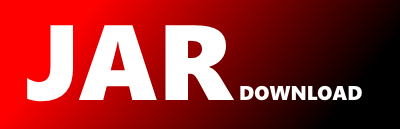
com.pulumi.googlenative.apigeeregistry.v1.outputs.GetSpecResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.apigeeregistry.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetSpecResult {
/**
* @return Annotations attach non-identifying metadata to resources. Annotation keys and values are less restricted than those of labels, but should be generally used for small values of broad interest. Larger, topic- specific metadata should be stored in Artifacts.
*
*/
private Map annotations;
/**
* @return Input only. The contents of the spec. Provided by API callers when specs are created or updated. To access the contents of a spec, use GetApiSpecContents.
*
*/
private String contents;
/**
* @return Creation timestamp; when the spec resource was created.
*
*/
private String createTime;
/**
* @return A detailed description.
*
*/
private String description;
/**
* @return A possibly-hierarchical name used to refer to the spec from other specs.
*
*/
private String filename;
/**
* @return A SHA-256 hash of the spec's contents. If the spec is gzipped, this is the hash of the uncompressed spec.
*
*/
private String hash;
/**
* @return Labels attach identifying metadata to resources. Identifying metadata can be used to filter list operations. Label keys and values can be no longer than 64 characters (Unicode codepoints), can only contain lowercase letters, numeric characters, underscores and dashes. International characters are allowed. No more than 64 user labels can be associated with one resource (System labels are excluded). See https://goo.gl/xmQnxf for more information and examples of labels. System reserved label keys are prefixed with `apigeeregistry.googleapis.com/` and cannot be changed.
*
*/
private Map labels;
/**
* @return A style (format) descriptor for this spec that is specified as a Media Type (https://en.wikipedia.org/wiki/Media_type). Possible values include `application/vnd.apigee.proto`, `application/vnd.apigee.openapi`, and `application/vnd.apigee.graphql`, with possible suffixes representing compression types. These hypothetical names are defined in the vendor tree defined in RFC6838 (https://tools.ietf.org/html/rfc6838) and are not final. Content types can specify compression. Currently only GZip compression is supported (indicated with "+gzip").
*
*/
private String mimeType;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Revision creation timestamp; when the represented revision was created.
*
*/
private String revisionCreateTime;
/**
* @return Immutable. The revision ID of the spec. A new revision is committed whenever the spec contents are changed. The format is an 8-character hexadecimal string.
*
*/
private String revisionId;
/**
* @return Last update timestamp: when the represented revision was last modified.
*
*/
private String revisionUpdateTime;
/**
* @return The size of the spec file in bytes. If the spec is gzipped, this is the size of the uncompressed spec.
*
*/
private Integer sizeBytes;
/**
* @return The original source URI of the spec (if one exists). This is an external location that can be used for reference purposes but which may not be authoritative since this external resource may change after the spec is retrieved.
*
*/
private String sourceUri;
private GetSpecResult() {}
/**
* @return Annotations attach non-identifying metadata to resources. Annotation keys and values are less restricted than those of labels, but should be generally used for small values of broad interest. Larger, topic- specific metadata should be stored in Artifacts.
*
*/
public Map annotations() {
return this.annotations;
}
/**
* @return Input only. The contents of the spec. Provided by API callers when specs are created or updated. To access the contents of a spec, use GetApiSpecContents.
*
*/
public String contents() {
return this.contents;
}
/**
* @return Creation timestamp; when the spec resource was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return A detailed description.
*
*/
public String description() {
return this.description;
}
/**
* @return A possibly-hierarchical name used to refer to the spec from other specs.
*
*/
public String filename() {
return this.filename;
}
/**
* @return A SHA-256 hash of the spec's contents. If the spec is gzipped, this is the hash of the uncompressed spec.
*
*/
public String hash() {
return this.hash;
}
/**
* @return Labels attach identifying metadata to resources. Identifying metadata can be used to filter list operations. Label keys and values can be no longer than 64 characters (Unicode codepoints), can only contain lowercase letters, numeric characters, underscores and dashes. International characters are allowed. No more than 64 user labels can be associated with one resource (System labels are excluded). See https://goo.gl/xmQnxf for more information and examples of labels. System reserved label keys are prefixed with `apigeeregistry.googleapis.com/` and cannot be changed.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return A style (format) descriptor for this spec that is specified as a Media Type (https://en.wikipedia.org/wiki/Media_type). Possible values include `application/vnd.apigee.proto`, `application/vnd.apigee.openapi`, and `application/vnd.apigee.graphql`, with possible suffixes representing compression types. These hypothetical names are defined in the vendor tree defined in RFC6838 (https://tools.ietf.org/html/rfc6838) and are not final. Content types can specify compression. Currently only GZip compression is supported (indicated with "+gzip").
*
*/
public String mimeType() {
return this.mimeType;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Revision creation timestamp; when the represented revision was created.
*
*/
public String revisionCreateTime() {
return this.revisionCreateTime;
}
/**
* @return Immutable. The revision ID of the spec. A new revision is committed whenever the spec contents are changed. The format is an 8-character hexadecimal string.
*
*/
public String revisionId() {
return this.revisionId;
}
/**
* @return Last update timestamp: when the represented revision was last modified.
*
*/
public String revisionUpdateTime() {
return this.revisionUpdateTime;
}
/**
* @return The size of the spec file in bytes. If the spec is gzipped, this is the size of the uncompressed spec.
*
*/
public Integer sizeBytes() {
return this.sizeBytes;
}
/**
* @return The original source URI of the spec (if one exists). This is an external location that can be used for reference purposes but which may not be authoritative since this external resource may change after the spec is retrieved.
*
*/
public String sourceUri() {
return this.sourceUri;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetSpecResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Map annotations;
private String contents;
private String createTime;
private String description;
private String filename;
private String hash;
private Map labels;
private String mimeType;
private String name;
private String revisionCreateTime;
private String revisionId;
private String revisionUpdateTime;
private Integer sizeBytes;
private String sourceUri;
public Builder() {}
public Builder(GetSpecResult defaults) {
Objects.requireNonNull(defaults);
this.annotations = defaults.annotations;
this.contents = defaults.contents;
this.createTime = defaults.createTime;
this.description = defaults.description;
this.filename = defaults.filename;
this.hash = defaults.hash;
this.labels = defaults.labels;
this.mimeType = defaults.mimeType;
this.name = defaults.name;
this.revisionCreateTime = defaults.revisionCreateTime;
this.revisionId = defaults.revisionId;
this.revisionUpdateTime = defaults.revisionUpdateTime;
this.sizeBytes = defaults.sizeBytes;
this.sourceUri = defaults.sourceUri;
}
@CustomType.Setter
public Builder annotations(Map annotations) {
this.annotations = Objects.requireNonNull(annotations);
return this;
}
@CustomType.Setter
public Builder contents(String contents) {
this.contents = Objects.requireNonNull(contents);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder filename(String filename) {
this.filename = Objects.requireNonNull(filename);
return this;
}
@CustomType.Setter
public Builder hash(String hash) {
this.hash = Objects.requireNonNull(hash);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder mimeType(String mimeType) {
this.mimeType = Objects.requireNonNull(mimeType);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder revisionCreateTime(String revisionCreateTime) {
this.revisionCreateTime = Objects.requireNonNull(revisionCreateTime);
return this;
}
@CustomType.Setter
public Builder revisionId(String revisionId) {
this.revisionId = Objects.requireNonNull(revisionId);
return this;
}
@CustomType.Setter
public Builder revisionUpdateTime(String revisionUpdateTime) {
this.revisionUpdateTime = Objects.requireNonNull(revisionUpdateTime);
return this;
}
@CustomType.Setter
public Builder sizeBytes(Integer sizeBytes) {
this.sizeBytes = Objects.requireNonNull(sizeBytes);
return this;
}
@CustomType.Setter
public Builder sourceUri(String sourceUri) {
this.sourceUri = Objects.requireNonNull(sourceUri);
return this;
}
public GetSpecResult build() {
final var o = new GetSpecResult();
o.annotations = annotations;
o.contents = contents;
o.createTime = createTime;
o.description = description;
o.filename = filename;
o.hash = hash;
o.labels = labels;
o.mimeType = mimeType;
o.name = name;
o.revisionCreateTime = revisionCreateTime;
o.revisionId = revisionId;
o.revisionUpdateTime = revisionUpdateTime;
o.sizeBytes = sizeBytes;
o.sourceUri = sourceUri;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy