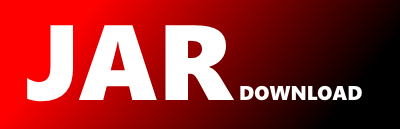
com.pulumi.googlenative.cloudfunctions.v1.outputs.SecretVolumeResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.cloudfunctions.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.cloudfunctions.v1.outputs.SecretVersionResponse;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class SecretVolumeResponse {
/**
* @return The path within the container to mount the secret volume. For example, setting the mount_path as `/etc/secrets` would mount the secret value files under the `/etc/secrets` directory. This directory will also be completely shadowed and unavailable to mount any other secrets. Recommended mount paths: /etc/secrets Restricted mount paths: /cloudsql, /dev/log, /pod, /proc, /var/log
*
*/
private String mountPath;
/**
* @return Project identifier (preferrably project number but can also be the project ID) of the project that contains the secret. If not set, it will be populated with the function's project assuming that the secret exists in the same project as of the function.
*
*/
private String project;
/**
* @return Name of the secret in secret manager (not the full resource name).
*
*/
private String secret;
/**
* @return List of secret versions to mount for this secret. If empty, the `latest` version of the secret will be made available in a file named after the secret under the mount point.
*
*/
private List versions;
private SecretVolumeResponse() {}
/**
* @return The path within the container to mount the secret volume. For example, setting the mount_path as `/etc/secrets` would mount the secret value files under the `/etc/secrets` directory. This directory will also be completely shadowed and unavailable to mount any other secrets. Recommended mount paths: /etc/secrets Restricted mount paths: /cloudsql, /dev/log, /pod, /proc, /var/log
*
*/
public String mountPath() {
return this.mountPath;
}
/**
* @return Project identifier (preferrably project number but can also be the project ID) of the project that contains the secret. If not set, it will be populated with the function's project assuming that the secret exists in the same project as of the function.
*
*/
public String project() {
return this.project;
}
/**
* @return Name of the secret in secret manager (not the full resource name).
*
*/
public String secret() {
return this.secret;
}
/**
* @return List of secret versions to mount for this secret. If empty, the `latest` version of the secret will be made available in a file named after the secret under the mount point.
*
*/
public List versions() {
return this.versions;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SecretVolumeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String mountPath;
private String project;
private String secret;
private List versions;
public Builder() {}
public Builder(SecretVolumeResponse defaults) {
Objects.requireNonNull(defaults);
this.mountPath = defaults.mountPath;
this.project = defaults.project;
this.secret = defaults.secret;
this.versions = defaults.versions;
}
@CustomType.Setter
public Builder mountPath(String mountPath) {
this.mountPath = Objects.requireNonNull(mountPath);
return this;
}
@CustomType.Setter
public Builder project(String project) {
this.project = Objects.requireNonNull(project);
return this;
}
@CustomType.Setter
public Builder secret(String secret) {
this.secret = Objects.requireNonNull(secret);
return this;
}
@CustomType.Setter
public Builder versions(List versions) {
this.versions = Objects.requireNonNull(versions);
return this;
}
public Builder versions(SecretVersionResponse... versions) {
return versions(List.of(versions));
}
public SecretVolumeResponse build() {
final var o = new SecretVolumeResponse();
o.mountPath = mountPath;
o.project = project;
o.secret = secret;
o.versions = versions;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy