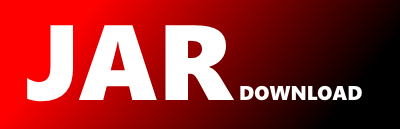
com.pulumi.googlenative.billingbudgets.v1.outputs.GetBudgetResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.billingbudgets.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.billingbudgets.v1.outputs.GoogleCloudBillingBudgetsV1BudgetAmountResponse;
import com.pulumi.googlenative.billingbudgets.v1.outputs.GoogleCloudBillingBudgetsV1FilterResponse;
import com.pulumi.googlenative.billingbudgets.v1.outputs.GoogleCloudBillingBudgetsV1NotificationsRuleResponse;
import com.pulumi.googlenative.billingbudgets.v1.outputs.GoogleCloudBillingBudgetsV1ThresholdRuleResponse;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetBudgetResult {
/**
* @return Budgeted amount.
*
*/
private GoogleCloudBillingBudgetsV1BudgetAmountResponse amount;
/**
* @return Optional. Filters that define which resources are used to compute the actual spend against the budget amount, such as projects, services, and the budget's time period, as well as other filters.
*
*/
private GoogleCloudBillingBudgetsV1FilterResponse budgetFilter;
/**
* @return User data for display name in UI. The name must be less than or equal to 60 characters.
*
*/
private String displayName;
/**
* @return Optional. Etag to validate that the object is unchanged for a read-modify-write operation. An empty etag causes an update to overwrite other changes.
*
*/
private String etag;
/**
* @return Resource name of the budget. The resource name implies the scope of a budget. Values are of the form `billingAccounts/{billingAccountId}/budgets/{budgetId}`.
*
*/
private String name;
/**
* @return Optional. Rules to apply to notifications sent based on budget spend and thresholds.
*
*/
private GoogleCloudBillingBudgetsV1NotificationsRuleResponse notificationsRule;
/**
* @return Optional. Rules that trigger alerts (notifications of thresholds being crossed) when spend exceeds the specified percentages of the budget. Optional for `pubsubTopic` notifications. Required if using email notifications.
*
*/
private List thresholdRules;
private GetBudgetResult() {}
/**
* @return Budgeted amount.
*
*/
public GoogleCloudBillingBudgetsV1BudgetAmountResponse amount() {
return this.amount;
}
/**
* @return Optional. Filters that define which resources are used to compute the actual spend against the budget amount, such as projects, services, and the budget's time period, as well as other filters.
*
*/
public GoogleCloudBillingBudgetsV1FilterResponse budgetFilter() {
return this.budgetFilter;
}
/**
* @return User data for display name in UI. The name must be less than or equal to 60 characters.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Optional. Etag to validate that the object is unchanged for a read-modify-write operation. An empty etag causes an update to overwrite other changes.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource name of the budget. The resource name implies the scope of a budget. Values are of the form `billingAccounts/{billingAccountId}/budgets/{budgetId}`.
*
*/
public String name() {
return this.name;
}
/**
* @return Optional. Rules to apply to notifications sent based on budget spend and thresholds.
*
*/
public GoogleCloudBillingBudgetsV1NotificationsRuleResponse notificationsRule() {
return this.notificationsRule;
}
/**
* @return Optional. Rules that trigger alerts (notifications of thresholds being crossed) when spend exceeds the specified percentages of the budget. Optional for `pubsubTopic` notifications. Required if using email notifications.
*
*/
public List thresholdRules() {
return this.thresholdRules;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBudgetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private GoogleCloudBillingBudgetsV1BudgetAmountResponse amount;
private GoogleCloudBillingBudgetsV1FilterResponse budgetFilter;
private String displayName;
private String etag;
private String name;
private GoogleCloudBillingBudgetsV1NotificationsRuleResponse notificationsRule;
private List thresholdRules;
public Builder() {}
public Builder(GetBudgetResult defaults) {
Objects.requireNonNull(defaults);
this.amount = defaults.amount;
this.budgetFilter = defaults.budgetFilter;
this.displayName = defaults.displayName;
this.etag = defaults.etag;
this.name = defaults.name;
this.notificationsRule = defaults.notificationsRule;
this.thresholdRules = defaults.thresholdRules;
}
@CustomType.Setter
public Builder amount(GoogleCloudBillingBudgetsV1BudgetAmountResponse amount) {
this.amount = Objects.requireNonNull(amount);
return this;
}
@CustomType.Setter
public Builder budgetFilter(GoogleCloudBillingBudgetsV1FilterResponse budgetFilter) {
this.budgetFilter = Objects.requireNonNull(budgetFilter);
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
this.displayName = Objects.requireNonNull(displayName);
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
this.etag = Objects.requireNonNull(etag);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder notificationsRule(GoogleCloudBillingBudgetsV1NotificationsRuleResponse notificationsRule) {
this.notificationsRule = Objects.requireNonNull(notificationsRule);
return this;
}
@CustomType.Setter
public Builder thresholdRules(List thresholdRules) {
this.thresholdRules = Objects.requireNonNull(thresholdRules);
return this;
}
public Builder thresholdRules(GoogleCloudBillingBudgetsV1ThresholdRuleResponse... thresholdRules) {
return thresholdRules(List.of(thresholdRules));
}
public GetBudgetResult build() {
final var o = new GetBudgetResult();
o.amount = amount;
o.budgetFilter = budgetFilter;
o.displayName = displayName;
o.etag = etag;
o.name = name;
o.notificationsRule = notificationsRule;
o.thresholdRules = thresholdRules;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy