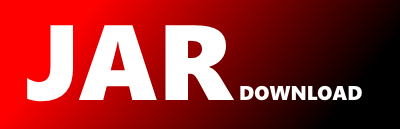
com.pulumi.googlenative.appengine.v1.inputs.AutomaticScalingArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.appengine.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.appengine.v1.inputs.CpuUtilizationArgs;
import com.pulumi.googlenative.appengine.v1.inputs.DiskUtilizationArgs;
import com.pulumi.googlenative.appengine.v1.inputs.NetworkUtilizationArgs;
import com.pulumi.googlenative.appengine.v1.inputs.RequestUtilizationArgs;
import com.pulumi.googlenative.appengine.v1.inputs.StandardSchedulerSettingsArgs;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Automatic scaling is based on request rate, response latencies, and other application metrics.
*
*/
public final class AutomaticScalingArgs extends com.pulumi.resources.ResourceArgs {
public static final AutomaticScalingArgs Empty = new AutomaticScalingArgs();
/**
* The time period that the Autoscaler (https://cloud.google.com/compute/docs/autoscaler/) should wait before it starts collecting information from a new instance. This prevents the autoscaler from collecting information when the instance is initializing, during which the collected usage would not be reliable. Only applicable in the App Engine flexible environment.
*
*/
@Import(name="coolDownPeriod")
private @Nullable Output coolDownPeriod;
/**
* @return The time period that the Autoscaler (https://cloud.google.com/compute/docs/autoscaler/) should wait before it starts collecting information from a new instance. This prevents the autoscaler from collecting information when the instance is initializing, during which the collected usage would not be reliable. Only applicable in the App Engine flexible environment.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy