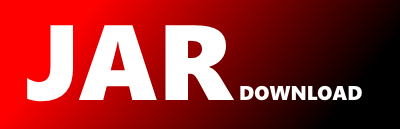
com.pulumi.googlenative.apigeeregistry.v1.outputs.GetDeploymentResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigeeregistry.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetDeploymentResult {
/**
* @return Text briefly describing how to access the endpoint. Changes to this value will not affect the revision.
*
*/
private String accessGuidance;
/**
* @return Annotations attach non-identifying metadata to resources. Annotation keys and values are less restricted than those of labels, but should be generally used for small values of broad interest. Larger, topic- specific metadata should be stored in Artifacts.
*
*/
private Map annotations;
/**
* @return The full resource name (including revision ID) of the spec of the API being served by the deployment. Changes to this value will update the revision. Format: `projects/{project}/locations/{location}/apis/{api}/versions/{version}/specs/{spec@revision}`
*
*/
private String apiSpecRevision;
/**
* @return Creation timestamp; when the deployment resource was created.
*
*/
private String createTime;
/**
* @return A detailed description.
*
*/
private String description;
/**
* @return Human-meaningful name.
*
*/
private String displayName;
/**
* @return The address where the deployment is serving. Changes to this value will update the revision.
*
*/
private String endpointUri;
/**
* @return The address of the external channel of the API (e.g., the Developer Portal). Changes to this value will not affect the revision.
*
*/
private String externalChannelUri;
/**
* @return Text briefly identifying the intended audience of the API. Changes to this value will not affect the revision.
*
*/
private String intendedAudience;
/**
* @return Labels attach identifying metadata to resources. Identifying metadata can be used to filter list operations. Label keys and values can be no longer than 64 characters (Unicode codepoints), can only contain lowercase letters, numeric characters, underscores and dashes. International characters are allowed. No more than 64 user labels can be associated with one resource (System labels are excluded). See https://goo.gl/xmQnxf for more information and examples of labels. System reserved label keys are prefixed with `apigeeregistry.googleapis.com/` and cannot be changed.
*
*/
private Map labels;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Revision creation timestamp; when the represented revision was created.
*
*/
private String revisionCreateTime;
/**
* @return Immutable. The revision ID of the deployment. A new revision is committed whenever the deployment contents are changed. The format is an 8-character hexadecimal string.
*
*/
private String revisionId;
/**
* @return Last update timestamp: when the represented revision was last modified.
*
*/
private String revisionUpdateTime;
private GetDeploymentResult() {}
/**
* @return Text briefly describing how to access the endpoint. Changes to this value will not affect the revision.
*
*/
public String accessGuidance() {
return this.accessGuidance;
}
/**
* @return Annotations attach non-identifying metadata to resources. Annotation keys and values are less restricted than those of labels, but should be generally used for small values of broad interest. Larger, topic- specific metadata should be stored in Artifacts.
*
*/
public Map annotations() {
return this.annotations;
}
/**
* @return The full resource name (including revision ID) of the spec of the API being served by the deployment. Changes to this value will update the revision. Format: `projects/{project}/locations/{location}/apis/{api}/versions/{version}/specs/{spec@revision}`
*
*/
public String apiSpecRevision() {
return this.apiSpecRevision;
}
/**
* @return Creation timestamp; when the deployment resource was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return A detailed description.
*
*/
public String description() {
return this.description;
}
/**
* @return Human-meaningful name.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return The address where the deployment is serving. Changes to this value will update the revision.
*
*/
public String endpointUri() {
return this.endpointUri;
}
/**
* @return The address of the external channel of the API (e.g., the Developer Portal). Changes to this value will not affect the revision.
*
*/
public String externalChannelUri() {
return this.externalChannelUri;
}
/**
* @return Text briefly identifying the intended audience of the API. Changes to this value will not affect the revision.
*
*/
public String intendedAudience() {
return this.intendedAudience;
}
/**
* @return Labels attach identifying metadata to resources. Identifying metadata can be used to filter list operations. Label keys and values can be no longer than 64 characters (Unicode codepoints), can only contain lowercase letters, numeric characters, underscores and dashes. International characters are allowed. No more than 64 user labels can be associated with one resource (System labels are excluded). See https://goo.gl/xmQnxf for more information and examples of labels. System reserved label keys are prefixed with `apigeeregistry.googleapis.com/` and cannot be changed.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Revision creation timestamp; when the represented revision was created.
*
*/
public String revisionCreateTime() {
return this.revisionCreateTime;
}
/**
* @return Immutable. The revision ID of the deployment. A new revision is committed whenever the deployment contents are changed. The format is an 8-character hexadecimal string.
*
*/
public String revisionId() {
return this.revisionId;
}
/**
* @return Last update timestamp: when the represented revision was last modified.
*
*/
public String revisionUpdateTime() {
return this.revisionUpdateTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDeploymentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessGuidance;
private Map annotations;
private String apiSpecRevision;
private String createTime;
private String description;
private String displayName;
private String endpointUri;
private String externalChannelUri;
private String intendedAudience;
private Map labels;
private String name;
private String revisionCreateTime;
private String revisionId;
private String revisionUpdateTime;
public Builder() {}
public Builder(GetDeploymentResult defaults) {
Objects.requireNonNull(defaults);
this.accessGuidance = defaults.accessGuidance;
this.annotations = defaults.annotations;
this.apiSpecRevision = defaults.apiSpecRevision;
this.createTime = defaults.createTime;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.endpointUri = defaults.endpointUri;
this.externalChannelUri = defaults.externalChannelUri;
this.intendedAudience = defaults.intendedAudience;
this.labels = defaults.labels;
this.name = defaults.name;
this.revisionCreateTime = defaults.revisionCreateTime;
this.revisionId = defaults.revisionId;
this.revisionUpdateTime = defaults.revisionUpdateTime;
}
@CustomType.Setter
public Builder accessGuidance(String accessGuidance) {
this.accessGuidance = Objects.requireNonNull(accessGuidance);
return this;
}
@CustomType.Setter
public Builder annotations(Map annotations) {
this.annotations = Objects.requireNonNull(annotations);
return this;
}
@CustomType.Setter
public Builder apiSpecRevision(String apiSpecRevision) {
this.apiSpecRevision = Objects.requireNonNull(apiSpecRevision);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
this.displayName = Objects.requireNonNull(displayName);
return this;
}
@CustomType.Setter
public Builder endpointUri(String endpointUri) {
this.endpointUri = Objects.requireNonNull(endpointUri);
return this;
}
@CustomType.Setter
public Builder externalChannelUri(String externalChannelUri) {
this.externalChannelUri = Objects.requireNonNull(externalChannelUri);
return this;
}
@CustomType.Setter
public Builder intendedAudience(String intendedAudience) {
this.intendedAudience = Objects.requireNonNull(intendedAudience);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder revisionCreateTime(String revisionCreateTime) {
this.revisionCreateTime = Objects.requireNonNull(revisionCreateTime);
return this;
}
@CustomType.Setter
public Builder revisionId(String revisionId) {
this.revisionId = Objects.requireNonNull(revisionId);
return this;
}
@CustomType.Setter
public Builder revisionUpdateTime(String revisionUpdateTime) {
this.revisionUpdateTime = Objects.requireNonNull(revisionUpdateTime);
return this;
}
public GetDeploymentResult build() {
final var o = new GetDeploymentResult();
o.accessGuidance = accessGuidance;
o.annotations = annotations;
o.apiSpecRevision = apiSpecRevision;
o.createTime = createTime;
o.description = description;
o.displayName = displayName;
o.endpointUri = endpointUri;
o.externalChannelUri = externalChannelUri;
o.intendedAudience = intendedAudience;
o.labels = labels;
o.name = name;
o.revisionCreateTime = revisionCreateTime;
o.revisionId = revisionId;
o.revisionUpdateTime = revisionUpdateTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy