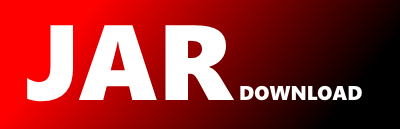
com.pulumi.googlenative.assuredworkloads.v1.Workload Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.assuredworkloads.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.assuredworkloads.v1.WorkloadArgs;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadComplianceStatusResponse;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadEkmProvisioningResponseResponse;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadKMSSettingsResponse;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadPartnerPermissionsResponse;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadResourceInfoResponse;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadResourceSettingsResponse;
import com.pulumi.googlenative.assuredworkloads.v1.outputs.GoogleCloudAssuredworkloadsV1WorkloadSaaEnrollmentResponseResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates Assured Workload.
*
*/
@ResourceType(type="google-native:assuredworkloads/v1:Workload")
public class Workload extends com.pulumi.resources.CustomResource {
/**
* Optional. The billing account used for the resources which are direct children of workload. This billing account is initially associated with the resources created as part of Workload creation. After the initial creation of these resources, the customer can change the assigned billing account. The resource name has the form `billingAccounts/{billing_account_id}`. For example, `billingAccounts/012345-567890-ABCDEF`.
*
*/
@Export(name="billingAccount", type=String.class, parameters={})
private Output billingAccount;
/**
* @return Optional. The billing account used for the resources which are direct children of workload. This billing account is initially associated with the resources created as part of Workload creation. After the initial creation of these resources, the customer can change the assigned billing account. The resource name has the form `billingAccounts/{billing_account_id}`. For example, `billingAccounts/012345-567890-ABCDEF`.
*
*/
public Output billingAccount() {
return this.billingAccount;
}
/**
* Immutable. Compliance Regime associated with this workload.
*
*/
@Export(name="complianceRegime", type=String.class, parameters={})
private Output complianceRegime;
/**
* @return Immutable. Compliance Regime associated with this workload.
*
*/
public Output complianceRegime() {
return this.complianceRegime;
}
/**
* Count of active Violations in the Workload.
*
*/
@Export(name="complianceStatus", type=GoogleCloudAssuredworkloadsV1WorkloadComplianceStatusResponse.class, parameters={})
private Output complianceStatus;
/**
* @return Count of active Violations in the Workload.
*
*/
public Output complianceStatus() {
return this.complianceStatus;
}
/**
* Urls for services which are compliant for this Assured Workload, but which are currently disallowed by the ResourceUsageRestriction org policy. Invoke RestrictAllowedResources endpoint to allow your project developers to use these services in their environment."
*
*/
@Export(name="compliantButDisallowedServices", type=List.class, parameters={String.class})
private Output> compliantButDisallowedServices;
/**
* @return Urls for services which are compliant for this Assured Workload, but which are currently disallowed by the ResourceUsageRestriction org policy. Invoke RestrictAllowedResources endpoint to allow your project developers to use these services in their environment."
*
*/
public Output> compliantButDisallowedServices() {
return this.compliantButDisallowedServices;
}
/**
* Immutable. The Workload creation timestamp.
*
*/
@Export(name="createTime", type=String.class, parameters={})
private Output createTime;
/**
* @return Immutable. The Workload creation timestamp.
*
*/
public Output createTime() {
return this.createTime;
}
/**
* The user-assigned display name of the Workload. When present it must be between 4 to 30 characters. Allowed characters are: lowercase and uppercase letters, numbers, hyphen, and spaces. Example: My Workload
*
*/
@Export(name="displayName", type=String.class, parameters={})
private Output displayName;
/**
* @return The user-assigned display name of the Workload. When present it must be between 4 to 30 characters. Allowed characters are: lowercase and uppercase letters, numbers, hyphen, and spaces. Example: My Workload
*
*/
public Output displayName() {
return this.displayName;
}
/**
* Optional. Represents the Ekm Provisioning State of the given workload.
*
*/
@Export(name="ekmProvisioningResponse", type=GoogleCloudAssuredworkloadsV1WorkloadEkmProvisioningResponseResponse.class, parameters={})
private Output ekmProvisioningResponse;
/**
* @return Optional. Represents the Ekm Provisioning State of the given workload.
*
*/
public Output ekmProvisioningResponse() {
return this.ekmProvisioningResponse;
}
/**
* Optional. Indicates the sovereignty status of the given workload. Currently meant to be used by Europe/Canada customers.
*
*/
@Export(name="enableSovereignControls", type=Boolean.class, parameters={})
private Output enableSovereignControls;
/**
* @return Optional. Indicates the sovereignty status of the given workload. Currently meant to be used by Europe/Canada customers.
*
*/
public Output enableSovereignControls() {
return this.enableSovereignControls;
}
/**
* Optional. ETag of the workload, it is calculated on the basis of the Workload contents. It will be used in Update & Delete operations.
*
*/
@Export(name="etag", type=String.class, parameters={})
private Output etag;
/**
* @return Optional. ETag of the workload, it is calculated on the basis of the Workload contents. It will be used in Update & Delete operations.
*
*/
public Output etag() {
return this.etag;
}
/**
* Optional. A identifier associated with the workload and underlying projects which allows for the break down of billing costs for a workload. The value provided for the identifier will add a label to the workload and contained projects with the identifier as the value.
*
*/
@Export(name="externalId", type=String.class, parameters={})
private Output* @Nullable */ String> externalId;
/**
* @return Optional. A identifier associated with the workload and underlying projects which allows for the break down of billing costs for a workload. The value provided for the identifier will add a label to the workload and contained projects with the identifier as the value.
*
*/
public Output> externalId() {
return Codegen.optional(this.externalId);
}
/**
* Represents the KAJ enrollment state of the given workload.
*
*/
@Export(name="kajEnrollmentState", type=String.class, parameters={})
private Output kajEnrollmentState;
/**
* @return Represents the KAJ enrollment state of the given workload.
*
*/
public Output kajEnrollmentState() {
return this.kajEnrollmentState;
}
/**
* Input only. Settings used to create a CMEK crypto key. When set, a project with a KMS CMEK key is provisioned. This field is deprecated as of Feb 28, 2022. In order to create a Keyring, callers should specify, ENCRYPTION_KEYS_PROJECT or KEYRING in ResourceSettings.resource_type field.
*
* @deprecated
* Input only. Settings used to create a CMEK crypto key. When set, a project with a KMS CMEK key is provisioned. This field is deprecated as of Feb 28, 2022. In order to create a Keyring, callers should specify, ENCRYPTION_KEYS_PROJECT or KEYRING in ResourceSettings.resource_type field.
*
*/
@Deprecated /* Input only. Settings used to create a CMEK crypto key. When set, a project with a KMS CMEK key is provisioned. This field is deprecated as of Feb 28, 2022. In order to create a Keyring, callers should specify, ENCRYPTION_KEYS_PROJECT or KEYRING in ResourceSettings.resource_type field. */
@Export(name="kmsSettings", type=GoogleCloudAssuredworkloadsV1WorkloadKMSSettingsResponse.class, parameters={})
private Output kmsSettings;
/**
* @return Input only. Settings used to create a CMEK crypto key. When set, a project with a KMS CMEK key is provisioned. This field is deprecated as of Feb 28, 2022. In order to create a Keyring, callers should specify, ENCRYPTION_KEYS_PROJECT or KEYRING in ResourceSettings.resource_type field.
*
*/
public Output kmsSettings() {
return this.kmsSettings;
}
/**
* Optional. Labels applied to the workload.
*
*/
@Export(name="labels", type=Map.class, parameters={String.class, String.class})
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy