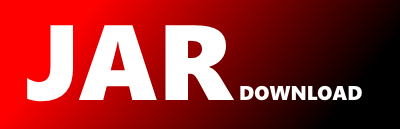
com.pulumi.googlenative.cloudtasks.v2beta2.outputs.HttpRequestResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.cloudtasks.v2beta2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.cloudtasks.v2beta2.outputs.OAuthTokenResponse;
import com.pulumi.googlenative.cloudtasks.v2beta2.outputs.OidcTokenResponse;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class HttpRequestResponse {
/**
* @return HTTP request body. A request body is allowed only if the HTTP method is POST, PUT, or PATCH. It is an error to set body on a task with an incompatible HttpMethod.
*
*/
private String body;
/**
* @return HTTP request headers. This map contains the header field names and values. Headers can be set when running the task is created or task is created. These headers represent a subset of the headers that will accompany the task's HTTP request. Some HTTP request headers will be ignored or replaced. A partial list of headers that will be ignored or replaced is: * Any header that is prefixed with "X-CloudTasks-" will be treated as service header. Service headers define properties of the task and are predefined in CloudTask. * Host: This will be computed by Cloud Tasks and derived from HttpRequest.url. * Content-Length: This will be computed by Cloud Tasks. * User-Agent: This will be set to `"Google-Cloud-Tasks"`. * `X-Google-*`: Google use only. * `X-AppEngine-*`: Google use only. `Content-Type` won't be set by Cloud Tasks. You can explicitly set `Content-Type` to a media type when the task is created. For example, `Content-Type` can be set to `"application/octet-stream"` or `"application/json"`. Headers which can have multiple values (according to RFC2616) can be specified using comma-separated values. The size of the headers must be less than 80KB.
*
*/
private Map headers;
/**
* @return The HTTP method to use for the request. The default is POST.
*
*/
private String httpMethod;
/**
* @return If specified, an [OAuth token](https://developers.google.com/identity/protocols/OAuth2) will be generated and attached as an `Authorization` header in the HTTP request. This type of authorization should generally only be used when calling Google APIs hosted on *.googleapis.com.
*
*/
private OAuthTokenResponse oauthToken;
/**
* @return If specified, an [OIDC](https://developers.google.com/identity/protocols/OpenIDConnect) token will be generated and attached as an `Authorization` header in the HTTP request. This type of authorization can be used for many scenarios, including calling Cloud Run, or endpoints where you intend to validate the token yourself.
*
*/
private OidcTokenResponse oidcToken;
/**
* @return The full url path that the request will be sent to. This string must begin with either "http://" or "https://". Some examples are: `http://acme.com` and `https://acme.com/sales:8080`. Cloud Tasks will encode some characters for safety and compatibility. The maximum allowed URL length is 2083 characters after encoding. The `Location` header response from a redirect response [`300` - `399`] may be followed. The redirect is not counted as a separate attempt.
*
*/
private String url;
private HttpRequestResponse() {}
/**
* @return HTTP request body. A request body is allowed only if the HTTP method is POST, PUT, or PATCH. It is an error to set body on a task with an incompatible HttpMethod.
*
*/
public String body() {
return this.body;
}
/**
* @return HTTP request headers. This map contains the header field names and values. Headers can be set when running the task is created or task is created. These headers represent a subset of the headers that will accompany the task's HTTP request. Some HTTP request headers will be ignored or replaced. A partial list of headers that will be ignored or replaced is: * Any header that is prefixed with "X-CloudTasks-" will be treated as service header. Service headers define properties of the task and are predefined in CloudTask. * Host: This will be computed by Cloud Tasks and derived from HttpRequest.url. * Content-Length: This will be computed by Cloud Tasks. * User-Agent: This will be set to `"Google-Cloud-Tasks"`. * `X-Google-*`: Google use only. * `X-AppEngine-*`: Google use only. `Content-Type` won't be set by Cloud Tasks. You can explicitly set `Content-Type` to a media type when the task is created. For example, `Content-Type` can be set to `"application/octet-stream"` or `"application/json"`. Headers which can have multiple values (according to RFC2616) can be specified using comma-separated values. The size of the headers must be less than 80KB.
*
*/
public Map headers() {
return this.headers;
}
/**
* @return The HTTP method to use for the request. The default is POST.
*
*/
public String httpMethod() {
return this.httpMethod;
}
/**
* @return If specified, an [OAuth token](https://developers.google.com/identity/protocols/OAuth2) will be generated and attached as an `Authorization` header in the HTTP request. This type of authorization should generally only be used when calling Google APIs hosted on *.googleapis.com.
*
*/
public OAuthTokenResponse oauthToken() {
return this.oauthToken;
}
/**
* @return If specified, an [OIDC](https://developers.google.com/identity/protocols/OpenIDConnect) token will be generated and attached as an `Authorization` header in the HTTP request. This type of authorization can be used for many scenarios, including calling Cloud Run, or endpoints where you intend to validate the token yourself.
*
*/
public OidcTokenResponse oidcToken() {
return this.oidcToken;
}
/**
* @return The full url path that the request will be sent to. This string must begin with either "http://" or "https://". Some examples are: `http://acme.com` and `https://acme.com/sales:8080`. Cloud Tasks will encode some characters for safety and compatibility. The maximum allowed URL length is 2083 characters after encoding. The `Location` header response from a redirect response [`300` - `399`] may be followed. The redirect is not counted as a separate attempt.
*
*/
public String url() {
return this.url;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HttpRequestResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String body;
private Map headers;
private String httpMethod;
private OAuthTokenResponse oauthToken;
private OidcTokenResponse oidcToken;
private String url;
public Builder() {}
public Builder(HttpRequestResponse defaults) {
Objects.requireNonNull(defaults);
this.body = defaults.body;
this.headers = defaults.headers;
this.httpMethod = defaults.httpMethod;
this.oauthToken = defaults.oauthToken;
this.oidcToken = defaults.oidcToken;
this.url = defaults.url;
}
@CustomType.Setter
public Builder body(String body) {
this.body = Objects.requireNonNull(body);
return this;
}
@CustomType.Setter
public Builder headers(Map headers) {
this.headers = Objects.requireNonNull(headers);
return this;
}
@CustomType.Setter
public Builder httpMethod(String httpMethod) {
this.httpMethod = Objects.requireNonNull(httpMethod);
return this;
}
@CustomType.Setter
public Builder oauthToken(OAuthTokenResponse oauthToken) {
this.oauthToken = Objects.requireNonNull(oauthToken);
return this;
}
@CustomType.Setter
public Builder oidcToken(OidcTokenResponse oidcToken) {
this.oidcToken = Objects.requireNonNull(oidcToken);
return this;
}
@CustomType.Setter
public Builder url(String url) {
this.url = Objects.requireNonNull(url);
return this;
}
public HttpRequestResponse build() {
final var o = new HttpRequestResponse();
o.body = body;
o.headers = headers;
o.httpMethod = httpMethod;
o.oauthToken = oauthToken;
o.oidcToken = oidcToken;
o.url = url;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy