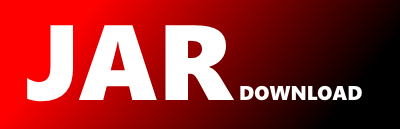
com.pulumi.googlenative.baremetalsolution.v2.outputs.GetProvisioningConfigResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.baremetalsolution.v2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.InstanceConfigResponse;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.NetworkConfigResponse;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.VolumeConfigResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetProvisioningConfigResult {
/**
* @return URI to Cloud Console UI view of this provisioning config.
*
*/
private String cloudConsoleUri;
/**
* @return Optional. The user-defined identifier of the provisioning config.
*
*/
private String customId;
/**
* @return Email provided to send a confirmation with provisioning config to. Deprecated in favour of email field in request messages.
*
* @deprecated
* Email provided to send a confirmation with provisioning config to. Deprecated in favour of email field in request messages.
*
*/
@Deprecated /* Email provided to send a confirmation with provisioning config to. Deprecated in favour of email field in request messages. */
private String email;
/**
* @return A service account to enable customers to access instance credentials upon handover.
*
*/
private String handoverServiceAccount;
/**
* @return Instances to be created.
*
*/
private List instances;
/**
* @return Optional. Location name of this ProvisioningConfig. It is optional only for Intake UI transition period.
*
*/
private String location;
/**
* @return The system-generated name of the provisioning config. This follows the UUID format.
*
*/
private String name;
/**
* @return Networks to be created.
*
*/
private List networks;
/**
* @return State of ProvisioningConfig.
*
*/
private String state;
/**
* @return Optional status messages associated with the FAILED state.
*
*/
private String statusMessage;
/**
* @return A generated ticket id to track provisioning request.
*
*/
private String ticketId;
/**
* @return Last update timestamp.
*
*/
private String updateTime;
/**
* @return Volumes to be created.
*
*/
private List volumes;
/**
* @return If true, VPC SC is enabled for the cluster.
*
*/
private Boolean vpcScEnabled;
private GetProvisioningConfigResult() {}
/**
* @return URI to Cloud Console UI view of this provisioning config.
*
*/
public String cloudConsoleUri() {
return this.cloudConsoleUri;
}
/**
* @return Optional. The user-defined identifier of the provisioning config.
*
*/
public String customId() {
return this.customId;
}
/**
* @return Email provided to send a confirmation with provisioning config to. Deprecated in favour of email field in request messages.
*
* @deprecated
* Email provided to send a confirmation with provisioning config to. Deprecated in favour of email field in request messages.
*
*/
@Deprecated /* Email provided to send a confirmation with provisioning config to. Deprecated in favour of email field in request messages. */
public String email() {
return this.email;
}
/**
* @return A service account to enable customers to access instance credentials upon handover.
*
*/
public String handoverServiceAccount() {
return this.handoverServiceAccount;
}
/**
* @return Instances to be created.
*
*/
public List instances() {
return this.instances;
}
/**
* @return Optional. Location name of this ProvisioningConfig. It is optional only for Intake UI transition period.
*
*/
public String location() {
return this.location;
}
/**
* @return The system-generated name of the provisioning config. This follows the UUID format.
*
*/
public String name() {
return this.name;
}
/**
* @return Networks to be created.
*
*/
public List networks() {
return this.networks;
}
/**
* @return State of ProvisioningConfig.
*
*/
public String state() {
return this.state;
}
/**
* @return Optional status messages associated with the FAILED state.
*
*/
public String statusMessage() {
return this.statusMessage;
}
/**
* @return A generated ticket id to track provisioning request.
*
*/
public String ticketId() {
return this.ticketId;
}
/**
* @return Last update timestamp.
*
*/
public String updateTime() {
return this.updateTime;
}
/**
* @return Volumes to be created.
*
*/
public List volumes() {
return this.volumes;
}
/**
* @return If true, VPC SC is enabled for the cluster.
*
*/
public Boolean vpcScEnabled() {
return this.vpcScEnabled;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetProvisioningConfigResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String cloudConsoleUri;
private String customId;
private String email;
private String handoverServiceAccount;
private List instances;
private String location;
private String name;
private List networks;
private String state;
private String statusMessage;
private String ticketId;
private String updateTime;
private List volumes;
private Boolean vpcScEnabled;
public Builder() {}
public Builder(GetProvisioningConfigResult defaults) {
Objects.requireNonNull(defaults);
this.cloudConsoleUri = defaults.cloudConsoleUri;
this.customId = defaults.customId;
this.email = defaults.email;
this.handoverServiceAccount = defaults.handoverServiceAccount;
this.instances = defaults.instances;
this.location = defaults.location;
this.name = defaults.name;
this.networks = defaults.networks;
this.state = defaults.state;
this.statusMessage = defaults.statusMessage;
this.ticketId = defaults.ticketId;
this.updateTime = defaults.updateTime;
this.volumes = defaults.volumes;
this.vpcScEnabled = defaults.vpcScEnabled;
}
@CustomType.Setter
public Builder cloudConsoleUri(String cloudConsoleUri) {
this.cloudConsoleUri = Objects.requireNonNull(cloudConsoleUri);
return this;
}
@CustomType.Setter
public Builder customId(String customId) {
this.customId = Objects.requireNonNull(customId);
return this;
}
@CustomType.Setter
public Builder email(String email) {
this.email = Objects.requireNonNull(email);
return this;
}
@CustomType.Setter
public Builder handoverServiceAccount(String handoverServiceAccount) {
this.handoverServiceAccount = Objects.requireNonNull(handoverServiceAccount);
return this;
}
@CustomType.Setter
public Builder instances(List instances) {
this.instances = Objects.requireNonNull(instances);
return this;
}
public Builder instances(InstanceConfigResponse... instances) {
return instances(List.of(instances));
}
@CustomType.Setter
public Builder location(String location) {
this.location = Objects.requireNonNull(location);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder networks(List networks) {
this.networks = Objects.requireNonNull(networks);
return this;
}
public Builder networks(NetworkConfigResponse... networks) {
return networks(List.of(networks));
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder statusMessage(String statusMessage) {
this.statusMessage = Objects.requireNonNull(statusMessage);
return this;
}
@CustomType.Setter
public Builder ticketId(String ticketId) {
this.ticketId = Objects.requireNonNull(ticketId);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
@CustomType.Setter
public Builder volumes(List volumes) {
this.volumes = Objects.requireNonNull(volumes);
return this;
}
public Builder volumes(VolumeConfigResponse... volumes) {
return volumes(List.of(volumes));
}
@CustomType.Setter
public Builder vpcScEnabled(Boolean vpcScEnabled) {
this.vpcScEnabled = Objects.requireNonNull(vpcScEnabled);
return this;
}
public GetProvisioningConfigResult build() {
final var o = new GetProvisioningConfigResult();
o.cloudConsoleUri = cloudConsoleUri;
o.customId = customId;
o.email = email;
o.handoverServiceAccount = handoverServiceAccount;
o.instances = instances;
o.location = location;
o.name = name;
o.networks = networks;
o.state = state;
o.statusMessage = statusMessage;
o.ticketId = ticketId;
o.updateTime = updateTime;
o.volumes = volumes;
o.vpcScEnabled = vpcScEnabled;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy