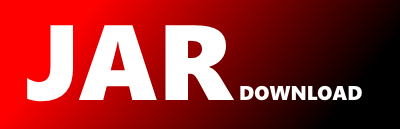
com.pulumi.googlenative.cloudfunctions.v2beta.inputs.SecretVolumeArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.cloudfunctions.v2beta.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.cloudfunctions.v2beta.inputs.SecretVersionArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configuration for a secret volume. It has the information necessary to fetch the secret value from secret manager and make it available as files mounted at the requested paths within the application container.
*
*/
public final class SecretVolumeArgs extends com.pulumi.resources.ResourceArgs {
public static final SecretVolumeArgs Empty = new SecretVolumeArgs();
/**
* The path within the container to mount the secret volume. For example, setting the mount_path as `/etc/secrets` would mount the secret value files under the `/etc/secrets` directory. This directory will also be completely shadowed and unavailable to mount any other secrets. Recommended mount path: /etc/secrets
*
*/
@Import(name="mountPath")
private @Nullable Output mountPath;
/**
* @return The path within the container to mount the secret volume. For example, setting the mount_path as `/etc/secrets` would mount the secret value files under the `/etc/secrets` directory. This directory will also be completely shadowed and unavailable to mount any other secrets. Recommended mount path: /etc/secrets
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy