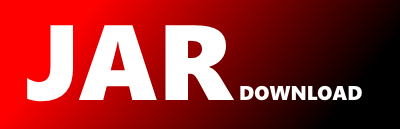
com.pulumi.googlenative.cloudidentity.v1.outputs.GetGroupResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.cloudidentity.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.cloudidentity.v1.outputs.DynamicGroupMetadataResponse;
import com.pulumi.googlenative.cloudidentity.v1.outputs.EntityKeyResponse;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetGroupResult {
/**
* @return Additional group keys associated with the Group.
*
*/
private List additionalGroupKeys;
/**
* @return The time when the `Group` was created.
*
*/
private String createTime;
/**
* @return An extended description to help users determine the purpose of a `Group`. Must not be longer than 4,096 characters.
*
*/
private String description;
/**
* @return The display name of the `Group`.
*
*/
private String displayName;
/**
* @return Optional. Dynamic group metadata like queries and status.
*
*/
private DynamicGroupMetadataResponse dynamicGroupMetadata;
/**
* @return The `EntityKey` of the `Group`.
*
*/
private EntityKeyResponse groupKey;
/**
* @return One or more label entries that apply to the Group. Currently supported labels contain a key with an empty value. Google Groups are the default type of group and have a label with a key of `cloudidentity.googleapis.com/groups.discussion_forum` and an empty value. Existing Google Groups can have an additional label with a key of `cloudidentity.googleapis.com/groups.security` and an empty value added to them. **This is an immutable change and the security label cannot be removed once added.** Dynamic groups have a label with a key of `cloudidentity.googleapis.com/groups.dynamic`. Identity-mapped groups for Cloud Search have a label with a key of `system/groups/external` and an empty value.
*
*/
private Map labels;
/**
* @return The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group`. Shall be of the form `groups/{group}`.
*
*/
private String name;
/**
* @return Immutable. The resource name of the entity under which this `Group` resides in the Cloud Identity resource hierarchy. Must be of the form `identitysources/{identity_source}` for external [identity-mapped groups](https://support.google.com/a/answer/9039510) or `customers/{customer_id}` for Google Groups. The `customer_id` must begin with "C" (for example, 'C046psxkn'). [Find your customer ID.] (https://support.google.com/cloudidentity/answer/10070793)
*
*/
private String parent;
/**
* @return The time when the `Group` was last updated.
*
*/
private String updateTime;
private GetGroupResult() {}
/**
* @return Additional group keys associated with the Group.
*
*/
public List additionalGroupKeys() {
return this.additionalGroupKeys;
}
/**
* @return The time when the `Group` was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return An extended description to help users determine the purpose of a `Group`. Must not be longer than 4,096 characters.
*
*/
public String description() {
return this.description;
}
/**
* @return The display name of the `Group`.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Optional. Dynamic group metadata like queries and status.
*
*/
public DynamicGroupMetadataResponse dynamicGroupMetadata() {
return this.dynamicGroupMetadata;
}
/**
* @return The `EntityKey` of the `Group`.
*
*/
public EntityKeyResponse groupKey() {
return this.groupKey;
}
/**
* @return One or more label entries that apply to the Group. Currently supported labels contain a key with an empty value. Google Groups are the default type of group and have a label with a key of `cloudidentity.googleapis.com/groups.discussion_forum` and an empty value. Existing Google Groups can have an additional label with a key of `cloudidentity.googleapis.com/groups.security` and an empty value added to them. **This is an immutable change and the security label cannot be removed once added.** Dynamic groups have a label with a key of `cloudidentity.googleapis.com/groups.dynamic`. Identity-mapped groups for Cloud Search have a label with a key of `system/groups/external` and an empty value.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return The [resource name](https://cloud.google.com/apis/design/resource_names) of the `Group`. Shall be of the form `groups/{group}`.
*
*/
public String name() {
return this.name;
}
/**
* @return Immutable. The resource name of the entity under which this `Group` resides in the Cloud Identity resource hierarchy. Must be of the form `identitysources/{identity_source}` for external [identity-mapped groups](https://support.google.com/a/answer/9039510) or `customers/{customer_id}` for Google Groups. The `customer_id` must begin with "C" (for example, 'C046psxkn'). [Find your customer ID.] (https://support.google.com/cloudidentity/answer/10070793)
*
*/
public String parent() {
return this.parent;
}
/**
* @return The time when the `Group` was last updated.
*
*/
public String updateTime() {
return this.updateTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List additionalGroupKeys;
private String createTime;
private String description;
private String displayName;
private DynamicGroupMetadataResponse dynamicGroupMetadata;
private EntityKeyResponse groupKey;
private Map labels;
private String name;
private String parent;
private String updateTime;
public Builder() {}
public Builder(GetGroupResult defaults) {
Objects.requireNonNull(defaults);
this.additionalGroupKeys = defaults.additionalGroupKeys;
this.createTime = defaults.createTime;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.dynamicGroupMetadata = defaults.dynamicGroupMetadata;
this.groupKey = defaults.groupKey;
this.labels = defaults.labels;
this.name = defaults.name;
this.parent = defaults.parent;
this.updateTime = defaults.updateTime;
}
@CustomType.Setter
public Builder additionalGroupKeys(List additionalGroupKeys) {
this.additionalGroupKeys = Objects.requireNonNull(additionalGroupKeys);
return this;
}
public Builder additionalGroupKeys(EntityKeyResponse... additionalGroupKeys) {
return additionalGroupKeys(List.of(additionalGroupKeys));
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
this.displayName = Objects.requireNonNull(displayName);
return this;
}
@CustomType.Setter
public Builder dynamicGroupMetadata(DynamicGroupMetadataResponse dynamicGroupMetadata) {
this.dynamicGroupMetadata = Objects.requireNonNull(dynamicGroupMetadata);
return this;
}
@CustomType.Setter
public Builder groupKey(EntityKeyResponse groupKey) {
this.groupKey = Objects.requireNonNull(groupKey);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder parent(String parent) {
this.parent = Objects.requireNonNull(parent);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
public GetGroupResult build() {
final var o = new GetGroupResult();
o.additionalGroupKeys = additionalGroupKeys;
o.createTime = createTime;
o.description = description;
o.displayName = displayName;
o.dynamicGroupMetadata = dynamicGroupMetadata;
o.groupKey = groupKey;
o.labels = labels;
o.name = name;
o.parent = parent;
o.updateTime = updateTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy