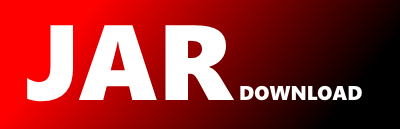
com.pulumi.googlenative.baremetalsolution.v2.outputs.InstanceConfigResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.baremetalsolution.v2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.GoogleCloudBaremetalsolutionV2LogicalInterfaceResponse;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.NetworkAddressResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class InstanceConfigResponse {
/**
* @return If true networks can be from different projects of the same vendor account.
*
*/
private Boolean accountNetworksEnabled;
/**
* @return Client network address. Filled if InstanceConfig.multivlan_config is false.
*
*/
private NetworkAddressResponse clientNetwork;
/**
* @return Whether the instance should be provisioned with Hyperthreading enabled.
*
*/
private Boolean hyperthreading;
/**
* @return Instance type. [Available types](https://cloud.google.com/bare-metal/docs/bms-planning#server_configurations)
*
*/
private String instanceType;
/**
* @return List of logical interfaces for the instance. The number of logical interfaces will be the same as number of hardware bond/nic on the chosen network template. Filled if InstanceConfig.multivlan_config is true.
*
*/
private List logicalInterfaces;
/**
* @return The name of the instance config.
*
*/
private String name;
/**
* @return The type of network configuration on the instance.
*
*/
private String networkConfig;
/**
* @return Server network template name. Filled if InstanceConfig.multivlan_config is true.
*
*/
private String networkTemplate;
/**
* @return OS image to initialize the instance. [Available images](https://cloud.google.com/bare-metal/docs/bms-planning#server_configurations)
*
*/
private String osImage;
/**
* @return Private network address, if any. Filled if InstanceConfig.multivlan_config is false.
*
*/
private NetworkAddressResponse privateNetwork;
/**
* @return User note field, it can be used by customers to add additional information for the BMS Ops team .
*
*/
private String userNote;
private InstanceConfigResponse() {}
/**
* @return If true networks can be from different projects of the same vendor account.
*
*/
public Boolean accountNetworksEnabled() {
return this.accountNetworksEnabled;
}
/**
* @return Client network address. Filled if InstanceConfig.multivlan_config is false.
*
*/
public NetworkAddressResponse clientNetwork() {
return this.clientNetwork;
}
/**
* @return Whether the instance should be provisioned with Hyperthreading enabled.
*
*/
public Boolean hyperthreading() {
return this.hyperthreading;
}
/**
* @return Instance type. [Available types](https://cloud.google.com/bare-metal/docs/bms-planning#server_configurations)
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return List of logical interfaces for the instance. The number of logical interfaces will be the same as number of hardware bond/nic on the chosen network template. Filled if InstanceConfig.multivlan_config is true.
*
*/
public List logicalInterfaces() {
return this.logicalInterfaces;
}
/**
* @return The name of the instance config.
*
*/
public String name() {
return this.name;
}
/**
* @return The type of network configuration on the instance.
*
*/
public String networkConfig() {
return this.networkConfig;
}
/**
* @return Server network template name. Filled if InstanceConfig.multivlan_config is true.
*
*/
public String networkTemplate() {
return this.networkTemplate;
}
/**
* @return OS image to initialize the instance. [Available images](https://cloud.google.com/bare-metal/docs/bms-planning#server_configurations)
*
*/
public String osImage() {
return this.osImage;
}
/**
* @return Private network address, if any. Filled if InstanceConfig.multivlan_config is false.
*
*/
public NetworkAddressResponse privateNetwork() {
return this.privateNetwork;
}
/**
* @return User note field, it can be used by customers to add additional information for the BMS Ops team .
*
*/
public String userNote() {
return this.userNote;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InstanceConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean accountNetworksEnabled;
private NetworkAddressResponse clientNetwork;
private Boolean hyperthreading;
private String instanceType;
private List logicalInterfaces;
private String name;
private String networkConfig;
private String networkTemplate;
private String osImage;
private NetworkAddressResponse privateNetwork;
private String userNote;
public Builder() {}
public Builder(InstanceConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.accountNetworksEnabled = defaults.accountNetworksEnabled;
this.clientNetwork = defaults.clientNetwork;
this.hyperthreading = defaults.hyperthreading;
this.instanceType = defaults.instanceType;
this.logicalInterfaces = defaults.logicalInterfaces;
this.name = defaults.name;
this.networkConfig = defaults.networkConfig;
this.networkTemplate = defaults.networkTemplate;
this.osImage = defaults.osImage;
this.privateNetwork = defaults.privateNetwork;
this.userNote = defaults.userNote;
}
@CustomType.Setter
public Builder accountNetworksEnabled(Boolean accountNetworksEnabled) {
this.accountNetworksEnabled = Objects.requireNonNull(accountNetworksEnabled);
return this;
}
@CustomType.Setter
public Builder clientNetwork(NetworkAddressResponse clientNetwork) {
this.clientNetwork = Objects.requireNonNull(clientNetwork);
return this;
}
@CustomType.Setter
public Builder hyperthreading(Boolean hyperthreading) {
this.hyperthreading = Objects.requireNonNull(hyperthreading);
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
this.instanceType = Objects.requireNonNull(instanceType);
return this;
}
@CustomType.Setter
public Builder logicalInterfaces(List logicalInterfaces) {
this.logicalInterfaces = Objects.requireNonNull(logicalInterfaces);
return this;
}
public Builder logicalInterfaces(GoogleCloudBaremetalsolutionV2LogicalInterfaceResponse... logicalInterfaces) {
return logicalInterfaces(List.of(logicalInterfaces));
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder networkConfig(String networkConfig) {
this.networkConfig = Objects.requireNonNull(networkConfig);
return this;
}
@CustomType.Setter
public Builder networkTemplate(String networkTemplate) {
this.networkTemplate = Objects.requireNonNull(networkTemplate);
return this;
}
@CustomType.Setter
public Builder osImage(String osImage) {
this.osImage = Objects.requireNonNull(osImage);
return this;
}
@CustomType.Setter
public Builder privateNetwork(NetworkAddressResponse privateNetwork) {
this.privateNetwork = Objects.requireNonNull(privateNetwork);
return this;
}
@CustomType.Setter
public Builder userNote(String userNote) {
this.userNote = Objects.requireNonNull(userNote);
return this;
}
public InstanceConfigResponse build() {
final var o = new InstanceConfigResponse();
o.accountNetworksEnabled = accountNetworksEnabled;
o.clientNetwork = clientNetwork;
o.hyperthreading = hyperthreading;
o.instanceType = instanceType;
o.logicalInterfaces = logicalInterfaces;
o.name = name;
o.networkConfig = networkConfig;
o.networkTemplate = networkTemplate;
o.osImage = osImage;
o.privateNetwork = privateNetwork;
o.userNote = userNote;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy