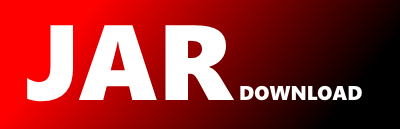
com.pulumi.googlenative.baremetalsolution.v2.inputs.InstanceConfigArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.baremetalsolution.v2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.baremetalsolution.v2.enums.InstanceConfigNetworkConfig;
import com.pulumi.googlenative.baremetalsolution.v2.inputs.GoogleCloudBaremetalsolutionV2LogicalInterfaceArgs;
import com.pulumi.googlenative.baremetalsolution.v2.inputs.NetworkAddressArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configuration parameters for a new instance.
*
*/
public final class InstanceConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final InstanceConfigArgs Empty = new InstanceConfigArgs();
/**
* If true networks can be from different projects of the same vendor account.
*
*/
@Import(name="accountNetworksEnabled")
private @Nullable Output accountNetworksEnabled;
/**
* @return If true networks can be from different projects of the same vendor account.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy