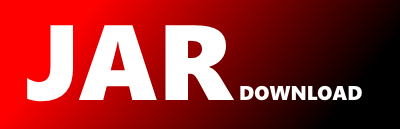
com.pulumi.googlenative.bigqueryreservation.v1beta1.ReservationArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.bigqueryreservation.v1beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ReservationArgs extends com.pulumi.resources.ResourceArgs {
public static final ReservationArgs Empty = new ReservationArgs();
/**
* Maximum number of queries that are allowed to run concurrently in this reservation. This is a soft limit due to asynchronous nature of the system and various optimizations for small queries. Default value is 0 which means that concurrency will be automatically set based on the reservation size.
*
*/
@Import(name="concurrency")
private @Nullable Output concurrency;
/**
* @return Maximum number of queries that are allowed to run concurrently in this reservation. This is a soft limit due to asynchronous nature of the system and various optimizations for small queries. Default value is 0 which means that concurrency will be automatically set based on the reservation size.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy