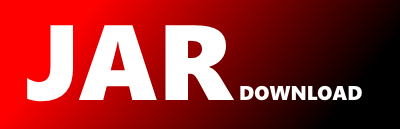
com.pulumi.googlenative.appengine.v1.outputs.AutomaticScalingResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.appengine.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.appengine.v1.outputs.CpuUtilizationResponse;
import com.pulumi.googlenative.appengine.v1.outputs.DiskUtilizationResponse;
import com.pulumi.googlenative.appengine.v1.outputs.NetworkUtilizationResponse;
import com.pulumi.googlenative.appengine.v1.outputs.RequestUtilizationResponse;
import com.pulumi.googlenative.appengine.v1.outputs.StandardSchedulerSettingsResponse;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class AutomaticScalingResponse {
/**
* @return The time period that the Autoscaler (https://cloud.google.com/compute/docs/autoscaler/) should wait before it starts collecting information from a new instance. This prevents the autoscaler from collecting information when the instance is initializing, during which the collected usage would not be reliable. Only applicable in the App Engine flexible environment.
*
*/
private String coolDownPeriod;
/**
* @return Target scaling by CPU usage.
*
*/
private CpuUtilizationResponse cpuUtilization;
/**
* @return Target scaling by disk usage.
*
*/
private DiskUtilizationResponse diskUtilization;
/**
* @return Number of concurrent requests an automatic scaling instance can accept before the scheduler spawns a new instance.Defaults to a runtime-specific value.
*
*/
private Integer maxConcurrentRequests;
/**
* @return Maximum number of idle instances that should be maintained for this version.
*
*/
private Integer maxIdleInstances;
/**
* @return Maximum amount of time that a request should wait in the pending queue before starting a new instance to handle it.
*
*/
private String maxPendingLatency;
/**
* @return Maximum number of instances that should be started to handle requests for this version.
*
*/
private Integer maxTotalInstances;
/**
* @return Minimum number of idle instances that should be maintained for this version. Only applicable for the default version of a service.
*
*/
private Integer minIdleInstances;
/**
* @return Minimum amount of time a request should wait in the pending queue before starting a new instance to handle it.
*
*/
private String minPendingLatency;
/**
* @return Minimum number of running instances that should be maintained for this version.
*
*/
private Integer minTotalInstances;
/**
* @return Target scaling by network usage.
*
*/
private NetworkUtilizationResponse networkUtilization;
/**
* @return Target scaling by request utilization.
*
*/
private RequestUtilizationResponse requestUtilization;
/**
* @return Scheduler settings for standard environment.
*
*/
private StandardSchedulerSettingsResponse standardSchedulerSettings;
private AutomaticScalingResponse() {}
/**
* @return The time period that the Autoscaler (https://cloud.google.com/compute/docs/autoscaler/) should wait before it starts collecting information from a new instance. This prevents the autoscaler from collecting information when the instance is initializing, during which the collected usage would not be reliable. Only applicable in the App Engine flexible environment.
*
*/
public String coolDownPeriod() {
return this.coolDownPeriod;
}
/**
* @return Target scaling by CPU usage.
*
*/
public CpuUtilizationResponse cpuUtilization() {
return this.cpuUtilization;
}
/**
* @return Target scaling by disk usage.
*
*/
public DiskUtilizationResponse diskUtilization() {
return this.diskUtilization;
}
/**
* @return Number of concurrent requests an automatic scaling instance can accept before the scheduler spawns a new instance.Defaults to a runtime-specific value.
*
*/
public Integer maxConcurrentRequests() {
return this.maxConcurrentRequests;
}
/**
* @return Maximum number of idle instances that should be maintained for this version.
*
*/
public Integer maxIdleInstances() {
return this.maxIdleInstances;
}
/**
* @return Maximum amount of time that a request should wait in the pending queue before starting a new instance to handle it.
*
*/
public String maxPendingLatency() {
return this.maxPendingLatency;
}
/**
* @return Maximum number of instances that should be started to handle requests for this version.
*
*/
public Integer maxTotalInstances() {
return this.maxTotalInstances;
}
/**
* @return Minimum number of idle instances that should be maintained for this version. Only applicable for the default version of a service.
*
*/
public Integer minIdleInstances() {
return this.minIdleInstances;
}
/**
* @return Minimum amount of time a request should wait in the pending queue before starting a new instance to handle it.
*
*/
public String minPendingLatency() {
return this.minPendingLatency;
}
/**
* @return Minimum number of running instances that should be maintained for this version.
*
*/
public Integer minTotalInstances() {
return this.minTotalInstances;
}
/**
* @return Target scaling by network usage.
*
*/
public NetworkUtilizationResponse networkUtilization() {
return this.networkUtilization;
}
/**
* @return Target scaling by request utilization.
*
*/
public RequestUtilizationResponse requestUtilization() {
return this.requestUtilization;
}
/**
* @return Scheduler settings for standard environment.
*
*/
public StandardSchedulerSettingsResponse standardSchedulerSettings() {
return this.standardSchedulerSettings;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AutomaticScalingResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String coolDownPeriod;
private CpuUtilizationResponse cpuUtilization;
private DiskUtilizationResponse diskUtilization;
private Integer maxConcurrentRequests;
private Integer maxIdleInstances;
private String maxPendingLatency;
private Integer maxTotalInstances;
private Integer minIdleInstances;
private String minPendingLatency;
private Integer minTotalInstances;
private NetworkUtilizationResponse networkUtilization;
private RequestUtilizationResponse requestUtilization;
private StandardSchedulerSettingsResponse standardSchedulerSettings;
public Builder() {}
public Builder(AutomaticScalingResponse defaults) {
Objects.requireNonNull(defaults);
this.coolDownPeriod = defaults.coolDownPeriod;
this.cpuUtilization = defaults.cpuUtilization;
this.diskUtilization = defaults.diskUtilization;
this.maxConcurrentRequests = defaults.maxConcurrentRequests;
this.maxIdleInstances = defaults.maxIdleInstances;
this.maxPendingLatency = defaults.maxPendingLatency;
this.maxTotalInstances = defaults.maxTotalInstances;
this.minIdleInstances = defaults.minIdleInstances;
this.minPendingLatency = defaults.minPendingLatency;
this.minTotalInstances = defaults.minTotalInstances;
this.networkUtilization = defaults.networkUtilization;
this.requestUtilization = defaults.requestUtilization;
this.standardSchedulerSettings = defaults.standardSchedulerSettings;
}
@CustomType.Setter
public Builder coolDownPeriod(String coolDownPeriod) {
this.coolDownPeriod = Objects.requireNonNull(coolDownPeriod);
return this;
}
@CustomType.Setter
public Builder cpuUtilization(CpuUtilizationResponse cpuUtilization) {
this.cpuUtilization = Objects.requireNonNull(cpuUtilization);
return this;
}
@CustomType.Setter
public Builder diskUtilization(DiskUtilizationResponse diskUtilization) {
this.diskUtilization = Objects.requireNonNull(diskUtilization);
return this;
}
@CustomType.Setter
public Builder maxConcurrentRequests(Integer maxConcurrentRequests) {
this.maxConcurrentRequests = Objects.requireNonNull(maxConcurrentRequests);
return this;
}
@CustomType.Setter
public Builder maxIdleInstances(Integer maxIdleInstances) {
this.maxIdleInstances = Objects.requireNonNull(maxIdleInstances);
return this;
}
@CustomType.Setter
public Builder maxPendingLatency(String maxPendingLatency) {
this.maxPendingLatency = Objects.requireNonNull(maxPendingLatency);
return this;
}
@CustomType.Setter
public Builder maxTotalInstances(Integer maxTotalInstances) {
this.maxTotalInstances = Objects.requireNonNull(maxTotalInstances);
return this;
}
@CustomType.Setter
public Builder minIdleInstances(Integer minIdleInstances) {
this.minIdleInstances = Objects.requireNonNull(minIdleInstances);
return this;
}
@CustomType.Setter
public Builder minPendingLatency(String minPendingLatency) {
this.minPendingLatency = Objects.requireNonNull(minPendingLatency);
return this;
}
@CustomType.Setter
public Builder minTotalInstances(Integer minTotalInstances) {
this.minTotalInstances = Objects.requireNonNull(minTotalInstances);
return this;
}
@CustomType.Setter
public Builder networkUtilization(NetworkUtilizationResponse networkUtilization) {
this.networkUtilization = Objects.requireNonNull(networkUtilization);
return this;
}
@CustomType.Setter
public Builder requestUtilization(RequestUtilizationResponse requestUtilization) {
this.requestUtilization = Objects.requireNonNull(requestUtilization);
return this;
}
@CustomType.Setter
public Builder standardSchedulerSettings(StandardSchedulerSettingsResponse standardSchedulerSettings) {
this.standardSchedulerSettings = Objects.requireNonNull(standardSchedulerSettings);
return this;
}
public AutomaticScalingResponse build() {
final var o = new AutomaticScalingResponse();
o.coolDownPeriod = coolDownPeriod;
o.cpuUtilization = cpuUtilization;
o.diskUtilization = diskUtilization;
o.maxConcurrentRequests = maxConcurrentRequests;
o.maxIdleInstances = maxIdleInstances;
o.maxPendingLatency = maxPendingLatency;
o.maxTotalInstances = maxTotalInstances;
o.minIdleInstances = minIdleInstances;
o.minPendingLatency = minPendingLatency;
o.minTotalInstances = minTotalInstances;
o.networkUtilization = networkUtilization;
o.requestUtilization = requestUtilization;
o.standardSchedulerSettings = standardSchedulerSettings;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy