GetQueryResult defaults) {
Objects.requireNonNull(defaults);
this.created = defaults.created;
this.envgroupHostname = defaults.envgroupHostname;
this.error = defaults.error;
this.executionTime = defaults.executionTime;
this.name = defaults.name;
this.queryParams = defaults.queryParams;
this.reportDefinitionId = defaults.reportDefinitionId;
this.result = defaults.result;
this.resultFileSize = defaults.resultFileSize;
this.resultRows = defaults.resultRows;
this.self = defaults.self;
this.state = defaults.state;
this.updated = defaults.updated;
}
@CustomType.Setter
public Builder created(String created) {
this.created = Objects.requireNonNull(created);
return this;
}
@CustomType.Setter
public Builder envgroupHostname(String envgroupHostname) {
this.envgroupHostname = Objects.requireNonNull(envgroupHostname);
return this;
}
@CustomType.Setter
public Builder error(String error) {
this.error = Objects.requireNonNull(error);
return this;
}
@CustomType.Setter
public Builder executionTime(String executionTime) {
this.executionTime = Objects.requireNonNull(executionTime);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder queryParams(GoogleCloudApigeeV1QueryMetadataResponse queryParams) {
this.queryParams = Objects.requireNonNull(queryParams);
return this;
}
@CustomType.Setter
public Builder reportDefinitionId(String reportDefinitionId) {
this.reportDefinitionId = Objects.requireNonNull(reportDefinitionId);
return this;
}
@CustomType.Setter
public Builder result(GoogleCloudApigeeV1AsyncQueryResultResponse result) {
this.result = Objects.requireNonNull(result);
return this;
}
@CustomType.Setter
public Builder resultFileSize(String resultFileSize) {
this.resultFileSize = Objects.requireNonNull(resultFileSize);
return this;
}
@CustomType.Setter
public Builder resultRows(String resultRows) {
this.resultRows = Objects.requireNonNull(resultRows);
return this;
}
@CustomType.Setter
public Builder self(String self) {
this.self = Objects.requireNonNull(self);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder updated(String updated) {
this.updated = Objects.requireNonNull(updated);
return this;
}
public GetQueryResult build() {
final var o = new GetQueryResult();
o.created = created;
o.envgroupHostname = envgroupHostname;
o.error = error;
o.executionTime = executionTime;
o.name = name;
o.queryParams = queryParams;
o.reportDefinitionId = reportDefinitionId;
o.result = result;
o.resultFileSize = resultFileSize;
o.resultRows = resultRows;
o.self = self;
o.state = state;
o.updated = updated;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy