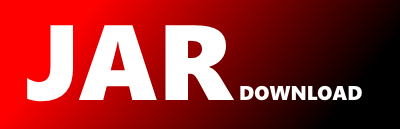
com.pulumi.googlenative.baremetalsolution.v2.outputs.NetworkResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.baremetalsolution.v2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.NetworkAddressReservationResponse;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.NetworkMountPointResponse;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.VRFResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class NetworkResponse {
/**
* @return The cidr of the Network.
*
*/
private String cidr;
/**
* @return Gateway ip address.
*
*/
private String gatewayIp;
/**
* @return IP address configured.
*
*/
private String ipAddress;
/**
* @return Whether network uses standard frames or jumbo ones.
*
*/
private Boolean jumboFramesEnabled;
/**
* @return Labels as key value pairs.
*
*/
private Map labels;
/**
* @return List of physical interfaces.
*
*/
private List macAddress;
/**
* @return Input only. List of mount points to attach the network to.
*
*/
private List mountPoints;
/**
* @return The resource name of this `Network`. Resource names are schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. Format: `projects/{project}/locations/{location}/networks/{network}`
*
*/
private String name;
/**
* @return Pod name.
*
*/
private String pod;
/**
* @return List of IP address reservations in this network. When updating this field, an error will be generated if a reservation conflicts with an IP address already allocated to a physical server.
*
*/
private List reservations;
/**
* @return IP range for reserved for services (e.g. NFS).
*
*/
private String servicesCidr;
/**
* @return The Network state.
*
*/
private String state;
/**
* @return The type of this network.
*
*/
private String type;
/**
* @return The vlan id of the Network.
*
*/
private String vlanId;
/**
* @return The vrf for the Network.
*
*/
private VRFResponse vrf;
private NetworkResponse() {}
/**
* @return The cidr of the Network.
*
*/
public String cidr() {
return this.cidr;
}
/**
* @return Gateway ip address.
*
*/
public String gatewayIp() {
return this.gatewayIp;
}
/**
* @return IP address configured.
*
*/
public String ipAddress() {
return this.ipAddress;
}
/**
* @return Whether network uses standard frames or jumbo ones.
*
*/
public Boolean jumboFramesEnabled() {
return this.jumboFramesEnabled;
}
/**
* @return Labels as key value pairs.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return List of physical interfaces.
*
*/
public List macAddress() {
return this.macAddress;
}
/**
* @return Input only. List of mount points to attach the network to.
*
*/
public List mountPoints() {
return this.mountPoints;
}
/**
* @return The resource name of this `Network`. Resource names are schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. Format: `projects/{project}/locations/{location}/networks/{network}`
*
*/
public String name() {
return this.name;
}
/**
* @return Pod name.
*
*/
public String pod() {
return this.pod;
}
/**
* @return List of IP address reservations in this network. When updating this field, an error will be generated if a reservation conflicts with an IP address already allocated to a physical server.
*
*/
public List reservations() {
return this.reservations;
}
/**
* @return IP range for reserved for services (e.g. NFS).
*
*/
public String servicesCidr() {
return this.servicesCidr;
}
/**
* @return The Network state.
*
*/
public String state() {
return this.state;
}
/**
* @return The type of this network.
*
*/
public String type() {
return this.type;
}
/**
* @return The vlan id of the Network.
*
*/
public String vlanId() {
return this.vlanId;
}
/**
* @return The vrf for the Network.
*
*/
public VRFResponse vrf() {
return this.vrf;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NetworkResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String cidr;
private String gatewayIp;
private String ipAddress;
private Boolean jumboFramesEnabled;
private Map labels;
private List macAddress;
private List mountPoints;
private String name;
private String pod;
private List reservations;
private String servicesCidr;
private String state;
private String type;
private String vlanId;
private VRFResponse vrf;
public Builder() {}
public Builder(NetworkResponse defaults) {
Objects.requireNonNull(defaults);
this.cidr = defaults.cidr;
this.gatewayIp = defaults.gatewayIp;
this.ipAddress = defaults.ipAddress;
this.jumboFramesEnabled = defaults.jumboFramesEnabled;
this.labels = defaults.labels;
this.macAddress = defaults.macAddress;
this.mountPoints = defaults.mountPoints;
this.name = defaults.name;
this.pod = defaults.pod;
this.reservations = defaults.reservations;
this.servicesCidr = defaults.servicesCidr;
this.state = defaults.state;
this.type = defaults.type;
this.vlanId = defaults.vlanId;
this.vrf = defaults.vrf;
}
@CustomType.Setter
public Builder cidr(String cidr) {
this.cidr = Objects.requireNonNull(cidr);
return this;
}
@CustomType.Setter
public Builder gatewayIp(String gatewayIp) {
this.gatewayIp = Objects.requireNonNull(gatewayIp);
return this;
}
@CustomType.Setter
public Builder ipAddress(String ipAddress) {
this.ipAddress = Objects.requireNonNull(ipAddress);
return this;
}
@CustomType.Setter
public Builder jumboFramesEnabled(Boolean jumboFramesEnabled) {
this.jumboFramesEnabled = Objects.requireNonNull(jumboFramesEnabled);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder macAddress(List macAddress) {
this.macAddress = Objects.requireNonNull(macAddress);
return this;
}
public Builder macAddress(String... macAddress) {
return macAddress(List.of(macAddress));
}
@CustomType.Setter
public Builder mountPoints(List mountPoints) {
this.mountPoints = Objects.requireNonNull(mountPoints);
return this;
}
public Builder mountPoints(NetworkMountPointResponse... mountPoints) {
return mountPoints(List.of(mountPoints));
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder pod(String pod) {
this.pod = Objects.requireNonNull(pod);
return this;
}
@CustomType.Setter
public Builder reservations(List reservations) {
this.reservations = Objects.requireNonNull(reservations);
return this;
}
public Builder reservations(NetworkAddressReservationResponse... reservations) {
return reservations(List.of(reservations));
}
@CustomType.Setter
public Builder servicesCidr(String servicesCidr) {
this.servicesCidr = Objects.requireNonNull(servicesCidr);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder type(String type) {
this.type = Objects.requireNonNull(type);
return this;
}
@CustomType.Setter
public Builder vlanId(String vlanId) {
this.vlanId = Objects.requireNonNull(vlanId);
return this;
}
@CustomType.Setter
public Builder vrf(VRFResponse vrf) {
this.vrf = Objects.requireNonNull(vrf);
return this;
}
public NetworkResponse build() {
final var o = new NetworkResponse();
o.cidr = cidr;
o.gatewayIp = gatewayIp;
o.ipAddress = ipAddress;
o.jumboFramesEnabled = jumboFramesEnabled;
o.labels = labels;
o.macAddress = macAddress;
o.mountPoints = mountPoints;
o.name = name;
o.pod = pod;
o.reservations = reservations;
o.servicesCidr = servicesCidr;
o.state = state;
o.type = type;
o.vlanId = vlanId;
o.vrf = vrf;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy