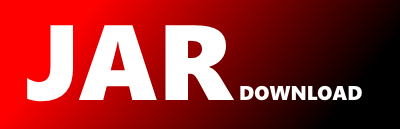
com.pulumi.googlenative.cloudbuild.v1.inputs.GitFileSourceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.cloudbuild.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.cloudbuild.v1.enums.GitFileSourceRepoType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* GitFileSource describes a file within a (possibly remote) code repository.
*
*/
public final class GitFileSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final GitFileSourceArgs Empty = new GitFileSourceArgs();
/**
* The full resource name of the bitbucket server config. Format: `projects/{project}/locations/{location}/bitbucketServerConfigs/{id}`.
*
*/
@Import(name="bitbucketServerConfig")
private @Nullable Output bitbucketServerConfig;
/**
* @return The full resource name of the bitbucket server config. Format: `projects/{project}/locations/{location}/bitbucketServerConfigs/{id}`.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy