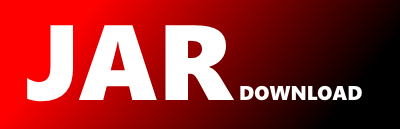
com.pulumi.googlenative.clouddeploy.v1.outputs.TargetResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.clouddeploy.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.clouddeploy.v1.outputs.AnthosClusterResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.CloudRunLocationResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.ExecutionConfigResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.GkeClusterResponse;
import com.pulumi.googlenative.clouddeploy.v1.outputs.MultiTargetResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class TargetResponse {
/**
* @return Optional. User annotations. These attributes can only be set and used by the user, and not by Google Cloud Deploy. See https://google.aip.dev/128#annotations for more details such as format and size limitations.
*
*/
private Map annotations;
/**
* @return Information specifying an Anthos Cluster.
*
*/
private AnthosClusterResponse anthosCluster;
/**
* @return Time at which the `Target` was created.
*
*/
private String createTime;
/**
* @return Optional. Description of the `Target`. Max length is 255 characters.
*
*/
private String description;
/**
* @return Optional. This checksum is computed by the server based on the value of other fields, and may be sent on update and delete requests to ensure the client has an up-to-date value before proceeding.
*
*/
private String etag;
/**
* @return Configurations for all execution that relates to this `Target`. Each `ExecutionEnvironmentUsage` value may only be used in a single configuration; using the same value multiple times is an error. When one or more configurations are specified, they must include the `RENDER` and `DEPLOY` `ExecutionEnvironmentUsage` values. When no configurations are specified, execution will use the default specified in `DefaultPool`.
*
*/
private List executionConfigs;
/**
* @return Information specifying a GKE Cluster.
*
*/
private GkeClusterResponse gke;
/**
* @return Optional. Labels are attributes that can be set and used by both the user and by Google Cloud Deploy. Labels must meet the following constraints: * Keys and values can contain only lowercase letters, numeric characters, underscores, and dashes. * All characters must use UTF-8 encoding, and international characters are allowed. * Keys must start with a lowercase letter or international character. * Each resource is limited to a maximum of 64 labels. Both keys and values are additionally constrained to be <= 128 bytes.
*
*/
private Map labels;
/**
* @return Information specifying a multiTarget.
*
*/
private MultiTargetResponse multiTarget;
/**
* @return Optional. Name of the `Target`. Format is projects/{project}/locations/{location}/targets/a-z{0,62}.
*
*/
private String name;
/**
* @return Optional. Whether or not the `Target` requires approval.
*
*/
private Boolean requireApproval;
/**
* @return Information specifying a Cloud Run deployment target.
*
*/
private CloudRunLocationResponse run;
/**
* @return Resource id of the `Target`.
*
*/
private String targetId;
/**
* @return Unique identifier of the `Target`.
*
*/
private String uid;
/**
* @return Most recent time at which the `Target` was updated.
*
*/
private String updateTime;
private TargetResponse() {}
/**
* @return Optional. User annotations. These attributes can only be set and used by the user, and not by Google Cloud Deploy. See https://google.aip.dev/128#annotations for more details such as format and size limitations.
*
*/
public Map annotations() {
return this.annotations;
}
/**
* @return Information specifying an Anthos Cluster.
*
*/
public AnthosClusterResponse anthosCluster() {
return this.anthosCluster;
}
/**
* @return Time at which the `Target` was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return Optional. Description of the `Target`. Max length is 255 characters.
*
*/
public String description() {
return this.description;
}
/**
* @return Optional. This checksum is computed by the server based on the value of other fields, and may be sent on update and delete requests to ensure the client has an up-to-date value before proceeding.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Configurations for all execution that relates to this `Target`. Each `ExecutionEnvironmentUsage` value may only be used in a single configuration; using the same value multiple times is an error. When one or more configurations are specified, they must include the `RENDER` and `DEPLOY` `ExecutionEnvironmentUsage` values. When no configurations are specified, execution will use the default specified in `DefaultPool`.
*
*/
public List executionConfigs() {
return this.executionConfigs;
}
/**
* @return Information specifying a GKE Cluster.
*
*/
public GkeClusterResponse gke() {
return this.gke;
}
/**
* @return Optional. Labels are attributes that can be set and used by both the user and by Google Cloud Deploy. Labels must meet the following constraints: * Keys and values can contain only lowercase letters, numeric characters, underscores, and dashes. * All characters must use UTF-8 encoding, and international characters are allowed. * Keys must start with a lowercase letter or international character. * Each resource is limited to a maximum of 64 labels. Both keys and values are additionally constrained to be <= 128 bytes.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return Information specifying a multiTarget.
*
*/
public MultiTargetResponse multiTarget() {
return this.multiTarget;
}
/**
* @return Optional. Name of the `Target`. Format is projects/{project}/locations/{location}/targets/a-z{0,62}.
*
*/
public String name() {
return this.name;
}
/**
* @return Optional. Whether or not the `Target` requires approval.
*
*/
public Boolean requireApproval() {
return this.requireApproval;
}
/**
* @return Information specifying a Cloud Run deployment target.
*
*/
public CloudRunLocationResponse run() {
return this.run;
}
/**
* @return Resource id of the `Target`.
*
*/
public String targetId() {
return this.targetId;
}
/**
* @return Unique identifier of the `Target`.
*
*/
public String uid() {
return this.uid;
}
/**
* @return Most recent time at which the `Target` was updated.
*
*/
public String updateTime() {
return this.updateTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TargetResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Map annotations;
private AnthosClusterResponse anthosCluster;
private String createTime;
private String description;
private String etag;
private List executionConfigs;
private GkeClusterResponse gke;
private Map labels;
private MultiTargetResponse multiTarget;
private String name;
private Boolean requireApproval;
private CloudRunLocationResponse run;
private String targetId;
private String uid;
private String updateTime;
public Builder() {}
public Builder(TargetResponse defaults) {
Objects.requireNonNull(defaults);
this.annotations = defaults.annotations;
this.anthosCluster = defaults.anthosCluster;
this.createTime = defaults.createTime;
this.description = defaults.description;
this.etag = defaults.etag;
this.executionConfigs = defaults.executionConfigs;
this.gke = defaults.gke;
this.labels = defaults.labels;
this.multiTarget = defaults.multiTarget;
this.name = defaults.name;
this.requireApproval = defaults.requireApproval;
this.run = defaults.run;
this.targetId = defaults.targetId;
this.uid = defaults.uid;
this.updateTime = defaults.updateTime;
}
@CustomType.Setter
public Builder annotations(Map annotations) {
this.annotations = Objects.requireNonNull(annotations);
return this;
}
@CustomType.Setter
public Builder anthosCluster(AnthosClusterResponse anthosCluster) {
this.anthosCluster = Objects.requireNonNull(anthosCluster);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
this.etag = Objects.requireNonNull(etag);
return this;
}
@CustomType.Setter
public Builder executionConfigs(List executionConfigs) {
this.executionConfigs = Objects.requireNonNull(executionConfigs);
return this;
}
public Builder executionConfigs(ExecutionConfigResponse... executionConfigs) {
return executionConfigs(List.of(executionConfigs));
}
@CustomType.Setter
public Builder gke(GkeClusterResponse gke) {
this.gke = Objects.requireNonNull(gke);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder multiTarget(MultiTargetResponse multiTarget) {
this.multiTarget = Objects.requireNonNull(multiTarget);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder requireApproval(Boolean requireApproval) {
this.requireApproval = Objects.requireNonNull(requireApproval);
return this;
}
@CustomType.Setter
public Builder run(CloudRunLocationResponse run) {
this.run = Objects.requireNonNull(run);
return this;
}
@CustomType.Setter
public Builder targetId(String targetId) {
this.targetId = Objects.requireNonNull(targetId);
return this;
}
@CustomType.Setter
public Builder uid(String uid) {
this.uid = Objects.requireNonNull(uid);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
public TargetResponse build() {
final var o = new TargetResponse();
o.annotations = annotations;
o.anthosCluster = anthosCluster;
o.createTime = createTime;
o.description = description;
o.etag = etag;
o.executionConfigs = executionConfigs;
o.gke = gke;
o.labels = labels;
o.multiTarget = multiTarget;
o.name = name;
o.requireApproval = requireApproval;
o.run = run;
o.targetId = targetId;
o.uid = uid;
o.updateTime = updateTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy