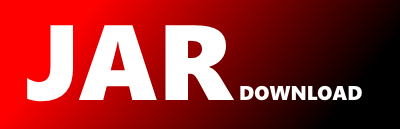
com.pulumi.googlenative.bigqueryreservation.v1.CapacityCommitmentArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.bigqueryreservation.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.bigqueryreservation.v1.enums.CapacityCommitmentEdition;
import com.pulumi.googlenative.bigqueryreservation.v1.enums.CapacityCommitmentPlan;
import com.pulumi.googlenative.bigqueryreservation.v1.enums.CapacityCommitmentRenewalPlan;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CapacityCommitmentArgs extends com.pulumi.resources.ResourceArgs {
public static final CapacityCommitmentArgs Empty = new CapacityCommitmentArgs();
/**
* The optional capacity commitment ID. Capacity commitment name will be generated automatically if this field is empty. This field must only contain lower case alphanumeric characters or dashes. The first and last character cannot be a dash. Max length is 64 characters. NOTE: this ID won't be kept if the capacity commitment is split or merged.
*
*/
@Import(name="capacityCommitmentId")
private @Nullable Output capacityCommitmentId;
/**
* @return The optional capacity commitment ID. Capacity commitment name will be generated automatically if this field is empty. This field must only contain lower case alphanumeric characters or dashes. The first and last character cannot be a dash. Max length is 64 characters. NOTE: this ID won't be kept if the capacity commitment is split or merged.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy