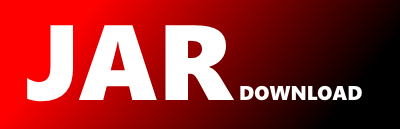
com.pulumi.googlenative.baremetalsolution.v2.outputs.VolumeResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.baremetalsolution.v2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.baremetalsolution.v2.outputs.SnapshotReservationDetailResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class VolumeResponse {
/**
* @return Is the Volume attached at at least one instance. This field is a lightweight counterpart of `instances` field. It is filled in List responses as well.
*
*/
private Boolean attached;
/**
* @return The size, in GiB, that this storage volume has expanded as a result of an auto grow policy. In the absence of auto-grow, the value is 0.
*
*/
private String autoGrownSizeGib;
/**
* @return Whether this volume is a boot volume. A boot volume is one which contains a boot LUN.
*
*/
private Boolean bootVolume;
/**
* @return The current size of this storage volume, in GiB, including space reserved for snapshots. This size might be different than the requested size if the storage volume has been configured with auto grow or auto shrink.
*
*/
private String currentSizeGib;
/**
* @return Additional emergency size that was requested for this Volume, in GiB. current_size_gib includes this value.
*
*/
private String emergencySizeGib;
/**
* @return Time after which volume will be fully deleted. It is filled only for volumes in COOLOFF state.
*
*/
private String expireTime;
/**
* @return Instances this Volume is attached to. This field is set only in Get requests.
*
*/
private List instances;
/**
* @return Labels as key value pairs.
*
*/
private Map labels;
/**
* @return Maximum size volume can be expanded to in case of evergency, in GiB.
*
*/
private String maxSizeGib;
/**
* @return The resource name of this `Volume`. Resource names are schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. Format: `projects/{project}/locations/{location}/volumes/{volume}`
*
*/
private String name;
/**
* @return Input only. User-specified notes for new Volume. Used to provision Volumes that require manual intervention.
*
*/
private String notes;
/**
* @return Originally requested size, in GiB.
*
*/
private String originallyRequestedSizeGib;
/**
* @return Immutable. Performance tier of the Volume. Default is SHARED.
*
*/
private String performanceTier;
/**
* @return Immutable. Pod name.
*
*/
private String pod;
/**
* @return Storage protocol for the Volume.
*
*/
private String protocol;
/**
* @return The space remaining in the storage volume for new LUNs, in GiB, excluding space reserved for snapshots.
*
*/
private String remainingSpaceGib;
/**
* @return The requested size of this storage volume, in GiB.
*
*/
private String requestedSizeGib;
/**
* @return The behavior to use when snapshot reserved space is full.
*
*/
private String snapshotAutoDeleteBehavior;
/**
* @return Whether snapshots are enabled.
*
*/
private Boolean snapshotEnabled;
/**
* @return Details about snapshot space reservation and usage on the storage volume.
*
*/
private SnapshotReservationDetailResponse snapshotReservationDetail;
/**
* @return The name of the snapshot schedule policy in use for this volume, if any.
*
*/
private String snapshotSchedulePolicy;
/**
* @return The state of this storage volume.
*
*/
private String state;
/**
* @return Input only. Name of the storage aggregate pool to allocate the volume in. Can be used only for VOLUME_PERFORMANCE_TIER_ASSIGNED volumes.
*
*/
private String storageAggregatePool;
/**
* @return The storage type for this volume.
*
*/
private String storageType;
/**
* @return The workload profile for the volume.
*
*/
private String workloadProfile;
private VolumeResponse() {}
/**
* @return Is the Volume attached at at least one instance. This field is a lightweight counterpart of `instances` field. It is filled in List responses as well.
*
*/
public Boolean attached() {
return this.attached;
}
/**
* @return The size, in GiB, that this storage volume has expanded as a result of an auto grow policy. In the absence of auto-grow, the value is 0.
*
*/
public String autoGrownSizeGib() {
return this.autoGrownSizeGib;
}
/**
* @return Whether this volume is a boot volume. A boot volume is one which contains a boot LUN.
*
*/
public Boolean bootVolume() {
return this.bootVolume;
}
/**
* @return The current size of this storage volume, in GiB, including space reserved for snapshots. This size might be different than the requested size if the storage volume has been configured with auto grow or auto shrink.
*
*/
public String currentSizeGib() {
return this.currentSizeGib;
}
/**
* @return Additional emergency size that was requested for this Volume, in GiB. current_size_gib includes this value.
*
*/
public String emergencySizeGib() {
return this.emergencySizeGib;
}
/**
* @return Time after which volume will be fully deleted. It is filled only for volumes in COOLOFF state.
*
*/
public String expireTime() {
return this.expireTime;
}
/**
* @return Instances this Volume is attached to. This field is set only in Get requests.
*
*/
public List instances() {
return this.instances;
}
/**
* @return Labels as key value pairs.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return Maximum size volume can be expanded to in case of evergency, in GiB.
*
*/
public String maxSizeGib() {
return this.maxSizeGib;
}
/**
* @return The resource name of this `Volume`. Resource names are schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. Format: `projects/{project}/locations/{location}/volumes/{volume}`
*
*/
public String name() {
return this.name;
}
/**
* @return Input only. User-specified notes for new Volume. Used to provision Volumes that require manual intervention.
*
*/
public String notes() {
return this.notes;
}
/**
* @return Originally requested size, in GiB.
*
*/
public String originallyRequestedSizeGib() {
return this.originallyRequestedSizeGib;
}
/**
* @return Immutable. Performance tier of the Volume. Default is SHARED.
*
*/
public String performanceTier() {
return this.performanceTier;
}
/**
* @return Immutable. Pod name.
*
*/
public String pod() {
return this.pod;
}
/**
* @return Storage protocol for the Volume.
*
*/
public String protocol() {
return this.protocol;
}
/**
* @return The space remaining in the storage volume for new LUNs, in GiB, excluding space reserved for snapshots.
*
*/
public String remainingSpaceGib() {
return this.remainingSpaceGib;
}
/**
* @return The requested size of this storage volume, in GiB.
*
*/
public String requestedSizeGib() {
return this.requestedSizeGib;
}
/**
* @return The behavior to use when snapshot reserved space is full.
*
*/
public String snapshotAutoDeleteBehavior() {
return this.snapshotAutoDeleteBehavior;
}
/**
* @return Whether snapshots are enabled.
*
*/
public Boolean snapshotEnabled() {
return this.snapshotEnabled;
}
/**
* @return Details about snapshot space reservation and usage on the storage volume.
*
*/
public SnapshotReservationDetailResponse snapshotReservationDetail() {
return this.snapshotReservationDetail;
}
/**
* @return The name of the snapshot schedule policy in use for this volume, if any.
*
*/
public String snapshotSchedulePolicy() {
return this.snapshotSchedulePolicy;
}
/**
* @return The state of this storage volume.
*
*/
public String state() {
return this.state;
}
/**
* @return Input only. Name of the storage aggregate pool to allocate the volume in. Can be used only for VOLUME_PERFORMANCE_TIER_ASSIGNED volumes.
*
*/
public String storageAggregatePool() {
return this.storageAggregatePool;
}
/**
* @return The storage type for this volume.
*
*/
public String storageType() {
return this.storageType;
}
/**
* @return The workload profile for the volume.
*
*/
public String workloadProfile() {
return this.workloadProfile;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VolumeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean attached;
private String autoGrownSizeGib;
private Boolean bootVolume;
private String currentSizeGib;
private String emergencySizeGib;
private String expireTime;
private List instances;
private Map labels;
private String maxSizeGib;
private String name;
private String notes;
private String originallyRequestedSizeGib;
private String performanceTier;
private String pod;
private String protocol;
private String remainingSpaceGib;
private String requestedSizeGib;
private String snapshotAutoDeleteBehavior;
private Boolean snapshotEnabled;
private SnapshotReservationDetailResponse snapshotReservationDetail;
private String snapshotSchedulePolicy;
private String state;
private String storageAggregatePool;
private String storageType;
private String workloadProfile;
public Builder() {}
public Builder(VolumeResponse defaults) {
Objects.requireNonNull(defaults);
this.attached = defaults.attached;
this.autoGrownSizeGib = defaults.autoGrownSizeGib;
this.bootVolume = defaults.bootVolume;
this.currentSizeGib = defaults.currentSizeGib;
this.emergencySizeGib = defaults.emergencySizeGib;
this.expireTime = defaults.expireTime;
this.instances = defaults.instances;
this.labels = defaults.labels;
this.maxSizeGib = defaults.maxSizeGib;
this.name = defaults.name;
this.notes = defaults.notes;
this.originallyRequestedSizeGib = defaults.originallyRequestedSizeGib;
this.performanceTier = defaults.performanceTier;
this.pod = defaults.pod;
this.protocol = defaults.protocol;
this.remainingSpaceGib = defaults.remainingSpaceGib;
this.requestedSizeGib = defaults.requestedSizeGib;
this.snapshotAutoDeleteBehavior = defaults.snapshotAutoDeleteBehavior;
this.snapshotEnabled = defaults.snapshotEnabled;
this.snapshotReservationDetail = defaults.snapshotReservationDetail;
this.snapshotSchedulePolicy = defaults.snapshotSchedulePolicy;
this.state = defaults.state;
this.storageAggregatePool = defaults.storageAggregatePool;
this.storageType = defaults.storageType;
this.workloadProfile = defaults.workloadProfile;
}
@CustomType.Setter
public Builder attached(Boolean attached) {
this.attached = Objects.requireNonNull(attached);
return this;
}
@CustomType.Setter
public Builder autoGrownSizeGib(String autoGrownSizeGib) {
this.autoGrownSizeGib = Objects.requireNonNull(autoGrownSizeGib);
return this;
}
@CustomType.Setter
public Builder bootVolume(Boolean bootVolume) {
this.bootVolume = Objects.requireNonNull(bootVolume);
return this;
}
@CustomType.Setter
public Builder currentSizeGib(String currentSizeGib) {
this.currentSizeGib = Objects.requireNonNull(currentSizeGib);
return this;
}
@CustomType.Setter
public Builder emergencySizeGib(String emergencySizeGib) {
this.emergencySizeGib = Objects.requireNonNull(emergencySizeGib);
return this;
}
@CustomType.Setter
public Builder expireTime(String expireTime) {
this.expireTime = Objects.requireNonNull(expireTime);
return this;
}
@CustomType.Setter
public Builder instances(List instances) {
this.instances = Objects.requireNonNull(instances);
return this;
}
public Builder instances(String... instances) {
return instances(List.of(instances));
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder maxSizeGib(String maxSizeGib) {
this.maxSizeGib = Objects.requireNonNull(maxSizeGib);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder notes(String notes) {
this.notes = Objects.requireNonNull(notes);
return this;
}
@CustomType.Setter
public Builder originallyRequestedSizeGib(String originallyRequestedSizeGib) {
this.originallyRequestedSizeGib = Objects.requireNonNull(originallyRequestedSizeGib);
return this;
}
@CustomType.Setter
public Builder performanceTier(String performanceTier) {
this.performanceTier = Objects.requireNonNull(performanceTier);
return this;
}
@CustomType.Setter
public Builder pod(String pod) {
this.pod = Objects.requireNonNull(pod);
return this;
}
@CustomType.Setter
public Builder protocol(String protocol) {
this.protocol = Objects.requireNonNull(protocol);
return this;
}
@CustomType.Setter
public Builder remainingSpaceGib(String remainingSpaceGib) {
this.remainingSpaceGib = Objects.requireNonNull(remainingSpaceGib);
return this;
}
@CustomType.Setter
public Builder requestedSizeGib(String requestedSizeGib) {
this.requestedSizeGib = Objects.requireNonNull(requestedSizeGib);
return this;
}
@CustomType.Setter
public Builder snapshotAutoDeleteBehavior(String snapshotAutoDeleteBehavior) {
this.snapshotAutoDeleteBehavior = Objects.requireNonNull(snapshotAutoDeleteBehavior);
return this;
}
@CustomType.Setter
public Builder snapshotEnabled(Boolean snapshotEnabled) {
this.snapshotEnabled = Objects.requireNonNull(snapshotEnabled);
return this;
}
@CustomType.Setter
public Builder snapshotReservationDetail(SnapshotReservationDetailResponse snapshotReservationDetail) {
this.snapshotReservationDetail = Objects.requireNonNull(snapshotReservationDetail);
return this;
}
@CustomType.Setter
public Builder snapshotSchedulePolicy(String snapshotSchedulePolicy) {
this.snapshotSchedulePolicy = Objects.requireNonNull(snapshotSchedulePolicy);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder storageAggregatePool(String storageAggregatePool) {
this.storageAggregatePool = Objects.requireNonNull(storageAggregatePool);
return this;
}
@CustomType.Setter
public Builder storageType(String storageType) {
this.storageType = Objects.requireNonNull(storageType);
return this;
}
@CustomType.Setter
public Builder workloadProfile(String workloadProfile) {
this.workloadProfile = Objects.requireNonNull(workloadProfile);
return this;
}
public VolumeResponse build() {
final var o = new VolumeResponse();
o.attached = attached;
o.autoGrownSizeGib = autoGrownSizeGib;
o.bootVolume = bootVolume;
o.currentSizeGib = currentSizeGib;
o.emergencySizeGib = emergencySizeGib;
o.expireTime = expireTime;
o.instances = instances;
o.labels = labels;
o.maxSizeGib = maxSizeGib;
o.name = name;
o.notes = notes;
o.originallyRequestedSizeGib = originallyRequestedSizeGib;
o.performanceTier = performanceTier;
o.pod = pod;
o.protocol = protocol;
o.remainingSpaceGib = remainingSpaceGib;
o.requestedSizeGib = requestedSizeGib;
o.snapshotAutoDeleteBehavior = snapshotAutoDeleteBehavior;
o.snapshotEnabled = snapshotEnabled;
o.snapshotReservationDetail = snapshotReservationDetail;
o.snapshotSchedulePolicy = snapshotSchedulePolicy;
o.state = state;
o.storageAggregatePool = storageAggregatePool;
o.storageType = storageType;
o.workloadProfile = workloadProfile;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy