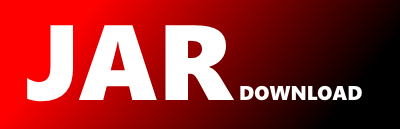
com.pulumi.googlenative.clouddeploy.v1.outputs.GetReleaseResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.clouddeploy.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.clouddeploy.v1.outputs.BuildArtifactResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.DeliveryPipelineResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.ReleaseConditionResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.TargetResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetReleaseResult {
/**
* @return Indicates whether this is an abandoned release.
*
*/
private Boolean abandoned;
/**
* @return User annotations. These attributes can only be set and used by the user, and not by Google Cloud Deploy. See https://google.aip.dev/128#annotations for more details such as format and size limitations.
*
*/
private Map annotations;
/**
* @return List of artifacts to pass through to Skaffold command.
*
*/
private List buildArtifacts;
/**
* @return Information around the state of the Release.
*
*/
private ReleaseConditionResponse condition;
/**
* @return Time at which the `Release` was created.
*
*/
private String createTime;
/**
* @return Snapshot of the parent pipeline taken at release creation time.
*
*/
private DeliveryPipelineResponse deliveryPipelineSnapshot;
/**
* @return Description of the `Release`. Max length is 255 characters.
*
*/
private String description;
/**
* @return This checksum is computed by the server based on the value of other fields, and may be sent on update and delete requests to ensure the client has an up-to-date value before proceeding.
*
*/
private String etag;
/**
* @return Labels are attributes that can be set and used by both the user and by Google Cloud Deploy. Labels must meet the following constraints: * Keys and values can contain only lowercase letters, numeric characters, underscores, and dashes. * All characters must use UTF-8 encoding, and international characters are allowed. * Keys must start with a lowercase letter or international character. * Each resource is limited to a maximum of 64 labels. Both keys and values are additionally constrained to be <= 128 bytes.
*
*/
private Map labels;
/**
* @return Optional. Name of the `Release`. Format is projects/{project}/ locations/{location}/deliveryPipelines/{deliveryPipeline}/ releases/a-z{0,62}.
*
*/
private String name;
/**
* @return Time at which the render completed.
*
*/
private String renderEndTime;
/**
* @return Time at which the render began.
*
*/
private String renderStartTime;
/**
* @return Current state of the render operation.
*
*/
private String renderState;
/**
* @return Filepath of the Skaffold config inside of the config URI.
*
*/
private String skaffoldConfigPath;
/**
* @return Cloud Storage URI of tar.gz archive containing Skaffold configuration.
*
*/
private String skaffoldConfigUri;
/**
* @return The Skaffold version to use when operating on this release, such as "1.20.0". Not all versions are valid; Google Cloud Deploy supports a specific set of versions. If unset, the most recent supported Skaffold version will be used.
*
*/
private String skaffoldVersion;
/**
* @return Map from target ID to the target artifacts created during the render operation.
*
*/
private Map targetArtifacts;
/**
* @return Map from target ID to details of the render operation for that target.
*
*/
private Map targetRenders;
/**
* @return Snapshot of the targets taken at release creation time.
*
*/
private List targetSnapshots;
/**
* @return Unique identifier of the `Release`.
*
*/
private String uid;
private GetReleaseResult() {}
/**
* @return Indicates whether this is an abandoned release.
*
*/
public Boolean abandoned() {
return this.abandoned;
}
/**
* @return User annotations. These attributes can only be set and used by the user, and not by Google Cloud Deploy. See https://google.aip.dev/128#annotations for more details such as format and size limitations.
*
*/
public Map annotations() {
return this.annotations;
}
/**
* @return List of artifacts to pass through to Skaffold command.
*
*/
public List buildArtifacts() {
return this.buildArtifacts;
}
/**
* @return Information around the state of the Release.
*
*/
public ReleaseConditionResponse condition() {
return this.condition;
}
/**
* @return Time at which the `Release` was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return Snapshot of the parent pipeline taken at release creation time.
*
*/
public DeliveryPipelineResponse deliveryPipelineSnapshot() {
return this.deliveryPipelineSnapshot;
}
/**
* @return Description of the `Release`. Max length is 255 characters.
*
*/
public String description() {
return this.description;
}
/**
* @return This checksum is computed by the server based on the value of other fields, and may be sent on update and delete requests to ensure the client has an up-to-date value before proceeding.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Labels are attributes that can be set and used by both the user and by Google Cloud Deploy. Labels must meet the following constraints: * Keys and values can contain only lowercase letters, numeric characters, underscores, and dashes. * All characters must use UTF-8 encoding, and international characters are allowed. * Keys must start with a lowercase letter or international character. * Each resource is limited to a maximum of 64 labels. Both keys and values are additionally constrained to be <= 128 bytes.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return Optional. Name of the `Release`. Format is projects/{project}/ locations/{location}/deliveryPipelines/{deliveryPipeline}/ releases/a-z{0,62}.
*
*/
public String name() {
return this.name;
}
/**
* @return Time at which the render completed.
*
*/
public String renderEndTime() {
return this.renderEndTime;
}
/**
* @return Time at which the render began.
*
*/
public String renderStartTime() {
return this.renderStartTime;
}
/**
* @return Current state of the render operation.
*
*/
public String renderState() {
return this.renderState;
}
/**
* @return Filepath of the Skaffold config inside of the config URI.
*
*/
public String skaffoldConfigPath() {
return this.skaffoldConfigPath;
}
/**
* @return Cloud Storage URI of tar.gz archive containing Skaffold configuration.
*
*/
public String skaffoldConfigUri() {
return this.skaffoldConfigUri;
}
/**
* @return The Skaffold version to use when operating on this release, such as "1.20.0". Not all versions are valid; Google Cloud Deploy supports a specific set of versions. If unset, the most recent supported Skaffold version will be used.
*
*/
public String skaffoldVersion() {
return this.skaffoldVersion;
}
/**
* @return Map from target ID to the target artifacts created during the render operation.
*
*/
public Map targetArtifacts() {
return this.targetArtifacts;
}
/**
* @return Map from target ID to details of the render operation for that target.
*
*/
public Map targetRenders() {
return this.targetRenders;
}
/**
* @return Snapshot of the targets taken at release creation time.
*
*/
public List targetSnapshots() {
return this.targetSnapshots;
}
/**
* @return Unique identifier of the `Release`.
*
*/
public String uid() {
return this.uid;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReleaseResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean abandoned;
private Map annotations;
private List buildArtifacts;
private ReleaseConditionResponse condition;
private String createTime;
private DeliveryPipelineResponse deliveryPipelineSnapshot;
private String description;
private String etag;
private Map labels;
private String name;
private String renderEndTime;
private String renderStartTime;
private String renderState;
private String skaffoldConfigPath;
private String skaffoldConfigUri;
private String skaffoldVersion;
private Map targetArtifacts;
private Map targetRenders;
private List targetSnapshots;
private String uid;
public Builder() {}
public Builder(GetReleaseResult defaults) {
Objects.requireNonNull(defaults);
this.abandoned = defaults.abandoned;
this.annotations = defaults.annotations;
this.buildArtifacts = defaults.buildArtifacts;
this.condition = defaults.condition;
this.createTime = defaults.createTime;
this.deliveryPipelineSnapshot = defaults.deliveryPipelineSnapshot;
this.description = defaults.description;
this.etag = defaults.etag;
this.labels = defaults.labels;
this.name = defaults.name;
this.renderEndTime = defaults.renderEndTime;
this.renderStartTime = defaults.renderStartTime;
this.renderState = defaults.renderState;
this.skaffoldConfigPath = defaults.skaffoldConfigPath;
this.skaffoldConfigUri = defaults.skaffoldConfigUri;
this.skaffoldVersion = defaults.skaffoldVersion;
this.targetArtifacts = defaults.targetArtifacts;
this.targetRenders = defaults.targetRenders;
this.targetSnapshots = defaults.targetSnapshots;
this.uid = defaults.uid;
}
@CustomType.Setter
public Builder abandoned(Boolean abandoned) {
this.abandoned = Objects.requireNonNull(abandoned);
return this;
}
@CustomType.Setter
public Builder annotations(Map annotations) {
this.annotations = Objects.requireNonNull(annotations);
return this;
}
@CustomType.Setter
public Builder buildArtifacts(List buildArtifacts) {
this.buildArtifacts = Objects.requireNonNull(buildArtifacts);
return this;
}
public Builder buildArtifacts(BuildArtifactResponse... buildArtifacts) {
return buildArtifacts(List.of(buildArtifacts));
}
@CustomType.Setter
public Builder condition(ReleaseConditionResponse condition) {
this.condition = Objects.requireNonNull(condition);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder deliveryPipelineSnapshot(DeliveryPipelineResponse deliveryPipelineSnapshot) {
this.deliveryPipelineSnapshot = Objects.requireNonNull(deliveryPipelineSnapshot);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
this.etag = Objects.requireNonNull(etag);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder renderEndTime(String renderEndTime) {
this.renderEndTime = Objects.requireNonNull(renderEndTime);
return this;
}
@CustomType.Setter
public Builder renderStartTime(String renderStartTime) {
this.renderStartTime = Objects.requireNonNull(renderStartTime);
return this;
}
@CustomType.Setter
public Builder renderState(String renderState) {
this.renderState = Objects.requireNonNull(renderState);
return this;
}
@CustomType.Setter
public Builder skaffoldConfigPath(String skaffoldConfigPath) {
this.skaffoldConfigPath = Objects.requireNonNull(skaffoldConfigPath);
return this;
}
@CustomType.Setter
public Builder skaffoldConfigUri(String skaffoldConfigUri) {
this.skaffoldConfigUri = Objects.requireNonNull(skaffoldConfigUri);
return this;
}
@CustomType.Setter
public Builder skaffoldVersion(String skaffoldVersion) {
this.skaffoldVersion = Objects.requireNonNull(skaffoldVersion);
return this;
}
@CustomType.Setter
public Builder targetArtifacts(Map targetArtifacts) {
this.targetArtifacts = Objects.requireNonNull(targetArtifacts);
return this;
}
@CustomType.Setter
public Builder targetRenders(Map targetRenders) {
this.targetRenders = Objects.requireNonNull(targetRenders);
return this;
}
@CustomType.Setter
public Builder targetSnapshots(List targetSnapshots) {
this.targetSnapshots = Objects.requireNonNull(targetSnapshots);
return this;
}
public Builder targetSnapshots(TargetResponse... targetSnapshots) {
return targetSnapshots(List.of(targetSnapshots));
}
@CustomType.Setter
public Builder uid(String uid) {
this.uid = Objects.requireNonNull(uid);
return this;
}
public GetReleaseResult build() {
final var o = new GetReleaseResult();
o.abandoned = abandoned;
o.annotations = annotations;
o.buildArtifacts = buildArtifacts;
o.condition = condition;
o.createTime = createTime;
o.deliveryPipelineSnapshot = deliveryPipelineSnapshot;
o.description = description;
o.etag = etag;
o.labels = labels;
o.name = name;
o.renderEndTime = renderEndTime;
o.renderStartTime = renderStartTime;
o.renderState = renderState;
o.skaffoldConfigPath = skaffoldConfigPath;
o.skaffoldConfigUri = skaffoldConfigUri;
o.skaffoldVersion = skaffoldVersion;
o.targetArtifacts = targetArtifacts;
o.targetRenders = targetRenders;
o.targetSnapshots = targetSnapshots;
o.uid = uid;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy