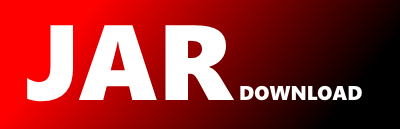
com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1TlsInfoResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigee.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.apigee.v1.outputs.GoogleCloudApigeeV1TlsInfoCommonNameResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GoogleCloudApigeeV1TlsInfoResponse {
/**
* @return The SSL/TLS cipher suites to be used. For programmable proxies, it must be one of the cipher suite names listed in: http://docs.oracle.com/javase/8/docs/technotes/guides/security/StandardNames.html#ciphersuites. For configurable proxies, it must follow the configuration specified in: https://commondatastorage.googleapis.com/chromium-boringssl-docs/ssl.h.html#Cipher-suite-configuration. This setting has no effect for configurable proxies when negotiating TLS 1.3.
*
*/
private List ciphers;
/**
* @return Optional. Enables two-way TLS.
*
*/
private Boolean clientAuthEnabled;
/**
* @return The TLS Common Name of the certificate.
*
*/
private GoogleCloudApigeeV1TlsInfoCommonNameResponse commonName;
/**
* @return Enables TLS. If false, neither one-way nor two-way TLS will be enabled.
*
*/
private Boolean enabled;
/**
* @return If true, Edge ignores TLS certificate errors. Valid when configuring TLS for target servers and target endpoints, and when configuring virtual hosts that use 2-way TLS. When used with a target endpoint/target server, if the backend system uses SNI and returns a cert with a subject Distinguished Name (DN) that does not match the hostname, there is no way to ignore the error and the connection fails.
*
*/
private Boolean ignoreValidationErrors;
/**
* @return Required if `client_auth_enabled` is true. The resource ID for the alias containing the private key and cert.
*
*/
private String keyAlias;
/**
* @return Required if `client_auth_enabled` is true. The resource ID of the keystore.
*
*/
private String keyStore;
/**
* @return The TLS versioins to be used.
*
*/
private List protocols;
/**
* @return The resource ID of the truststore.
*
*/
private String trustStore;
private GoogleCloudApigeeV1TlsInfoResponse() {}
/**
* @return The SSL/TLS cipher suites to be used. For programmable proxies, it must be one of the cipher suite names listed in: http://docs.oracle.com/javase/8/docs/technotes/guides/security/StandardNames.html#ciphersuites. For configurable proxies, it must follow the configuration specified in: https://commondatastorage.googleapis.com/chromium-boringssl-docs/ssl.h.html#Cipher-suite-configuration. This setting has no effect for configurable proxies when negotiating TLS 1.3.
*
*/
public List ciphers() {
return this.ciphers;
}
/**
* @return Optional. Enables two-way TLS.
*
*/
public Boolean clientAuthEnabled() {
return this.clientAuthEnabled;
}
/**
* @return The TLS Common Name of the certificate.
*
*/
public GoogleCloudApigeeV1TlsInfoCommonNameResponse commonName() {
return this.commonName;
}
/**
* @return Enables TLS. If false, neither one-way nor two-way TLS will be enabled.
*
*/
public Boolean enabled() {
return this.enabled;
}
/**
* @return If true, Edge ignores TLS certificate errors. Valid when configuring TLS for target servers and target endpoints, and when configuring virtual hosts that use 2-way TLS. When used with a target endpoint/target server, if the backend system uses SNI and returns a cert with a subject Distinguished Name (DN) that does not match the hostname, there is no way to ignore the error and the connection fails.
*
*/
public Boolean ignoreValidationErrors() {
return this.ignoreValidationErrors;
}
/**
* @return Required if `client_auth_enabled` is true. The resource ID for the alias containing the private key and cert.
*
*/
public String keyAlias() {
return this.keyAlias;
}
/**
* @return Required if `client_auth_enabled` is true. The resource ID of the keystore.
*
*/
public String keyStore() {
return this.keyStore;
}
/**
* @return The TLS versioins to be used.
*
*/
public List protocols() {
return this.protocols;
}
/**
* @return The resource ID of the truststore.
*
*/
public String trustStore() {
return this.trustStore;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GoogleCloudApigeeV1TlsInfoResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List ciphers;
private Boolean clientAuthEnabled;
private GoogleCloudApigeeV1TlsInfoCommonNameResponse commonName;
private Boolean enabled;
private Boolean ignoreValidationErrors;
private String keyAlias;
private String keyStore;
private List protocols;
private String trustStore;
public Builder() {}
public Builder(GoogleCloudApigeeV1TlsInfoResponse defaults) {
Objects.requireNonNull(defaults);
this.ciphers = defaults.ciphers;
this.clientAuthEnabled = defaults.clientAuthEnabled;
this.commonName = defaults.commonName;
this.enabled = defaults.enabled;
this.ignoreValidationErrors = defaults.ignoreValidationErrors;
this.keyAlias = defaults.keyAlias;
this.keyStore = defaults.keyStore;
this.protocols = defaults.protocols;
this.trustStore = defaults.trustStore;
}
@CustomType.Setter
public Builder ciphers(List ciphers) {
this.ciphers = Objects.requireNonNull(ciphers);
return this;
}
public Builder ciphers(String... ciphers) {
return ciphers(List.of(ciphers));
}
@CustomType.Setter
public Builder clientAuthEnabled(Boolean clientAuthEnabled) {
this.clientAuthEnabled = Objects.requireNonNull(clientAuthEnabled);
return this;
}
@CustomType.Setter
public Builder commonName(GoogleCloudApigeeV1TlsInfoCommonNameResponse commonName) {
this.commonName = Objects.requireNonNull(commonName);
return this;
}
@CustomType.Setter
public Builder enabled(Boolean enabled) {
this.enabled = Objects.requireNonNull(enabled);
return this;
}
@CustomType.Setter
public Builder ignoreValidationErrors(Boolean ignoreValidationErrors) {
this.ignoreValidationErrors = Objects.requireNonNull(ignoreValidationErrors);
return this;
}
@CustomType.Setter
public Builder keyAlias(String keyAlias) {
this.keyAlias = Objects.requireNonNull(keyAlias);
return this;
}
@CustomType.Setter
public Builder keyStore(String keyStore) {
this.keyStore = Objects.requireNonNull(keyStore);
return this;
}
@CustomType.Setter
public Builder protocols(List protocols) {
this.protocols = Objects.requireNonNull(protocols);
return this;
}
public Builder protocols(String... protocols) {
return protocols(List.of(protocols));
}
@CustomType.Setter
public Builder trustStore(String trustStore) {
this.trustStore = Objects.requireNonNull(trustStore);
return this;
}
public GoogleCloudApigeeV1TlsInfoResponse build() {
final var o = new GoogleCloudApigeeV1TlsInfoResponse();
o.ciphers = ciphers;
o.clientAuthEnabled = clientAuthEnabled;
o.commonName = commonName;
o.enabled = enabled;
o.ignoreValidationErrors = ignoreValidationErrors;
o.keyAlias = keyAlias;
o.keyStore = keyStore;
o.protocols = protocols;
o.trustStore = trustStore;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy