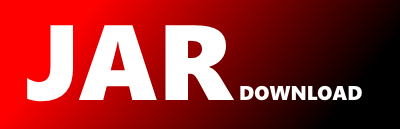
com.pulumi.googlenative.compute.alpha.NodeGroup Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.compute.alpha;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.compute.alpha.NodeGroupArgs;
import com.pulumi.googlenative.compute.alpha.outputs.NodeGroupAutoscalingPolicyResponse;
import com.pulumi.googlenative.compute.alpha.outputs.NodeGroupMaintenanceWindowResponse;
import com.pulumi.googlenative.compute.alpha.outputs.ShareSettingsResponse;
import java.lang.Integer;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates a NodeGroup resource in the specified project using the data included in the request.
*
*/
@ResourceType(type="google-native:compute/alpha:NodeGroup")
public class NodeGroup extends com.pulumi.resources.CustomResource {
/**
* Specifies how autoscaling should behave.
*
*/
@Export(name="autoscalingPolicy", type=NodeGroupAutoscalingPolicyResponse.class, parameters={})
private Output autoscalingPolicy;
/**
* @return Specifies how autoscaling should behave.
*
*/
public Output autoscalingPolicy() {
return this.autoscalingPolicy;
}
/**
* Creation timestamp in RFC3339 text format.
*
*/
@Export(name="creationTimestamp", type=String.class, parameters={})
private Output creationTimestamp;
/**
* @return Creation timestamp in RFC3339 text format.
*
*/
public Output creationTimestamp() {
return this.creationTimestamp;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
*
*/
@Export(name="description", type=String.class, parameters={})
private Output description;
/**
* @return An optional description of this resource. Provide this property when you create the resource.
*
*/
public Output description() {
return this.description;
}
@Export(name="fingerprint", type=String.class, parameters={})
private Output fingerprint;
public Output fingerprint() {
return this.fingerprint;
}
/**
* Initial count of nodes in the node group.
*
*/
@Export(name="initialNodeCount", type=Integer.class, parameters={})
private Output initialNodeCount;
/**
* @return Initial count of nodes in the node group.
*
*/
public Output initialNodeCount() {
return this.initialNodeCount;
}
/**
* The type of the resource. Always compute#nodeGroup for node group.
*
*/
@Export(name="kind", type=String.class, parameters={})
private Output kind;
/**
* @return The type of the resource. Always compute#nodeGroup for node group.
*
*/
public Output kind() {
return this.kind;
}
/**
* An opaque location hint used to place the Node close to other resources. This field is for use by internal tools that use the public API. The location hint here on the NodeGroup overrides any location_hint present in the NodeTemplate.
*
*/
@Export(name="locationHint", type=String.class, parameters={})
private Output locationHint;
/**
* @return An opaque location hint used to place the Node close to other resources. This field is for use by internal tools that use the public API. The location hint here on the NodeGroup overrides any location_hint present in the NodeTemplate.
*
*/
public Output locationHint() {
return this.locationHint;
}
/**
* Specifies the frequency of planned maintenance events. The accepted values are: `AS_NEEDED` and `RECURRENT`.
*
*/
@Export(name="maintenanceInterval", type=String.class, parameters={})
private Output maintenanceInterval;
/**
* @return Specifies the frequency of planned maintenance events. The accepted values are: `AS_NEEDED` and `RECURRENT`.
*
*/
public Output maintenanceInterval() {
return this.maintenanceInterval;
}
/**
* Specifies how to handle instances when a node in the group undergoes maintenance. Set to one of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT. For more information, see Maintenance policies.
*
*/
@Export(name="maintenancePolicy", type=String.class, parameters={})
private Output maintenancePolicy;
/**
* @return Specifies how to handle instances when a node in the group undergoes maintenance. Set to one of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT. For more information, see Maintenance policies.
*
*/
public Output maintenancePolicy() {
return this.maintenancePolicy;
}
@Export(name="maintenanceWindow", type=NodeGroupMaintenanceWindowResponse.class, parameters={})
private Output maintenanceWindow;
public Output maintenanceWindow() {
return this.maintenanceWindow;
}
/**
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*/
@Export(name="name", type=String.class, parameters={})
private Output name;
/**
* @return The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*/
public Output name() {
return this.name;
}
/**
* URL of the node template to create the node group from.
*
*/
@Export(name="nodeTemplate", type=String.class, parameters={})
private Output nodeTemplate;
/**
* @return URL of the node template to create the node group from.
*
*/
public Output nodeTemplate() {
return this.nodeTemplate;
}
@Export(name="project", type=String.class, parameters={})
private Output project;
public Output project() {
return this.project;
}
/**
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*/
@Export(name="requestId", type=String.class, parameters={})
private Output* @Nullable */ String> requestId;
/**
* @return An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*/
public Output> requestId() {
return Codegen.optional(this.requestId);
}
/**
* Server-defined URL for the resource.
*
*/
@Export(name="selfLink", type=String.class, parameters={})
private Output selfLink;
/**
* @return Server-defined URL for the resource.
*
*/
public Output selfLink() {
return this.selfLink;
}
/**
* Server-defined URL for this resource with the resource id.
*
*/
@Export(name="selfLinkWithId", type=String.class, parameters={})
private Output selfLinkWithId;
/**
* @return Server-defined URL for this resource with the resource id.
*
*/
public Output selfLinkWithId() {
return this.selfLinkWithId;
}
/**
* Share-settings for the node group
*
*/
@Export(name="shareSettings", type=ShareSettingsResponse.class, parameters={})
private Output shareSettings;
/**
* @return Share-settings for the node group
*
*/
public Output shareSettings() {
return this.shareSettings;
}
/**
* The total number of nodes in the node group.
*
*/
@Export(name="size", type=Integer.class, parameters={})
private Output size;
/**
* @return The total number of nodes in the node group.
*
*/
public Output size() {
return this.size;
}
@Export(name="status", type=String.class, parameters={})
private Output status;
public Output status() {
return this.status;
}
@Export(name="zone", type=String.class, parameters={})
private Output zone;
public Output zone() {
return this.zone;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public NodeGroup(String name) {
this(name, NodeGroupArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public NodeGroup(String name, NodeGroupArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public NodeGroup(String name, NodeGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:compute/alpha:NodeGroup", name, args == null ? NodeGroupArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private NodeGroup(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:compute/alpha:NodeGroup", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static NodeGroup get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new NodeGroup(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy