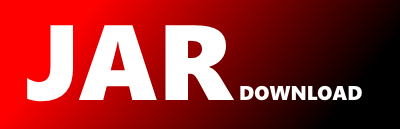
com.pulumi.googlenative.baremetalsolution.v2.inputs.LunArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.baremetalsolution.v2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.baremetalsolution.v2.enums.LunMultiprotocolType;
import com.pulumi.googlenative.baremetalsolution.v2.enums.LunState;
import com.pulumi.googlenative.baremetalsolution.v2.enums.LunStorageType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A storage volume logical unit number (LUN).
*
*/
public final class LunArgs extends com.pulumi.resources.ResourceArgs {
public static final LunArgs Empty = new LunArgs();
/**
* Display if this LUN is a boot LUN.
*
*/
@Import(name="bootLun")
private @Nullable Output bootLun;
/**
* @return Display if this LUN is a boot LUN.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy