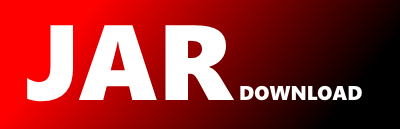
com.pulumi.googlenative.bigqueryreservation.v1.outputs.GetCapacityCommitmentResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.bigqueryreservation.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.bigqueryreservation.v1.outputs.StatusResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetCapacityCommitmentResult {
/**
* @return The end of the current commitment period. It is applicable only for ACTIVE capacity commitments.
*
*/
private String commitmentEndTime;
/**
* @return The start of the current commitment period. It is applicable only for ACTIVE capacity commitments.
*
*/
private String commitmentStartTime;
/**
* @return Edition of the capacity commitment.
*
*/
private String edition;
/**
* @return For FAILED commitment plan, provides the reason of failure.
*
*/
private StatusResponse failureStatus;
/**
* @return Applicable only for commitments located within one of the BigQuery multi-regions (US or EU). If set to true, this commitment is placed in the organization's secondary region which is designated for disaster recovery purposes. If false, this commitment is placed in the organization's default region. NOTE: this is a preview feature. Project must be allow-listed in order to set this field.
*
*/
private Boolean multiRegionAuxiliary;
/**
* @return The resource name of the capacity commitment, e.g., `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only contain lower case alphanumeric characters or dashes. It must start with a letter and must not end with a dash. Its maximum length is 64 characters.
*
*/
private String name;
/**
* @return Capacity commitment commitment plan.
*
*/
private String plan;
/**
* @return The plan this capacity commitment is converted to after commitment_end_time passes. Once the plan is changed, committed period is extended according to commitment plan. Only applicable for ANNUAL and TRIAL commitments.
*
*/
private String renewalPlan;
/**
* @return Number of slots in this commitment.
*
*/
private String slotCount;
/**
* @return State of the commitment.
*
*/
private String state;
private GetCapacityCommitmentResult() {}
/**
* @return The end of the current commitment period. It is applicable only for ACTIVE capacity commitments.
*
*/
public String commitmentEndTime() {
return this.commitmentEndTime;
}
/**
* @return The start of the current commitment period. It is applicable only for ACTIVE capacity commitments.
*
*/
public String commitmentStartTime() {
return this.commitmentStartTime;
}
/**
* @return Edition of the capacity commitment.
*
*/
public String edition() {
return this.edition;
}
/**
* @return For FAILED commitment plan, provides the reason of failure.
*
*/
public StatusResponse failureStatus() {
return this.failureStatus;
}
/**
* @return Applicable only for commitments located within one of the BigQuery multi-regions (US or EU). If set to true, this commitment is placed in the organization's secondary region which is designated for disaster recovery purposes. If false, this commitment is placed in the organization's default region. NOTE: this is a preview feature. Project must be allow-listed in order to set this field.
*
*/
public Boolean multiRegionAuxiliary() {
return this.multiRegionAuxiliary;
}
/**
* @return The resource name of the capacity commitment, e.g., `projects/myproject/locations/US/capacityCommitments/123` The commitment_id must only contain lower case alphanumeric characters or dashes. It must start with a letter and must not end with a dash. Its maximum length is 64 characters.
*
*/
public String name() {
return this.name;
}
/**
* @return Capacity commitment commitment plan.
*
*/
public String plan() {
return this.plan;
}
/**
* @return The plan this capacity commitment is converted to after commitment_end_time passes. Once the plan is changed, committed period is extended according to commitment plan. Only applicable for ANNUAL and TRIAL commitments.
*
*/
public String renewalPlan() {
return this.renewalPlan;
}
/**
* @return Number of slots in this commitment.
*
*/
public String slotCount() {
return this.slotCount;
}
/**
* @return State of the commitment.
*
*/
public String state() {
return this.state;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCapacityCommitmentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String commitmentEndTime;
private String commitmentStartTime;
private String edition;
private StatusResponse failureStatus;
private Boolean multiRegionAuxiliary;
private String name;
private String plan;
private String renewalPlan;
private String slotCount;
private String state;
public Builder() {}
public Builder(GetCapacityCommitmentResult defaults) {
Objects.requireNonNull(defaults);
this.commitmentEndTime = defaults.commitmentEndTime;
this.commitmentStartTime = defaults.commitmentStartTime;
this.edition = defaults.edition;
this.failureStatus = defaults.failureStatus;
this.multiRegionAuxiliary = defaults.multiRegionAuxiliary;
this.name = defaults.name;
this.plan = defaults.plan;
this.renewalPlan = defaults.renewalPlan;
this.slotCount = defaults.slotCount;
this.state = defaults.state;
}
@CustomType.Setter
public Builder commitmentEndTime(String commitmentEndTime) {
this.commitmentEndTime = Objects.requireNonNull(commitmentEndTime);
return this;
}
@CustomType.Setter
public Builder commitmentStartTime(String commitmentStartTime) {
this.commitmentStartTime = Objects.requireNonNull(commitmentStartTime);
return this;
}
@CustomType.Setter
public Builder edition(String edition) {
this.edition = Objects.requireNonNull(edition);
return this;
}
@CustomType.Setter
public Builder failureStatus(StatusResponse failureStatus) {
this.failureStatus = Objects.requireNonNull(failureStatus);
return this;
}
@CustomType.Setter
public Builder multiRegionAuxiliary(Boolean multiRegionAuxiliary) {
this.multiRegionAuxiliary = Objects.requireNonNull(multiRegionAuxiliary);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder plan(String plan) {
this.plan = Objects.requireNonNull(plan);
return this;
}
@CustomType.Setter
public Builder renewalPlan(String renewalPlan) {
this.renewalPlan = Objects.requireNonNull(renewalPlan);
return this;
}
@CustomType.Setter
public Builder slotCount(String slotCount) {
this.slotCount = Objects.requireNonNull(slotCount);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
public GetCapacityCommitmentResult build() {
final var o = new GetCapacityCommitmentResult();
o.commitmentEndTime = commitmentEndTime;
o.commitmentStartTime = commitmentStartTime;
o.edition = edition;
o.failureStatus = failureStatus;
o.multiRegionAuxiliary = multiRegionAuxiliary;
o.name = name;
o.plan = plan;
o.renewalPlan = renewalPlan;
o.slotCount = slotCount;
o.state = state;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy